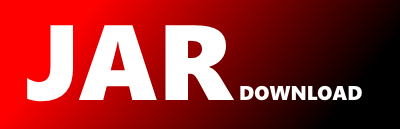
org.openrdf.sesame.sail.query.Intersect Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.sail.query;
import java.io.IOException;
import java.util.Set;
import org.openrdf.sesame.sail.RdfSource;
/**
* Determines the intersection of two sets of values that are produced
* by queries.
**/
public class Intersect extends SetOperator {
/**
* Creates a new Intersect object that only reports query answers that are
* present in the query answer sets of both the left- and right argument.
**/
public Intersect(Query leftArg, Query rightArg, boolean all) {
super(leftArg, rightArg, all);
}
// overrides Query.evaluate()
public void evaluate(RdfSource rdfSource, QueryAnswerListener listener)
throws SailQueryException
{
QueryAnswerCollector qac = new QueryAnswerCollector();
_rightArg.evaluate(rdfSource, qac);
if (!_all) {
listener = new DuplicatesFilter(listener);
}
listener = new IntersectionFilter(qac.getQueryAnswers(), listener);
_leftArg.evaluate(rdfSource, listener);
}
public String toString() {
return
"(" + _leftArg.toString() +
" INTERSECT " + (_all ? "ALL " : "") +
_rightArg.toString() + ")";
}
/*-------------------------------+
| inner class IntersectionFilter |
+-------------------------------*/
/**
* A QueryAnswerListener that filters out the answers that are not in
* the includeSet (an interect set-operation)
**/
private static class IntersectionFilter implements QueryAnswerListener {
/**
* Set of QueryAnswer objects which should not be filtered from the result.
**/
private Set _includeSet;
/**
* The listener to forward the filtered query results to.
**/
private QueryAnswerListener _listener;
/**
* Creates a new IntersectionFilter.
*
* @param includeSet A Set of QueryAnswer objects.
* @param l The QueryAnswerListener that will receive the filtered
* query results.
**/
public IntersectionFilter(Set includeSet, QueryAnswerListener l) {
_includeSet = includeSet;
_listener = l;
}
// implements QueryAnswerListener.queryAnswer(QueryAnswer)
public boolean queryAnswer(QueryAnswer qa)
throws IOException
{
if (_includeSet.contains(qa)) {
return _listener.queryAnswer(qa);
}
return true;
}
// implements QueryAnswerListener.clear()
public void clear() {
_listener.clear();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy