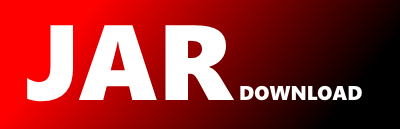
org.openrdf.sesame.query.GraphQuery Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.query;
import java.io.IOException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import org.openrdf.model.Resource;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import org.openrdf.sesame.sail.NamespaceIterator;
import org.openrdf.sesame.sail.RdfSource;
import org.openrdf.sesame.sail.query.QueryAnswer;
import org.openrdf.sesame.sail.query.QueryAnswerListener;
import org.openrdf.sesame.sail.query.Query;
import org.openrdf.sesame.sail.query.SailQueryException;
/**
* A GraphQuery is a query that produces an RDF graph (or a set of statements)
* as its result.
**/
public class GraphQuery {
/*----------+
| Variables |
+----------*/
private Query _query;
private Map _queryNamespaces;
/*-------------+
| Constructors |
+-------------*/
/**
* Creates a new Construct-From-Where query.
*
* @param query An object representing the actual query.
* @param namespaces A Map containing prefixes (key) and names
* (values) of namespaces used in the query.
**/
public GraphQuery(Query query, Map namespaces) {
_query = query;
_queryNamespaces = namespaces;
}
/*--------+
| Methods |
+--------*/
public Query getQuery() {
return _query;
}
public void optimize(RdfSource source) {
_query = source.optimizeQuery(_query);
}
/**
* Evaluates this Construct-From-Where query against the supplied RdfSource
* and reports the query results to the supplied GraphQueryResultListener.
**/
public void evaluate(RdfSource rdfSource, GraphQueryResultListener listener)
throws QueryEvaluationException
{
try {
_reportNamespaces(rdfSource, listener);
}
catch (IOException e) {
throw new QueryEvaluationException(e);
}
try {
listener.startGraphQueryResult();
_query.evaluate(rdfSource, new QAL2GQRL(listener));
listener.endGraphQueryResult();
}
catch (SailQueryException e) {
throw new QueryEvaluationException(e);
}
catch (IOException e) {
throw new QueryEvaluationException(e);
}
}
private void _reportNamespaces(RdfSource rdfSource, GraphQueryResultListener listener)
throws IOException
{
Map nsMap = new HashMap();
// Fill nsMap with namespaces from rdfSource
NamespaceIterator nsIter = rdfSource.getNamespaces();
while (nsIter.hasNext()) {
nsIter.next();
nsMap.put(nsIter.getPrefix(), nsIter.getName());
}
nsIter.close();
// Add the namespaces specified in the query, possibly overwriting the
// ones from rdfSource
Iterator prefixes = _queryNamespaces.keySet().iterator();
while (prefixes.hasNext()) {
String prefix = (String)prefixes.next();
String name = (String)_queryNamespaces.get(prefix);
// Remove any entries mapping the same namespace name
nsMap.values().remove(name);
// This will overwrite any existing mapping with the same namespace
nsMap.put(prefix, name);
}
// Report this final set to the GraphQueryResultListener
prefixes = nsMap.keySet().iterator();
while (prefixes.hasNext()) {
String prefix = (String)prefixes.next();
String name = (String)nsMap.get(prefix);
listener.namespace(prefix, name);
}
}
public String toString() {
return "GraphQuery [" + super.toString() + "]";
}
/*---------------------+
| Inner class QAL2GQRL |
+---------------------*/
/**
* An adapter translating QueryAnswerListener (QAL) method calls to
* GraphQueryResultListener (GQRL) method calls.
**/
private static class QAL2GQRL implements QueryAnswerListener {
private GraphQueryResultListener _listener;
/**
* Creates a new SfwQuery.
*
* @param listener A GraphQueryResultListener.
**/
public QAL2GQRL(GraphQueryResultListener listener) {
_listener = listener;
}
// implements QueryAnswerListener.queryAnswer(QueryAnswer)
public boolean queryAnswer(QueryAnswer qa)
throws IOException
{
Resource subject = (Resource)qa.getValue(0);
URI predicate = (URI)qa.getValue(1);
Value object = qa.getValue(2);
_listener.triple(subject, predicate, object);
return true;
}
// implements QueryAnswerListener.clear()
public void clear() {
}
public void reportError(String msg)
throws IOException, QueryEvaluationException
{
_listener.reportError(msg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy