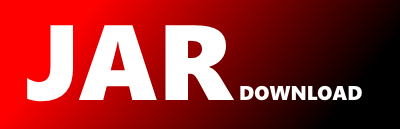
org.openrdf.sesame.config.ui.ConfigureSesameFrame Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.config.ui;
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Window;
import java.awt.event.ActionEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import javax.swing.AbstractAction;
import javax.swing.Action;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JTabbedPane;
import javax.swing.JToolBar;
import org.openrdf.sesame.config.SystemConfig;
import org.openrdf.sesame.config.SystemConfigListener;
import org.openrdf.sesame.config.handlers.SystemConfigFileHandler;
import org.openrdf.sesame.config.ui.util.Util;
/**
* Main JFrame of Configure Sesame!
*
* @author Peter van 't Hof
* @author Arjohn Kampman
* @version $Revision: 1.5.4.2 $
*/
public class ConfigureSesameFrame extends JFrame implements SystemConfigListener {
/*----------+
| Variables |
+----------*/
/** File chooser */
protected SystemConfigFileChooser _fileChooser;
/** Panel for loading/sending a system configuration from/to a server. **/
protected ServerIOPanel _serverIOPanel;
/** SystemConfig */
protected SystemConfig _config;
/** Indicates if the SystemConfig has changed */
protected boolean _configChanged;
/** SystemConfig File */
protected File _file;
/** Server URL **/
protected String _serverURL;
/*
* Actions
*/
protected Action _newAction;
protected Action _closeAction;
protected Action _loadFromServerAction;
protected Action _sendToServerAction;
protected Action _openFileAction;
protected Action _saveFileAction;
protected Action _saveFileAsAction;
protected Action _exitAction;
protected Action _aboutAction;
/*-------------+
| Constructors |
+-------------*/
/** Creates a new ConfigureSesameFrame */
public ConfigureSesameFrame() {
_config = new SystemConfig();
_config.addListener(this);
_configChanged = false;
_fileChooser = new SystemConfigFileChooser();
_serverIOPanel = new ServerIOPanel();
setTitle("Configure Sesame!");
// Icon on title bar
URL url = getClass().getResource("icons/cs.png");
ImageIcon imageIcon = new ImageIcon(url);
setIconImage(imageIcon.getImage());
// Create actions
_createActions();
// Create menu bar
JMenuBar menuBar = _createMenuBar();
setJMenuBar(menuBar);
// Create tool bar
JToolBar toolBar = _createToolBar();
JTabbedPane tabs = new JTabbedPane();
tabs.add("Users", new UserTab(_config));
tabs.add("Repositories", new RepositoryTab(_config));
tabs.add("Server", new ServerTab(_config));
Container contentPane = getContentPane();
contentPane.add(toolBar, BorderLayout.NORTH);
contentPane.add(tabs, BorderLayout.CENTER);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
if (_discardCurrentConf()) {
System.exit(0);
}
}
} );
}
protected void _createActions() {
_newAction = _createNewAction();
_closeAction = _createCloseAction();
_loadFromServerAction = _createLoadFromServerAction();
_sendToServerAction = _createSendToServerAction();
_openFileAction = _createOpenFileAction();
_saveFileAction = _createSaveFileAction();
_saveFileAsAction = _createSaveFileAsAction();
_exitAction = _createExitAction();
_aboutAction = _createAboutAction();
}
protected JMenuBar _createMenuBar() {
// File menu
JMenu fileMenu = new JMenu("File");
fileMenu.setMnemonic('F');
fileMenu.add(new JMenuItem(_newAction));
fileMenu.add(new JMenuItem(_closeAction));
fileMenu.addSeparator();
fileMenu.add(new JMenuItem(_loadFromServerAction));
fileMenu.add(new JMenuItem(_sendToServerAction));
fileMenu.addSeparator();
fileMenu.add(new JMenuItem(_openFileAction));
fileMenu.add(new JMenuItem(_saveFileAction));
fileMenu.add(new JMenuItem(_saveFileAsAction));
fileMenu.addSeparator();
fileMenu.add(new JMenuItem(_exitAction));
// Help menu
JMenu helpMenu = new JMenu("Help");
helpMenu.setMnemonic('H');
helpMenu.add(new JMenuItem(_aboutAction));
// Menu bar
JMenuBar menuBar = new JMenuBar();
menuBar.add(fileMenu);
menuBar.add(helpMenu);
return menuBar;
}
protected JToolBar _createToolBar() {
JToolBar toolBar = new JToolBar();
toolBar.add(_newAction);
toolBar.addSeparator();
toolBar.add(_loadFromServerAction);
toolBar.add(_sendToServerAction);
toolBar.addSeparator();
toolBar.add(_openFileAction);
toolBar.add(_saveFileAction);
return toolBar;
}
protected Action _createNewAction() {
URL url = this.getClass().getResource("icons/file_new.gif");
Icon icon = new ImageIcon(url);
Action action = new AbstractAction("New", icon)
{
public void actionPerformed(ActionEvent e) {
if (_discardCurrentConf()) {
_file = null;
_serverURL = null;
_configChanged = false;
_config.setSystemConfig(new SystemConfig());
_fileChooser.setSelectedFile(null);
}
}
};
action.putValue(Action.SHORT_DESCRIPTION, "New configuration");
action.putValue(Action.MNEMONIC_KEY, new Integer('N'));
return action;
}
protected Action _createCloseAction() {
Action action = new AbstractAction("Close")
{
public void actionPerformed(ActionEvent e) {
if (_discardCurrentConf()) {
_file = null;
_serverURL = null;
_configChanged = false;
_config.setSystemConfig(new SystemConfig());
_fileChooser.setSelectedFile(null);
}
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Close configuration");
action.putValue(Action.MNEMONIC_KEY, new Integer('C'));
return action;
}
protected Action _createLoadFromServerAction() {
URL url = this.getClass().getResource("icons/loadfromserver.png");
Icon icon = new ImageIcon(url);
Action action = new AbstractAction("Load from server...", icon)
{
public void actionPerformed(ActionEvent e) {
if (_discardCurrentConf()) {
SystemConfig newConfig = _serverIOPanel.showLoadDialog(ConfigureSesameFrame.this);
if (newConfig != null) {
_file = null;
_serverURL = _serverIOPanel.getServerURL();
_config.setSystemConfig(newConfig);
_configChanged = false;
_updateTitle();
}
}
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Load configuration from server");
action.putValue(Action.MNEMONIC_KEY, new Integer('L'));
return action;
}
protected Action _createSendToServerAction() {
URL url = this.getClass().getResource("icons/sendtoserver.png");
Icon icon = new ImageIcon(url);
Action action = new AbstractAction("Send to server...", icon)
{
public void actionPerformed(ActionEvent e) {
_serverIOPanel.showSendDialog(ConfigureSesameFrame.this, _config);
_configChanged = false;
_updateTitle();
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Send configuration to server");
action.putValue(Action.MNEMONIC_KEY, new Integer('S'));
return action;
}
protected Action _createOpenFileAction() {
URL url = this.getClass().getResource("icons/file_open.gif");
Icon icon = new ImageIcon(url);
Action action = new AbstractAction("Open file...", icon)
{
public void actionPerformed(ActionEvent e) {
if (_discardCurrentConf()) {
if (_fileChooser.showOpenDialog(ConfigureSesameFrame.this) == JFileChooser.APPROVE_OPTION) {
load( _fileChooser.getSelectedFile() );
}
}
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Open configuration file");
action.putValue(Action.MNEMONIC_KEY, new Integer('O'));
return action;
}
protected Action _createSaveFileAction() {
URL url = this.getClass().getResource("icons/file_save.gif");
Icon icon = new ImageIcon(url);
Action action = new AbstractAction("Save file", icon)
{
public void actionPerformed(ActionEvent e) {
if (_file == null) {
// New SystemConfig
_saveAs();
}
else {
_save();
}
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Save configuration file");
action.putValue(Action.MNEMONIC_KEY, new Integer('f'));
return action;
}
protected Action _createSaveFileAsAction() {
Action action = new AbstractAction("Save file as...")
{
public void actionPerformed(ActionEvent e) {
_saveAs();
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Save configuration file as");
action.putValue(Action.MNEMONIC_KEY, new Integer('a'));
return action;
}
protected Action _createExitAction() {
Action action = new AbstractAction("Exit")
{
public void actionPerformed(ActionEvent e) {
if (_discardCurrentConf()) {
System.exit(0);
}
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Exit Configure Sesame!");
action.putValue(Action.MNEMONIC_KEY, new Integer('x'));
return action;
}
protected Action _createAboutAction() {
Action action = new AbstractAction("About")
{
public void actionPerformed(ActionEvent e) {
AboutDialog dialog = new AboutDialog(ConfigureSesameFrame.this);
dialog.show();
}
};
action.putValue(Action.SHORT_DESCRIPTION, "Show info about Configure Sesame!");
action.putValue(Action.MNEMONIC_KEY, new Integer('A'));
return action;
}
/*
protected JMenuItem _createMenuItem(String text, String name, int keyCode, int mnemonic) {
// Get URL from CLASSPATH
URL url = this.getClass().getResource("icons/" + name);
JMenuItem item = new JMenuItem(text, new ImageIcon(url));
KeyStroke accelerator = KeyStroke.getKeyStroke(keyCode, InputEvent.CTRL_MASK);
item.setAccelerator(accelerator);
item.setMnemonic(mnemonic);
return item;
}
*/
/*--------+
| Methods |
+--------*/
public void configurationChanged() {
_configChanged = true;
_updateTitle();
}
protected void _updateTitle() {
String title = "";
if (_file != null ) {
title += _file.getName();
if (_configChanged) {
title += "*";
}
title += " - ";
}
else if (_serverURL != null) {
title += _serverURL;
if (_configChanged) {
title += "*";
}
title += " - ";
}
title += "Configure Sesame!";
setTitle(title);
}
/**
* Checks whether the current configuration contains any changes that
* have not yet been saved and, if this is the case, whether this
* configuration should be saved.
*
* @return true if the current configuration can be discarded (it has
* been saved or the user doesn't want to save it), false if the user
* wants to continue editing the current configuration.
**/
protected boolean _discardCurrentConf() {
if (_configChanged) {
Window owner = Util.getOwner(this);
String message = "Do you want to save your changes";
if (_file != null) {
message += " to '" + _file.getName() + "'";
}
message += "?";
int option = JOptionPane.showConfirmDialog
(owner, message, "Configure Sesame!", JOptionPane.YES_NO_CANCEL_OPTION);
if (option == JOptionPane.YES_OPTION) {
if (_file == null) {
_saveAs();
}
else {
_save();
}
}
else if (option == JOptionPane.CANCEL_OPTION) {
return false;
}
}
return true;
}
/**
* Loads a system.conf file.
**/
public void load(File file) {
_file = file;
_serverURL = null;
_load();
}
/**
* Loads the system.conf file whose filename is stored in _file.
**/
protected void _load() {
try {
InputStream in = new FileInputStream(_file);
SystemConfig newConfig = SystemConfigFileHandler.readConfiguration(in);
in.close();
_config.setSystemConfig(newConfig);
_configChanged = false;
_updateTitle();
}
catch (Exception e) {
// DOME What to do when Exception is thrown?
System.out.println(e);
}
}
protected void _saveAs() {
if (_file == null) {
// Propose default file name
_fileChooser.setSelectedFile(new File("system.conf"));
}
if (_fileChooser.showSaveDialog(this) == JFileChooser.APPROVE_OPTION) {
_file = _fileChooser.getSelectedFile();
_save();
}
_fileChooser.setSelectedFile(_file);
}
protected void _save() {
try {
OutputStream outputStream = new FileOutputStream(_file);
SystemConfigFileHandler.writeConfiguration(_config, outputStream);
outputStream.flush();
outputStream.close();
_configChanged = false;
_updateTitle();
}
catch (Exception e) {
// DOME What to do when Exception is thrown?
System.out.println(e);
}
}
/*--------------+
| Inner classes |
+--------------*/
class SystemConfigFileChooser extends JFileChooser {
public SystemConfigFileChooser() {
super();
this.setFileFilter(
new javax.swing.filechooser.FileFilter() {
public boolean accept(File file) {
return file.getName().endsWith(".conf") || file.isDirectory();
}
public String getDescription() {
return ".conf";
}
});
}
public void approveSelection() {
if (getDialogType() == JFileChooser.SAVE_DIALOG) {
File file = this.getSelectedFile();
if ((_file == null || !_file.getPath().equals(file.getPath())) &&
file.exists()) {
// Selected file is different from the SystemConfig
// file and already exists
Window owner = Util.getOwner(this);
int option = JOptionPane.showConfirmDialog(owner,
"The file '" + file.getName() + "' already exists.\n" +
"Are you sure you want to overwrite the existing file?",
"Configure Sesame!", JOptionPane.YES_NO_CANCEL_OPTION);
if (option == JOptionPane.NO_OPTION) {
// Return to file chooser
setSelectedFile(_file);
return;
}
else if (option == JOptionPane.CANCEL_OPTION) {
cancelSelection();
return;
}
}
}
else if (getDialogType() == JFileChooser.OPEN_DIALOG) {
File file = this.getSelectedFile();
if (!file.exists()) {
Window owner = Util.getOwner(this);
Util.showWarningDialog(owner,
"The file '" + file.getName() + "' does not exist.",
"Configure Sesame!");
return;
}
}
super.approveSelection();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy