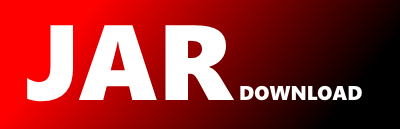
org.openrdf.sesame.query.rql.model.Like Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.query.rql.model;
import org.openrdf.model.Literal;
import org.openrdf.model.Value;
import org.openrdf.sesame.query.QueryEvaluationException;
import org.openrdf.sesame.sail.RdfSchemaSource;
import org.openrdf.sesame.sail.ValueIterator;
public class Like implements BooleanQuery {
/*-------------------------------------------+
| Variables |
+-------------------------------------------*/
protected ResourceQuery _arg1;
protected StringConstant _arg2;
/*-------------------------------------------+
| Constructors |
+-------------------------------------------*/
public Like(ResourceQuery arg1, StringConstant arg2) {
_arg1 = arg1;
_arg2 = arg2;
}
/*-------------------------------------------+
| Methods |
+-------------------------------------------*/
/**
* Determines whether the two operands match according to the
* like
operator. The operator is defined as a string
* comparison with the possible use of an asterisk (*) at the end
* and/or the start of the second operand to indicate substring
* matching.
*
* @param rss the repository abstraction layer which is to be queried
* during evaluation.
*
* @return true
if the operands match according to the
* like
operator, false
otherwise.
* @throws QueryEvaluationException when the first argument is a set
* (instead of a single value), or when it is not instantiated.
**/
public boolean isTrue(RdfSchemaSource rss) throws QueryEvaluationException {
ValueIterator iter1;
Value val;
String stringVal;
if (_arg1.returnsSet()) {
throw new QueryEvaluationException(
"Cannot compare a set to a string : " +
this.toString());
}
iter1 = _arg1.getResources(rss);
if (iter1 == null) {
throw new QueryEvaluationException(
_arg1.toString() + " has no assigned value. ");
}
// everything is peachy
val = iter1.next();
iter1.close();
if (val instanceof org.openrdf.model.URI) {
stringVal = ((org.openrdf.model.URI)val).getURI();
}
else if (val instanceof Literal) {
stringVal = ((Literal)val).getLabel();
}
else {
// Intersections or BNodes cannot be compared to a string pattern
return false;
}
return _like(stringVal, _arg2.getValue());
}
protected boolean _like(String val, String pattern) {
int valIndex = 0;
int prevPatternIndex = -1;
int patternIndex = pattern.indexOf('*');
if (patternIndex == -1) {
// No wildcards
return val.equalsIgnoreCase(pattern);
}
// Convert both strings to lower case
val = val.toLowerCase();
pattern = pattern.toLowerCase();
String snippet;
if (patternIndex > 0) {
// Pattern does not start with a wildcard, first part must match
snippet = pattern.substring(0, patternIndex);
if (!val.startsWith(snippet)) {
return false;
}
valIndex += snippet.length();
prevPatternIndex = patternIndex;
patternIndex = pattern.indexOf('*', patternIndex + 1);
}
while (patternIndex != -1) {
// Get snippet between previous wildcard and this wildcard
snippet = pattern.substring(prevPatternIndex + 1, patternIndex);
// Search for snippet
valIndex = val.indexOf(snippet, valIndex);
if (valIndex == -1) {
return false;
}
valIndex += snippet.length();
prevPatternIndex = patternIndex;
patternIndex = pattern.indexOf('*', patternIndex + 1);
}
// Part after last wildcard
snippet = pattern.substring(prevPatternIndex + 1);
if (snippet.length() > 0) {
// Pattern does not end with a wildcard.
// Search last occurence of the snippet.
valIndex = val.indexOf(snippet, valIndex);
int i;
while ((i = val.indexOf(snippet, valIndex + 1)) != -1) {
// A later occurence was found.
valIndex = i;
}
if (valIndex == -1) {
return false;
}
valIndex += snippet.length();
if (valIndex < val.length()) {
// Some characters were not matched
return false;
}
}
return true;
}
public String getQuery() {
return this.toString();
}
public String toString() {
return _arg1.toString() + " like " + _arg2.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy