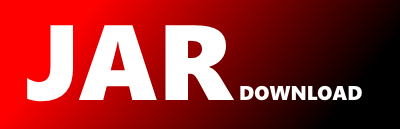
org.openrdf.sesame.query.rql.model.SuperClassOf Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.query.rql.model;
import java.io.IOException;
import org.openrdf.model.Resource;
import org.openrdf.model.Value;
import org.openrdf.sesame.query.QueryEvaluationException;
import org.openrdf.sesame.query.TableQueryResultListener;
import org.openrdf.sesame.query.rql.model.iterators.NestedResourceIterator;
import org.openrdf.sesame.query.rql.model.iterators.StatementObjectIterator;
import org.openrdf.sesame.sail.RdfSchemaSource;
import org.openrdf.sesame.sail.ResourceIterator;
import org.openrdf.sesame.sail.StatementIterator;
import org.openrdf.sesame.sail.ValueIterator;
public class SuperClassOf implements ClassQuery {
protected ClassQuery _classQ;
protected boolean _recursive;
public SuperClassOf(ClassQuery classQ, boolean recursive) {
_classQ = classQ;
_recursive = recursive;
}
public void evaluate(RdfSchemaSource rss, TableQueryResultListener listener)
throws QueryEvaluationException
{
ResourceIterator iter = null;
try {
String columnHeaders[] = new String[1];
columnHeaders[0] = this.getQuery();
listener.startTableQueryResult(columnHeaders);
iter = getClasses(rss);
while (iter.hasNext()) {
listener.startTuple();
listener.tupleValue(iter.next());
listener.endTuple();
}
listener.endTableQueryResult();
}
catch (IOException e) {
throw new QueryEvaluationException(e);
}
finally {
if (iter != null) {
iter.close();
}
}
}
public ClassQuery getArgument() {
return _classQ;
}
public ValueIterator getResources(RdfSchemaSource rss)
throws QueryEvaluationException
{
return getClasses(rss);
}
public ResourceIterator getClasses(RdfSchemaSource rss)
throws QueryEvaluationException
{
ResourceIterator classIter = _classQ.getClasses(rss);
return new Iterator(rss, classIter);
}
public boolean returnsSet() {
return true;
}
public String getQuery() {
return this.toString();
}
public String toString() {
if (_recursive) {
return "superClassOf(" + _classQ.toString() + ")";
}
else {
return "superClassOf^(" + _classQ.toString() + ")";
}
}
/*---------------------------------------------------+
| Iterator |
+---------------------------------------------------*/
class Iterator extends NestedResourceIterator {
protected RdfSchemaSource _rss;
public Iterator(RdfSchemaSource rss, ResourceIterator classIter) {
super(classIter);
_rss = rss;
}
public ResourceIterator getInnerIterator(Value classRes) {
StatementIterator statIter;
if (_recursive) {
statIter = _rss.getSubClassOf((Resource)classRes, null);
}
else {
statIter = _rss.getDirectSubClassOf((Resource)classRes, null);
}
return new StatementObjectIterator(statIter);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy