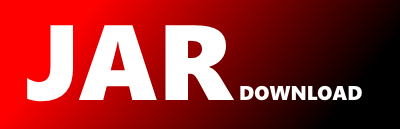
org.openrdf.util.uri.UriUtil Maven / Gradle / Ivy
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.util.uri;
/**
* Methods related to actions on URIs. The definition of a URI is taken from
* RFC 2396: URI Generic Syntax.
**/
public class UriUtil {
private static final String ESCAPE_CHARS = "<>#%\"{}|\\^[]`";
/**
* Encodes a string according to RFC 2396. According to this spec, any
* characters outside the range 0x20 - 0x7E must be escaped because they
* are not printable characters, except for characters in the fragment
* identifier. Even within this range a number of characters must be
* escaped. This method will perform this escaping.
*
* @param uri The URI to encode.
* @return The encoded URI.
**/
public static String encodeUri(String uri) {
StringBuffer result = new StringBuffer(2 * uri.length());
encodeUri(uri, result);
return result.toString();
}
/**
* Encodes a string according to RFC 2396.
*
* @param uri The URI to encode.
* @param buf The StringBuffer that the encoded URI will be appended to.
* @see #encodeUri(java.lang.String)
**/
public static void encodeUri(String uri, StringBuffer buf) {
for (int i = 0; i < uri.length(); i++) {
char c = uri.charAt(i);
int cInt = (int)c;
if (ESCAPE_CHARS.indexOf(c) >= 0 || cInt <= 0x20) {
// Escape character
buf.append('%');
String hexVal = Integer.toHexString(cInt);
// Ensure use of two characters
if (hexVal.length() == 1) {
buf.append('0');
}
buf.append(hexVal);
}
else {
buf.append(c);
}
}
}
/**
* Decodes a string according to RFC 2396. According to this spec, any
* characters outside the range 0x20 - 0x7E must be escaped because they
* are not printable characters, except for any characters in the fragment
* identifier. This method will translate any escaped characters back to
* the original.
*
* @param uri The URI to decode.
* @return The decoded URI.
**/
public static String decodeUri(String uri) {
StringBuffer result = new StringBuffer(uri.length());
decodeUri(uri, result);
return result.toString();
}
/**
* Decodes a string according to RFC 2396.
*
* @param uri The URI to decode.
* @param buf The StringBuffer that the decoded URI will be appended to.
* @see #decodeUri(java.lang.String)
**/
public static void decodeUri(String uri, StringBuffer buf) {
// Search for a fragment identifier
int indexOfHash = uri.indexOf('#');
if (indexOfHash == -1) {
// No fragment identifier
_decodeUri(uri, buf);
}
else {
// Fragment identifier found
String baseUri = uri.substring(0, indexOfHash);
String fragId = uri.substring(indexOfHash);
_decodeUri(baseUri, buf);
buf.append(fragId);
}
}
private static void _decodeUri(String uri, StringBuffer buf) {
int percentIdx = uri.indexOf('%');
int startIdx = 0;
while (percentIdx != -1) {
buf.append(uri.substring(startIdx, percentIdx));
// The two character following the '%' contain a hexadecimal
// code for the original character, i.e. '%20'
String xx = uri.substring(percentIdx + 1, percentIdx + 3);
int c = Integer.parseInt(xx, 16);
buf.append( (char)c );
startIdx = percentIdx + 3;
percentIdx = uri.indexOf('%', startIdx);
}
buf.append( uri.substring(startIdx) );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy