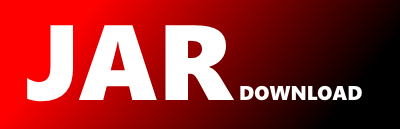
org.openrdf.sesame.repository.SesameRepository Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.repository;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.Reader;
import java.net.URL;
import org.openrdf.model.Graph;
import org.openrdf.model.Resource;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import org.openrdf.sesame.admin.AdminListener;
import org.openrdf.sesame.config.AccessDeniedException;
import org.openrdf.sesame.constants.QueryLanguage;
import org.openrdf.sesame.constants.RDFFormat;
import org.openrdf.sesame.query.GraphQueryResultListener;
import org.openrdf.sesame.query.MalformedQueryException;
import org.openrdf.sesame.query.QueryEvaluationException;
import org.openrdf.sesame.query.QueryResultsTable;
import org.openrdf.sesame.query.TableQueryResultListener;
/**
* Interface for access to a single Sesame repository. This interface
* defines a generic entry point for application developers to Sesame
* repositories. Implementations of this interface exist that supply
* client-server access, as well as local access.
*
* @author Jeen Broekstra
* @author Arjohn Kampman
*/
public interface SesameRepository {
/**
* Evaluates a query and reports the results as rows in a table to the
* supplied listener.
*
* @param language the query language in which the query is
* formulated.
* @param query the query.
* @param listener the result listener
*
* @exception IOException In case an I/O error occurs while sending the
* query to, or retrieving the result from a Sesame server.
* @exception MalformedQueryException If the supplied query is malformed.
* @exception QueryEvaluationException If a problem occurs during
* evaluation of the query.
* @exception AccessDeniedException In case the current user does not have
* read access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see QueryLanguage
*/
public void performTableQuery(QueryLanguage language, String query, TableQueryResultListener listener)
throws IOException, MalformedQueryException, QueryEvaluationException, AccessDeniedException;
/**
* Evaluates a query and returns the results in a
* QueryResultsTable.
*
* @param language The query language in which the query is formulated.
* @param query The query.
* @return A QueryResultsTable object that contains the query result. Note: if
* the query returned no results, an empty QueryResultsTable will be returned.
*
* @exception IOException In case an I/O error occurs while sending the
* query to, or retrieving the result from a Sesame server.
* @exception MalformedQueryException If the supplied query is malformed.
* @exception QueryEvaluationException If a problem occurs during
* evaluation of the query.
* @exception AccessDeniedException In case the current user does not have
* read access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see QueryLanguage
*/
public QueryResultsTable performTableQuery(QueryLanguage language, String query)
throws IOException, MalformedQueryException, QueryEvaluationException, AccessDeniedException;
/**
* Evaluates a graph query and reports the resulting statements to the
* specified listener.
*
* @param language The query language in which the query is formulated.
* Currently, only SeRQL supports graph queries and
* QueryLanguage.SERQL is the only legal value.
* @param query The query.
* @param listener A listener for the query result.
*
* @exception IOException In case an I/O error occurs while sending the
* query to, or retrieving the result from a Sesame server.
* @exception MalformedQueryException If the supplied query is malformed.
* @exception QueryEvaluationException If a problem occurs during
* evaluation of the query.
* @exception AccessDeniedException In case the current user does not have
* read access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see QueryLanguage
*/
public void performGraphQuery(QueryLanguage language, String query, GraphQueryResultListener listener)
throws IOException, MalformedQueryException, QueryEvaluationException, AccessDeniedException;
/**
* Evaluates a graph query and reports the resulting statements to the
* specified listener.
*
* @param language The query language in which the query is formulated.
* Currently, only SeRQL supports graph queries and
* QueryLanguage.SERQL is the only legal value.
* @param query The query.
*
* @return A Graph object that contains the query result.
*
* @exception IOException In case an I/O error occurs while sending the
* query to, or retrieving the result from a Sesame server.
* @exception MalformedQueryException If the supplied query is malformed.
* @exception QueryEvaluationException If a problem occurs during
* evaluation of the query.
* @exception AccessDeniedException In case the current user does not have
* read access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see QueryLanguage
*/
public Graph performGraphQuery(QueryLanguage language, String query)
throws IOException, MalformedQueryException, QueryEvaluationException, AccessDeniedException;
/**
* Adds the RDF data that can be found at the specified URL to the
* repository.
*
* @param dataURL the URL of the RDF data.
* @param baseURI the base URI to resolve any relative URIs against.
* This defaults to the value of dataURL if the value is set
* to null.
* @param format the serialization format of the data (one of
* RDFFormat.RDFXML, RDFFormat.NTRIPLES).
* @param verifyData if set to 'true', the RDF parser will verify the
* data before adding it to the repository.
* @param listener an AdminListener that will receive error- and
* progress messages.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
*/
public void addData(URL dataURL, String baseURI, RDFFormat format, boolean verifyData, AdminListener listener)
throws IOException, AccessDeniedException;
/**
* Adds RDF data from the specified file to the repository.
*
* @param dataFile a file containing RDF data.
* @param baseURI the base URI to resolve any relative URIs against.
* @param format the serialization format of the data (one of
* RDFFormat.RDFXML, RDFFormat.NTRIPLES).
* @param verifyData if set to 'true', the RDF parser will verify the
* data before adding it to the repository.
* @param listener an AdminListener that will receive error- and
* progress messages.
*
* @exception FileNotFoundException In case the file could not be found.
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
*/
public void addData(File dataFile, String baseURI, RDFFormat format, boolean verifyData, AdminListener listener)
throws FileNotFoundException, IOException, AccessDeniedException;
/**
* Adds an RDF document to the repository.
*
* @param data an RDF document.
* @param baseURI the base URI to resolve any relative URIs against.
* @param format the serialization format of the data (one of
* RDFFormat.RDFXML, RDFFormat.NTRIPLES).
* @param verifyData if set to 'true', the RDF parser will verify the
* data before adding it to the repository.
* @param listener an AdminListener that will receive error- and
* progress messages.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
*/
public void addData(String data, String baseURI, RDFFormat format, boolean verifyData, AdminListener listener)
throws IOException, AccessDeniedException;
/**
* Adds the contents of the supplied SesameRepository to this repository.
*
* @param repository a SesameRepository.
* @param listener an AdminListener that will receive error- and
* progress messages.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
*/
public void addData(SesameRepository repository, AdminListener listener)
throws IOException, AccessDeniedException;
/**
* Adds RDF data from a Reader to the repository. Note: uploading
* XML-encoded RDF using a Reader can destroy the character encoding
* of the data. Using an InputStream can prevent this.
*
* @param data a Reader from which RDF data can be read.
* @param baseURI the base URI to resolve any relative URIs against.
* @param format the serialization format of the data (one of
* RDFFormat.RDFXML, RDFFormat.NTRIPLES).
* @param verifyData if set to 'true', the RDF parser will verify the
* data before adding it to the repository.
* @param listener an AdminListener that will receive error- and
* progress messages.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
*/
public void addData(Reader data, String baseURI, RDFFormat format, boolean verifyData, AdminListener listener)
throws IOException, AccessDeniedException;
/**
* Adds RDF data from an InputStream to the repository.
*
* @param data an InputStream from which RDF data can be read.
* @param baseURI the base URI to resolve any relative URIs against.
* @param format the serialization format of the data (one of
* RDFFormat.RDFXML, RDFFormat.NTRIPLES).
* @param verifyData if set to 'true', the RDF parser will verify the
* data before adding it to the repository.
* @param listener an AdminListener that will receive error- and
* progress messages.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
*/
public void addData(InputStream data, String baseURI, RDFFormat format, boolean verifyData, AdminListener listener)
throws IOException, AccessDeniedException;
/**
* Adds the contents of the supplied graph to the repository. Blank nodes
* in the supplied graph that originate from this repository are reused.
*
* @param graph a Graph containing the RDF statements to be added.
*
* @throws IOException in case an I/O error occurs.
* @throws AccessDeniedException In case the current user does not have write access on
* this repository.
* @exception UnsupportedOperationException If this repository does not support the
* specified action.
* @see Graph
*/
public void addGraph(Graph graph) throws IOException, AccessDeniedException;
/**
* Adds the contents of the supplied graph to the repository.
*
* @param graph a Graph containing the RDF statements to be added.
* @param joinBlankNodes indicates adding behavior. If set to true, blank nodes
* in the supplied graph that originate from this repository are reused. If set to false,
* new blank nodes are created for each blank node in the supplied graph.
*
* @throws IOException in case an I/O error occurs.
* @throws AccessDeniedException In case the current user does not have write access on
* this repository.
* @exception UnsupportedOperationException If this repository does not support the
* specified action.
* @see Graph
*/
public void addGraph(Graph graph, boolean joinBlankNodes) throws IOException, AccessDeniedException;
/**
* Adds the contents of the graph specified by the supplied graph query to
* the repository. Blank nodes
* in the supplied graph that originate from this repository are reused.
*
* @param language the query language in which the query is formulated.
* @param query the query. Note that the query must be of a form that has
* an RDF graph as its result (e.g. a SeRQL CONSTRUCT query)
* @throws IOException in case an I/O error occurs
* @throws AccessDeniedException in case the current user does not have write access on
* this repository.
* @throws MalformedQueryException In case the supplied query is incorrect.
* @throws QueryEvaluationException In case an error occurs during query evaluation.
*/
public void addGraph(QueryLanguage language, String query)
throws IOException, AccessDeniedException;
/**
* Adds the contents of the graph specified by the supplied graph query to
* the repository.
*
* @param language the query language in which the query is formulated.
* @param query the query. Note that the query must be of a form that has
* an RDF graph as its result (e.g. a SeRQL CONSTRUCT query)
* @param joinBlankNodes indicates adding behavior. If set to true, blank nodes
* in the supplied graph that originate from this repository are reused. If set to false,
* new blank nodes are created for each blank node in the supplied graph.
*
* @throws IOException in case an I/O error occurs
* @throws AccessDeniedException in case the current user does not have write access on
* this repository.
* @throws MalformedQueryException In case the supplied query is incorrect.
* @throws QueryEvaluationException In case an error occurs during query evaluation.
*/
public void addGraph(QueryLanguage language, String query, boolean joinBlankNodes)
throws IOException, AccessDeniedException;
/**
* Removes the contents of the supplied graph from the repository. All statements in
* the graph are removed from the repository. Blank nodes in the supplied graph that
* originate from this repository are reused.
*
* @param graph a Graph containing RDF statements that are to be removed.
*
* @throws IOException in case an I/O error occurs.
* @throws AccessDeniedException In case the current user does not have write access on
* this repository.
* @exception UnsupportedOperationException If this repository does not support the
* specified action.
* @see Graph
*/
public void removeGraph(Graph graph) throws IOException, AccessDeniedException;
/**
* Removes the contents of the graph specified by the supplied graph query from
* the repository. Blank nodes in the supplied graph that originate from this
* repository are reused.
*
* @param language the query language in which the query is formulated.
* @param query the query. Note that the query must be of a form that has
* an RDF graph as its result (e.g. a SeRQL CONSTRUCT query)
* @throws IOException in case an I/O error occurs
* @throws AccessDeniedException in case the current user does not have write access on
* this repository.
*/
public void removeGraph(QueryLanguage language, String query)
throws IOException, AccessDeniedException;
/**
* Extracts data from the repository and returns it in the requested
* format. You can either extract the ontological data, the instance
* data, or both. For any of these, you can specify whether you want
* to extract all statements, or only the ones that were explicitly
* added.
*
* @param format The serialization format that should be used (one of
* RDFFormat.RDFXML, RDFFormat.NTRIPLES, RDFFormat.N3).
* @param ontology If true, the ontological part of the data will be
* extracted.
* @param instances If true, the instances part of the data will be
* extracted.
* @param explicitOnly If true, only explicitely added statements will
* be extracted, i.e. the inferred statements will be skipped.
* @param niceOutput If true, the server will try its best to make the
* data as much readable for humans as possible. Note that this will
* cost extra processing time, and it should be set to 'false' if not
* necessary.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* read access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
* @see RDFFormat
**/
public InputStream extractRDF(
RDFFormat format, boolean ontology, boolean instances, boolean explicitOnly, boolean niceOutput)
throws IOException, AccessDeniedException;
/**
* Removes statements from the repository. Statements that match the pattern
* specified by the parameters subject, predicate and
* object will be removed from the repository. null values
* for these parameters indicate wildcards. So calling
* removeStatements(mySubject, null, null, l) will remove all
* statements with a subject equal to mySubject.
*
* @param subject The subject of the statements that should be removed, or
* null if the subject is allowed to be anything.
* @param predicate The predicate of the statements that should be removed, or
* null if the predicate is allowed to be anything.
* @param object The object of the statements that should be removed, or
* null if the object is allowed to be anything.
* @param listener An AdminListener that will receive error- and progress
* messages.
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
*/
public void removeStatements(Resource subject, URI predicate, Value object, AdminListener listener)
throws IOException, AccessDeniedException;
/**
* Clears the repository. All statements will be removed.
*
* @param listener An AdminListener that will receive error- and
* progress messages.
*
* @exception IOException In case an I/O error occurs.
* @exception AccessDeniedException In case the current user does not have
* write access on this repository.
* @exception UnsupportedOperationException If this repository does not
* support the specified action.
*/
public void clear(AdminListener listener)
throws IOException, AccessDeniedException;
/**
* retrieves the repository id string for this repository.
*
* @return the id of the repository.
*/
public String getRepositoryId();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy