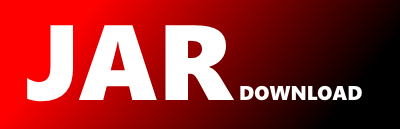
org.openrdf.sesame.sailimpl.omm.security.SecuritySail Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* OMM - Ontology Middleware Module
* Copyright (C) 2002 OntoText Lab, Sirma AI OOD
*
* Contact:
* Sirma AI OOD, OntoText Lab.
* 38A, Christo Botev Blvd.
* 1000 Sofia, Bulgaria
* tel. +359(2)981 00 18
* fax. +359(2)981 90 58
* [email protected]
*
* http://www.ontotext.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.sailimpl.omm.security;
import java.io.FileOutputStream;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import org.openrdf.util.jdbc.ConnectionPool;
import org.openrdf.util.log.ThreadLog;
import org.openrdf.vocabulary.RDF;
import org.openrdf.vocabulary.RDFS;
import org.openrdf.model.Graph;
import org.openrdf.model.Literal;
import org.openrdf.model.Resource;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import org.openrdf.model.ValueFactory;
import org.openrdf.model.impl.LiteralImpl;
import org.openrdf.model.impl.URIImpl;
import org.openrdf.model.impl.ValueFactoryImpl;
import org.openrdf.sesame.Sesame;
import org.openrdf.sesame.admin.XmlAdminMsgWriter;
import org.openrdf.sesame.constants.RDFFormat;
import org.openrdf.sesame.omm.SecurityServices;
import org.openrdf.sesame.omm.SessionContext;
import org.openrdf.sesame.omm.VersionManagement;
import org.openrdf.sesame.query.MalformedQueryException;
import org.openrdf.sesame.query.QueryErrorType;
import org.openrdf.sesame.query.TableQueryResultListener;
import org.openrdf.sesame.repository.local.LocalRepository;
import org.openrdf.sesame.repository.local.LocalService;
import org.openrdf.sesame.sail.LiteralIterator;
import org.openrdf.sesame.sail.NamespaceIterator;
import org.openrdf.sesame.sail.RdfRepository;
import org.openrdf.sesame.sail.RdfSchemaRepository;
import org.openrdf.sesame.sail.RdfSchemaSource;
import org.openrdf.sesame.sail.RdfSource;
import org.openrdf.sesame.sail.Sail;
import org.openrdf.sesame.sail.SailChangedListener;
import org.openrdf.sesame.sail.SailInitializationException;
import org.openrdf.sesame.sail.SailInternalException;
import org.openrdf.sesame.sail.SailUpdateException;
import org.openrdf.sesame.sail.StackedSail;
import org.openrdf.sesame.sail.StatementIterator;
import org.openrdf.sesame.sail.query.Query;
import org.openrdf.sesame.sailimpl.rdbms.RdbmsNamespace;
/* Jena depndency
import com.hp.hpl.mesa.rdf.jena.mem.*;
import com.hp.hpl.mesa.rdf.jena.common.prettywriter.*;
*/
/**
* SecuritySail.java
*
* Title: Knowledge Control System
*
*
*
*
* Company: OntoText Lab. Sirma AI.
*
*
* @author borislav popov
* @version 1.0 Security Sail's purpose is to provide a fine grained security
* filtering layer over an RDF repository (e.g. Sesame) in a stacked
* sails architecture.
*
* TODO:
*
* - PAUSED : getDomain,getRange :is properly filtered ?
*
- remove the literal set object method from the pattern
* restrictions
*
- BUG in the WEB interface: when selecting Extract data from the
* repository
*
- isValueAccessible implement fully : now only for resource
*
- QueryRestrictions : is correctly implemented? is defined to
* restrict statements but is used to restrict resources
*
*/
public class SecuritySail
implements RdfSchemaRepository, StackedSail,
SecurityServices, VersionManagement, TableQueryResultListener
{
/** the transient triples for the current transaction */
private ArrayList transients = new ArrayList();
private static int lastResourceId = 0;
private static int lastLiteralId = 0;
/*
* the following base references are either null either are casted to the
* type specified but all are one and the same object
*/
/** base Sail */
private Sail baseSail = null;
/** base RdfSchemaSource */
private RdfSchemaSource baseRdfSchemaSource = null;
/** base RdfRepository */
private RdfRepository baseRdfRepository = null;
/** base RdfSource */
private RdfSource baseRdfSource = null;
/** base VersionManagement Sail */
private VersionManagement versionMngmt = null;
/** the current query results table consisting of Values */
private ArrayList queryResult = new ArrayList();
/** Maps executed queries vs. returned results list */
Map queryResults = new HashMap();
/**
* flag to indicate the status of the query: whether it is ready or is being
* used currently
*/
boolean isQueryReady = true;
/** the class of the VersionManagement interface */
private final static String VERSION_SAIL = "org.openrdf.sesame.omm.VersionManagement";
/* > demo data related constants */
private final static String SKILL = "http://www.ontotext.com/otk/2002/05/skills.rdfs#Skill";
private final static String SKILL_LEVEL =
"http://www.ontotext.com/otk/2002/05/skills.rdfs#SkillLevel";
private final static String BUS_SKILL =
"http://www.ontotext.com/otk/2002/05/sirma_skills_hier.rdfs#BusinessSkill";
private final static String ESPIONAGE_SKILL =
"http://www.ontotext.com/otk/2002/05/sirma_skills_hier.rdfs#BusinessEspionage";
private final static String TECH_SKILL =
"http://www.ontotext.com/otk/2002/05/sirma_skills_hier.rdfs#TechnicalSkill";
private final static String SWING_SKILL =
"http://www.ontotext.com/otk/2002/05/sirma_skills_hier.rdfs#Swing";
private final static String DEMO_SWING_SKILL =
"http://www.ontotext.com/otk/2002/05/sirma_enter_kb.rdf#DemoSwingSkill";
private final static String RDF_TYPE =
"http://www.w3.org/1999/02/22-rdf-syntax-ns#type";
private final static String RESOURCE = "http://www.w3.org/2000/01/rdf-schema#Resource";
private final static String PERSON ="http://www.ontotext.com/otk/2002/05/enterprise.rdfs#Person";
private final static String HAS_SKILL = "http://www.ontotext.com/otk/2002/05/skills.rdfs#hasSkill";
private final static String HAS_POSITION = "http://www.ontotext.com/otk/2002/05/enterprise.rdfs#hasPosition";
private final static String SUB_CLASS_OF = "http://www.w3.org/2000/01/rdf-schema#subClassOf";
private final static String AI_SKILL_QUERY = "select * from {X} http://www.w3.org/2000/01/rdf-schema#subClassOf {Y} where X = http://www.ontotext.com/otk/2002/05/sirma_skills_hier.rdfs#AISkill";
private final static String HAS_POSITION_QUERY = "select * from {X} http://www.ontotext.com/otk/2002/05/enterprise.rdfs#hasPosition {Y}";
private final static String AI_SKILL ="http://www.ontotext.com/otk/2002/05/sirma_skills_hier.rdfs#AISkill";
private final static String MITAC ="http://www.ontotext.com/otk/2002/05/sirma_enter_kb.rdf#DimitarManov";
/* > db related constants */
private final static String KCS_URI = "http://www.ontotext.com/otk/2002/03/KCS.rdfs";
private final static String BASE_KCS_URI = "http://www.ontotext.com/otk/2002/03/kcs.rdfs#";
/** Name of table containing Users. */
public static final String USERS_TABLE = "users";
/** Name of table containing Users' roles. */
public static final String USERS_ROLES_TABLE = "users_roles";
/** Name of table containing Users' Rules. */
public static final String USERS_RULES_TABLE = "users_rules";
/** Name of table containing Roles. */
public static final String ROLES_TABLE = "roles";
/** Name of table containing Roles Hierarchy. */
public static final String ROLES_HIERARCHY_TABLE = "roles_hierarchy";
/** Name of table containing Roles' Rules. */
public static final String ROLES_RULES_TABLE = "roles_rules";
/** Name of table containing Security Rules. */
public static final String SECURITY_RULES_TABLE = "security_rules";
/** Name of table containing Restrictions. */
public static final String RESTRICTIONS_TABLE = "restrictions";
/** Name of table containing Resource Or Properties Restrictions. */
public static final String RES_PROP_RESTRS_TABLE = "res_propr_restrs";
/** Name of table containing Pattern Restrictions. */
public static final String PATTERN_RESTRS_TABLE = "pattern_restrs";
/** Name of table containing Query Restrictions. */
public static final String QUERY_RESTRS_TABLE = "query_restrs";
/** Name of table containing Resources. */
public static final String RESOURCES_TABLE = "resources";
/** Name of table containing Literals. */
public static final String LITERALS_TABLE = "literals";
/** Name of table containing Namespaces. */
public static final String NAMESPACES_TABLE = "namespaces";
/** drop table statement */
public static final String DROP_TABLE = "DROP TABLE ";
/** select * from statement */
public static final String SELECT = "SELECT * FROM ";
/** INSERT INTO statement */
public static final String INSERT = "INSERT INTO ";
/** VALUES part of statement */
public static final String VALUES = " VALUES ";
/** Integer datatype. */
protected final static String INT = "INTEGER UNSIGNED";
/** character 20 datatype */
protected final static String CHR20 = "character varying(20)";
/** character 255 datatype */
protected final static String CHR255 = "character varying(255)";
/* < db related constants */
/** a map of uris vs resource ids */
static Map resIdsByUri = new HashMap();
/** conection pool */
protected ConnectionPool conPool = null;
/* > Security Services implemenation related members */
/** the set of users */
private Set users = new HashSet();
/** the map of users' logins vs users objects */
private Map usersByLogin = new HashMap();
/** the map of users by id */
private Map usersById = new HashMap();
/** the set of security roles */
private Set roles = new HashSet();
/** the map of roles' ids vs roles objects */
private Map rolesById = new HashMap();
/** the map of roles' names vs roles objects */
private Map rolesByName = new HashMap();
/** the map of restriction ids vs. restriction objects */
private Map restrictionsById = new HashMap();
/** the map of rules ids vs security rules objects. */
private Map rulesById = new HashMap();
/* < Security Services implemenation related members */
/** Resources by Id map */
private Map resById = new HashMap();
/** Ids by Resources map */
private Map idByRes = new HashMap();
/** the last resource id that has been used */
private int lastResId = 0;
/** Namespaces by Id map */
private Map namespaces = new HashMap();
/** Literals by Id map */
private Map literalById = new HashMap();
/** Ids by Literals map */
private Map idByLiteral = new HashMap();
public SecuritySail() {
}
/* > */
/* > RDFSchemaSource implementation */
public StatementIterator getExplicitStatements(
Resource subj, URI pred, Value obj)
{
StatementIterator si = baseRdfSchemaSource.getExplicitStatements(subj,pred,obj);
try {
si = new StatementFilterIterator(si,this,Right.READ);
}
catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public boolean hasExplicitStatement(Resource subj, URI pred, Value obj)
{
boolean has = baseRdfSchemaSource.hasExplicitStatement(subj,pred,obj);
if ( has ) {
has = isStatementAccessible(subj,pred,obj,Right.READ);
}
return has;
}
public Query optimizeQuery(Query qc) {
return qc;
}
public StatementIterator getClasses() {
StatementIterator si = baseRdfSchemaSource.getClasses();
try {
si = new StatementFilterIterator(si,this,Right.READ);
} catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public boolean isClass(Resource resource) {
boolean is = baseRdfSchemaSource.isClass(resource);
if ( is ) {
is = is && isResourceAccessible(resource);
}
return is;
}
public StatementIterator getProperties() {
StatementIterator si = baseRdfSchemaSource.getProperties();
return si;
}
public boolean isProperty(Resource resource) {
boolean is = baseRdfSchemaSource.isProperty(resource);
if ( is ) {
is = is && isResourceAccessible(resource);
}
return is;
}
public StatementIterator getSubClassOf(Resource subClass, Resource superClass) {
StatementIterator si = baseRdfSchemaSource.getSubClassOf(subClass, superClass);
try {
si = new StatementFilterIterator(si, this, Right.READ);
}
catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public StatementIterator getDirectSubClassOf(Resource subClass, Resource superClass) {
StatementIterator si = baseRdfSchemaSource.getDirectSubClassOf(subClass, superClass);
try {
si = new StatementFilterIterator(si, this, Right.READ);
} catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public boolean isSubClassOf(Resource subClass, Resource superClass) {
boolean is = baseRdfSchemaSource.isSubClassOf(subClass,superClass);
if ( is ) {
is = is && isResourceAccessible(subClass);
is = is && isResourceAccessible(superClass);
}
return is;
}
public boolean isDirectSubClassOf(Resource subClass, Resource superClass) {
boolean is = baseRdfSchemaSource.isDirectSubClassOf(subClass,superClass);
if ( is ) {
is = is && isResourceAccessible(subClass);
is = is && isResourceAccessible(superClass);
}
return is;
}
public StatementIterator getSubPropertyOf(Resource subProperty, Resource superProperty) {
StatementIterator si = baseRdfSchemaSource.getSubPropertyOf(subProperty, superProperty);
try {
si = new StatementFilterIterator(si, this, Right.READ);
} catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public StatementIterator getDirectSubPropertyOf(Resource subProperty, Resource superProperty) {
StatementIterator si = baseRdfSchemaSource.getDirectSubPropertyOf(subProperty, superProperty);
try {
si = new StatementFilterIterator(si, this, Right.READ);
} catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public boolean isSubPropertyOf(Resource subProperty, Resource superProperty) {
boolean is = baseRdfSchemaSource.isSubPropertyOf(subProperty,superProperty);
if ( is ) {
is = is && isResourceAccessible(subProperty);
is = is && isResourceAccessible(superProperty);
}
return is;
}
public boolean isDirectSubPropertyOf(Resource subProperty, Resource superProperty) {
boolean is = baseRdfSchemaSource.isDirectSubPropertyOf(subProperty,superProperty);
if ( is ) {
is = is && isResourceAccessible(subProperty);
is = is && isResourceAccessible(superProperty);
}
return is;
}
public StatementIterator getDomain(Resource prop, Resource domain) {
StatementIterator si = baseRdfSchemaSource.getDomain(prop, domain);
try {
si = new StatementFilterIterator(si, this, Right.READ);
}
catch (Exception e) {
throw new SailInternalException(e);
}
return si;
}
public StatementIterator getRange(Resource prop, Resource range) {
StatementIterator si = baseRdfSchemaSource.getRange(prop, range);
try {
si = new StatementFilterIterator(si, this, Right.READ);
}
catch (Exception e) {
throw new SailInternalException(e);
}
return si;
}
public StatementIterator getType(Resource anInstance, Resource aClass) {
StatementIterator si = baseRdfSchemaSource.getType(anInstance, aClass);
try {
si = new StatementFilterIterator(si, this, Right.READ);
}
catch (Exception e) {
throw new SailInternalException(e);
}
return si;
}
public StatementIterator getDirectType(Resource anInstance, Resource aClass) {
StatementIterator si = baseRdfSchemaSource.getDirectType(anInstance, aClass);
try {
si = new StatementFilterIterator(si, this, Right.READ);
}
catch (Exception e) {
throw new SailInternalException(e);
}
return si;
}
public boolean isType(Resource anInstance, Resource aClass) {
boolean is = false;
if (isResourceAccessible(anInstance) &&
isResourceAccessible(aClass))
{
is = baseRdfSchemaSource.isType(anInstance,aClass);
}
return is;
}
public boolean isDirectType(Resource anInstance, Resource aClass) {
boolean is = false;
if (isResourceAccessible(anInstance) &&
isResourceAccessible(aClass))
{
is = baseRdfSchemaSource.isDirectType(anInstance,aClass);
}
return is;
}
public LiteralIterator getLiterals(
String label, String language, URI datatype)
{
// FIXME: do we need to filter this?
return baseRdfSchemaSource.getLiterals(label, language, datatype);
}
/* < RDFSchemaSource implementation */
/* > RDFRepository Implementation */
public void startTransaction() {
baseRdfRepository.startTransaction();
// reset the query results since they might be inaccurate after the
// transaction
queryResults = new HashMap();
}
public void commitTransaction() {
String status = "" ;
if (areThereTransients()) {
// freeze THE UPDATE COUNTER
if (null != versionMngmt)
versionMngmt.pauseCounterIncrement();
baseRdfRepository.commitTransaction();
//start a new transaction to remove the irrelevant statements
baseRdfRepository.startTransaction();
status = removeIrrelevantTransients();
// unFreeze the COUNTER
if (null != versionMngmt)
versionMngmt.continueCounterIncrement();
} // if there are transinients
baseRdfRepository.commitTransaction();
if (status.length()>0) {
ThreadLog.trace(status);
throw new SailInternalException(status);
}
}
public boolean transactionStarted() {
return baseRdfRepository.transactionStarted();
}
public void addStatement(Resource subj, URI pred, Value obj) throws SailUpdateException {
baseRdfRepository.addStatement(subj,pred,obj);
if (!isStatementAccessible(subj,pred,obj,Right.ADD)) {
addTransient(subj,pred,obj);
throw new SailUpdateException(
"Statement Added temporarily.\n "+
"The permissions will be checked at the end of the transaction.");
}
}
// public boolean addStatement(Resource subj, URI pred, Value obj, boolean
// bExplicit) throws SailUpdateException {
// return baseRdfRepository.addStatement(subj,pred,obj,bExplicit);
// }
public int removeStatements(Resource subj, URI pred, Value obj) throws SailUpdateException {
StatementIterator si = baseRdfRepository.getStatements(subj,pred,obj);
StatementFilterIterator sfi = null;
try {
sfi = new StatementFilterIterator(si,this,Right.REMOVE);
} catch (Exception e) {
throw new SailInternalException(e);
}
int removed = 0;
while ( sfi.hasNext() ) {
org.openrdf.model.Statement st =
(org.openrdf.model.Statement) sfi.next();
removed += baseRdfRepository.removeStatements(
st.getSubject(),st.getPredicate(),st.getObject());
}
return removed;
}
public void clearRepository()
throws SailUpdateException
{
if (
isRepositoryAccessible(Right.REMOVE)
||
isRepositoryAccessible(Right.ADMIN)) {
baseRdfRepository.clearRepository();
} // if accessible
else {
throw new SailInternalException(
"Cannot Clear the Repository. Access Denied.");
}
}
public void changeNamespacePrefix(String namespace, String prefix) throws SailUpdateException {
baseRdfRepository.changeNamespacePrefix(namespace, prefix);
}
/* > RDFRepository Implementation */
/* > RDFSource Implementation */
public ValueFactory getValueFactory() {
//FIXME: implement
return null;
}
public StatementIterator getStatements(Resource subj, URI pred, Value obj) {
StatementIterator si = baseRdfSource.getStatements(subj,pred,obj);
try {
si = new StatementFilterIterator(si,this,Right.READ);
} catch (Exception e){
throw new SailInternalException(e);
}
return si;
}
public boolean hasStatement(Resource subj, URI pred, Value obj) {
boolean has = baseRdfSource.hasStatement(subj,pred,obj);
if ( has ) {
has = has && isStatementAccessible(subj,pred,obj,Right.READ);
}
return has;
}
public NamespaceIterator getNamespaces() {
return baseRdfSource.getNamespaces();
}
/* < RDFSource Implementation */
public void initialize(Map configParams) throws SailInitializationException {
ThreadLog.trace("initializing SecuritySail>>>");
// Get initialization parameters
String jdbcDriver = (String)configParams.get("jdbcDriver");
String jdbcUrl = (String)configParams.get("jdbcUrl");
String user = (String)configParams.get("user");
String password = (String)configParams.get("password");
String securitySetup = (String)configParams.get("security_setup");
if (jdbcDriver == null) {
throw new SailInitializationException("parameter 'jdbcDriver' missing");
}
if (jdbcUrl == null) {
throw new SailInitializationException("parameter 'jdbcUrl' missing");
}
if (user == null) {
throw new SailInitializationException("parameter 'user' missing");
}
if (password == null) {
throw new SailInitializationException("parameter 'password' missing");
}
// Load jdbc driver
try {
Class.forName(jdbcDriver);
}
catch (ClassNotFoundException e) {
throw new SailInitializationException(
"Unable to load JDBC-driver '" + jdbcDriver + "'", e);
}
// Create ConnectionPool
conPool = new ConnectionPool(jdbcUrl, user, password);
// Initialize database schema, if necessary
initDatabase();
Connection con = null;
java.sql.Statement st = null;
try {
con = conPool.getConnection();
st = con.createStatement();
loadNameSpaceTable(con,st);
loadResourcesTable(con,st);
loadLiteralsTable(con,st);
if ( null != securitySetup) {
ThreadLog.trace("Loading Security Policy from url == "+securitySetup);
/*
* IMPORT SECURITY SETUP USING THE JENA DEPENDENT IMPORT
* java.net.URL surl = new java.net.URL(securitySetup);
*
* BufferedReader br = new BufferedReader(new
* InputStreamReader(surl.openStream()));
* baseRdfRepository.startTransaction();
*
* importSecurityPolicy(br);
* baseRdfRepository.commitTransaction();
*/
/* import security setup using the in-memory sail import */
java.net.URL surl = new java.net.URL(securitySetup);
LocalService service = Sesame.getService();
LocalRepository repository = service.createRepository("tempSecurityRep", false);
repository.addData(surl, "", RDFFormat.RDFXML, true,
new XmlAdminMsgWriter(new FileOutputStream("ImportSecurityPolicy.Log")));
//org.openrdf.sesame.sailimpl.memory.RdfRepository memRepos;
//Map map = new HashMap();
//map.put("file","TempSecurityPolicy.nt");
//memRepos= new org.openrdf.sesame.sailimpl.memory.RdfRepository();
//memRepos.initialize(map);
//memRepos.clearRepository();
//RdfAdmin admin = new RdfAdmin(memRepos);
//admin.addRdfModel(surl.openStream(),securitySetup ,
// new XmlAdminMsgWriter(),
// true);
baseRdfRepository.startTransaction();
importPolicy(repository.getGraph());
baseRdfRepository.commitTransaction();
service.removeRepository(repository.getRepositoryId());
repository = null;
loadNameSpaceTable(con,st);
loadResourcesTable(con,st);
loadLiteralsTable(con,st);
//Store the setup to the repository
storeSecuritySetup();
}
else {
ThreadLog.trace("Loading Security Policy from the database");
loadSecuritySetup();
}
}
catch (Exception x) {
throw new SailInternalException("While reading the security setup.",x);
}
ThreadLog.trace("<< Version Management implementation */
public void labelState(long stateUID, String label) {
if ( isVersionTrackingAccessible() )
versionMngmt.labelState(stateUID, label);
}
public void labelCurrentState(String label) {
if ( isVersionTrackingAccessible() )
versionMngmt.labelCurrentState(label) ;
}
public void revertToState(long stateUID) {
if ( isVersionTrackingAccessible() )
versionMngmt.revertToState(stateUID);
}
public String branchState(long stateUID) {
String result = "";
if ( isVersionTrackingAccessible() )
result = versionMngmt.branchState(stateUID);
return result;
}
public void workWithState(long stateUID) {
if ( isVersionTrackingAccessible() )
versionMngmt.workWithState(stateUID);
}
public Iterator getVersions() {
Iterator i = new ArrayList().iterator();
if ( isVersionTrackingAccessible() )
i = versionMngmt.getVersions();
return i;
}
public void lockStatements(Iterator statementsList) {
if ( isVersionTrackingAccessible() )
versionMngmt.lockStatements(statementsList);
}
public void unlockStatements(Iterator statementsList) {
if ( isVersionTrackingAccessible() )
versionMngmt.unlockStatements(statementsList);
}
public Iterator getUpdateIds() {
Iterator i = new ArrayList().iterator();
if ( isVersionTrackingAccessible() )
i= versionMngmt.getUpdateIds();
return i;
}
public void pauseCounterIncrement() {
if ( isVersionTrackingAccessible() )
versionMngmt.pauseCounterIncrement();
}
public void continueCounterIncrement() {
if ( isVersionTrackingAccessible() )
versionMngmt.continueCounterIncrement();
}
public boolean isPausedCounterIncrement() {
if ( isVersionTrackingAccessible() )
return versionMngmt.isPausedCounterIncrement();
return false;
}
public Iterator getVersionIds() {
Iterator i = new ArrayList().iterator();
if ( isVersionTrackingAccessible() )
i= versionMngmt.getVersionIds();
return i;
}
/* MetaInfo Retrieval Methods */
public Map getMetaInfo(String subj, String pred, String obj) {
Map mi = new HashMap();
if ( isVersionTrackingAccessible() )
mi = versionMngmt.getMetaInfo(subj,pred,obj);
return mi;
}
public Map getUpdateMetaInfo(String updateId) {
Map mi = new HashMap();
if ( isVersionTrackingAccessible() )
mi = versionMngmt.getUpdateMetaInfo(updateId);
return mi;
}
public Map getVersionMetaInfo(String versionId) {
Map mi = new HashMap();
if ( isVersionTrackingAccessible() )
mi = versionMngmt.getVersionMetaInfo(versionId);
return mi;
}
/* < Version Management implementation */
/* > SecurityServices implementation */
public int getResourceId(Resource res) throws SecurityException{
Integer i = (Integer)idByRes.get(res);
if (null == i) {
throw new SecurityException("Id not found for resource:\n" +res);
}
int ii = i.intValue();
return ii;
}
public Resource getResource(int id) {
Resource res = (Resource)resById.get(new Integer(id));
return res;
}
public boolean isVersionTrackingAccessible() {
return isRepositoryAccessible(Right.ADMIN) && versionMngmt != null;
}// isVersionTrackingAccessible(Right)
public boolean isSchemaAccessible(Right right) {
boolean result = false;
/* authenticate */
SessionContext context = SessionContext.getContext();
int userId = context.userID;
/* check */
User user = (User)usersById.get(new Integer(userId));
if (null!=user) {
Set rulz = user.getRules();
rulz.addAll(RoleImpl.getRules(user.getRoles(),false));
ArrayList rulis = new ArrayList(rulz);
for (int i = 0 ; i < rulis.size() ; i++ ) {
Rule ru = (Rule)rulis.get(i);
Restriction rst = ru.getRestriction();
if (rst.getType() != Restriction.REPOSITORY
&& rst.getType() != Restriction.SCHEMA) {
continue;
}
if ( right.equals(Right.READ) ) {
if (ru.getReadRight()) {
result = true;
break;
}
}
if ( right.equals(Right.ADD) ) {
if (ru.getAddRight()) {
result = true;
break;
}
}
if ( right.equals(Right.REMOVE) ) {
if (ru.getRemoveRight()) {
result = true;
break;
}
}
} // for rules
} // if user
return result;
} // isSchemaAccessible(Right)
public boolean isRepositoryAccessible(Right right) {
boolean result = false;
/* authenticate */
SessionContext context = SessionContext.getContext();
int userId = context.userID;
/* check */
User user = (User)usersById.get(new Integer(userId));
if (null!=user) {
Set rulz = user.getRules();
rulz.addAll(RoleImpl.getRules(user.getRoles(),false));
ArrayList rulis = new ArrayList(rulz);
for (int i = 0 ; i < rulis.size() ; i++ ) {
Rule ru = (Rule)rulis.get(i);
Restriction rst = ru.getRestriction();
if (rst.getType() != Restriction.REPOSITORY) {
continue;
}
if ( right.equals(Right.READ) ) {
if (ru.getReadRight()) {
result = true;
break;
}
}
if ( right.equals(Right.REMOVE) ) {
if (ru.getRemoveRight()) {
result = true;
break;
}
}
if ( right.equals(Right.ADMIN) ) {
if (ru.getAdminRight()) {
result = true;
break;
}
}
if ( right.equals(Right.HISTORY) ) {
if (ru.getHistoryRight()) {
result = true;
break;
}
}
} // for rules
} // if user
return result;
} // isRepositoryAccessible(Right)
public boolean isStatementAccessible(Resource subj, URI pred, Value obj, Right right) {
boolean result = false;
/* authenticate */
SessionContext context = SessionContext.getContext();
int userId = context.userID;
/* check */
User user = (User)usersById.get(new Integer(userId));
if (null!=user) {
Set rulz = user.getRules();
rulz.addAll(RoleImpl.getRules(user.getRoles(),false));
ArrayList rulis = new ArrayList(rulz);
for (int i = 0 ; i < rulis.size() ; i++ ) {
Rule ru = (Rule)rulis.get(i);
if (!ru.getAddRight() && !ru.getRemoveRight() && !ru.getReadRight()) {
continue;
}
if (right.equals(Right.READ) && !ru.getReadRight() ||
right.equals(Right.REMOVE) && !ru.getRemoveRight() ||
right.equals(Right.ADD) && !ru.getAddRight())
{
continue;
}
Restriction rst = ru.getRestriction();
if (rst.type == Restriction.REPOSITORY ) {
// OPTIMIZE
result = true;
break;
}
if (rst.type == Restriction.SCHEMA) {
/* check if the subject is part of the schema */
if (baseRdfSchemaSource.isType(subj,URIImpl.RDFS_CLASS) ||
baseRdfSchemaSource.isType(subj,URIImpl.RDF_PROPERTY))
{
result = true;
break;
} // if
} // schema
/*
* CHECK SUBJECT : IF ACCESSIBLE : THEN THE WHOLE STATEMENT IS
* ACCESSIBLE
*/
if (isResourceAccessible(subj,right)) {
result = true;
break;
}
/* PROPERTIES */
if (rst.type == Restriction.PROPERTIES) {
PropertiesRestriction propr = (PropertiesRestriction) rst;
ArrayList props = new ArrayList(propr.getProperties());
for (int pi = 0 ; pi < props.size() ; pi++ ) {
Resource prop = (Resource)props.get(pi);
if ( baseRdfSchemaSource.isSubPropertyOf(pred,prop) ) {
result = true;
break;
}
} // for properties
if ( result ) break;
} // Properties
/* PATTERN */
if (rst.type == Restriction.PATTERN ) {
boolean subjAccessible = true ;
boolean predAccessible = true ;
boolean objAccessible = true ;
PatternRestriction pat = (PatternRestriction) rst;
/* SUBJECT */
ArrayList subjs = new ArrayList( pat.getSubjectRestrictions());
for ( int si = 0 ; si < subjs.size() ; si++ ) {
subjAccessible = false;
ResourceRestriction sr = (ResourceRestriction)subjs.get(si);
ArrayList resList = new ArrayList(sr.getResources());
for ( int ri=0 ; ri < resList.size() ; ri++) {
Resource reso = (Resource) resList.get(ri);
if (sr.getType() == Restriction.CLASSES) {
if (baseRdfSchemaSource.isType(subj,reso)) {
subjAccessible = true;
break;
}
} else {
if (sr.getType() == Restriction.CLASSES_OVER_SCHEMA) {
if (baseRdfSchemaSource.isSubClassOf(subj,reso)) {
subjAccessible = true;
break;
}
} else {
if (sr.getType() == Restriction.INSTANCES) {
if (subj.equals(reso)) {
subjAccessible = true;
break;
}
} else {
throw new SailInternalException(
"Object Restrictions (part of the Pattern Restriction)\n "+
"should be of type ClassesRestriction or InstancesRestriction");
}
}
} // else
} // for reslist
if (subjAccessible) break;
} // for subjectrestrs
/* PREDICATE */
ArrayList preds = new ArrayList( pat.getPredicateRestrictions());
for ( int predi = 0 ; predi < preds.size() ; predi++ ) {
predAccessible = false;
PropertiesRestriction pr = (PropertiesRestriction)preds.get(predi);
ArrayList propList = new ArrayList(pr.getProperties());
for ( int propi = 0 ; propi < propList.size(); propi++) {
Resource prop = (Resource)propList.get(propi);
if ( baseRdfSchemaSource.isSubPropertyOf(pred,prop)){
predAccessible = true;
break;
}
} // for propi
if ( predAccessible ) break;
} // for predi
/* OBJECT */
ArrayList objs = new ArrayList( pat.getObjectRestrictions());
for ( int obji = 0 ; obji < objs.size() ; obji++ ) {
objAccessible = false;
Object objRestr = objs.get(obji);
if (objRestr instanceof Literal) {
Value val = (Value) objRestr;
if ( val.equals(obj)) {
objAccessible = true;
break;
}
} else {
if (objRestr instanceof ResourceRestriction) {
ResourceRestriction rr = (ResourceRestriction)objRestr;
ArrayList resList = new ArrayList(rr.getResources());
for ( int ri=0 ; ri < resList.size() ; ri++) {
Resource reso = (Resource) resList.get(ri);
if (rr.getType() == Restriction.CLASSES) {
if (obj instanceof Resource &&
baseRdfSchemaSource.isType((Resource)obj,reso))
{
objAccessible = true;
break;
}
}
else if (rr.getType() == Restriction.CLASSES_OVER_SCHEMA) {
if (obj instanceof Resource &&
baseRdfSchemaSource.isSubClassOf((Resource)obj,reso))
{
objAccessible = true;
break;
}
}
else if (rr.getType() == Restriction.INSTANCES) {
if (obj.equals(reso)) {
objAccessible = true;
break;
}
} // else
} // for reslist
} else {
throw new SailInternalException(
"The Object's Restrictions (in a Pattern restriction) should be \n"+
"either a Literal, either a ResourceRestriction.");
}
} //else
if ( objAccessible ) break;
} // for obji
if ( subjAccessible && predAccessible && objAccessible ) {
result = true;
break;
}
} // PATTERN
} //for all rulez
} // user not null
return result;
} //isStatementAccessible(Resource,URI,Value,Right)
public boolean isStatementAccessible(org.openrdf.model.Statement st,Right right) {
return isStatementAccessible(st.getSubject(),st.getPredicate(),st.getObject(),right);
} // isStatementAccessible(Statement,Right)
public boolean isValueAccessible(Value val) {
boolean is = true;
if ( val instanceof Resource) {
is = isResourceAccessible((Resource)val);
}
return is;
}
public boolean isResourceAccessible(Resource res, Right right) {
boolean result = false;
/* authenticate */
SessionContext context = SessionContext.getContext();
int userId = context.userID;
/* check */
User user = (User)usersById.get(new Integer(userId));
if (null!=user) {
Set rulz = user.getRules();
rulz.addAll(RoleImpl.getRules(user.getRoles(),false));
ArrayList rulis = new ArrayList(rulz);
for (int i = 0 ; i < rulis.size() ; i++ ) {
Rule ru = (Rule)rulis.get(i);
if (!ru.getAddRight() && !ru.getRemoveRight() && !ru.getReadRight()) {
continue;
}
if (right.equals(Right.READ) && !ru.getReadRight() ||
right.equals(Right.REMOVE) && !ru.getRemoveRight() ||
right.equals(Right.ADD) && !ru.getAddRight())
{
continue;
}
Restriction rst = ru.getRestriction();
if (rst.type == Restriction.REPOSITORY ) {
result = true;
break;
}
if (rst.type == Restriction.SCHEMA) {
/* check if the resource is part of the schema */
if (baseRdfSchemaSource.isType(res,URIImpl.RDFS_CLASS)
|| baseRdfSchemaSource.isType(res,URIImpl.RDF_PROPERTY)) {
result = true;
break;
} // if
} // schema
if (rst.type == Restriction.CLASSES){
ClassesRestriction cr = (ClassesRestriction) rst;
ArrayList classes = new ArrayList(cr.getResources());
for (int j=0; j < classes.size() ; j++) {
Resource clas = (Resource)classes.get(j);
if (baseRdfSchemaSource.isType(res,clas)) {
result = true;
break;
}
} // for classes
if (result) break;
} //if classes
if (rst.type == Restriction.CLASSES_OVER_SCHEMA){
ResourceRestriction rr = (ResourceRestriction) rst;
ArrayList classes = new ArrayList(rr.getResources());
for (int j=0; j < classes.size() ; j++) {
Resource clas = (Resource)classes.get(j);
if (baseRdfSchemaSource.isSubClassOf(res,clas)) {
result = true;
break;
}
} // for classes
if (result) break;
} //if classes over schema
if (rst.type == Restriction.INSTANCES){
InstancesRestriction ir = (InstancesRestriction) rst;
ArrayList instances = new ArrayList(ir.getResources());
for (int j=0; j < instances.size() ; j++) {
URI clas = (URI)instances.get(j);
if (res instanceof URI) {
if ( ((URI)res).getLocalName().equals(clas.getLocalName()) &&
((URI)res).getNamespace().equals(clas.getNamespace()) )
{
result = true;
break;
}
}
else {
// FIXME what to do for bNodes?
}
} // for instances
if (result) break;
} //if instances
if (rst.type == Restriction.QUERY) {
result = isQuAccessible((QueryRestriction) rst, res);
if (result) break;
}
} //for all rulez
} // user not null
return result;
} // isResourceAccessible(Resource,Right)
public boolean isResourceAccessible(Resource res) {
return isResourceAccessible(res,Right.READ);
}
public void addUser(int id, String login, String password, String name) {
try {
User user = new UserImpl(id,login,password,name);
users.add(user);
usersByLogin.put(login,user);
usersById.put(new Integer(id),user);
} catch (Exception e) {
throw new SailInternalException(e);
}
}
public void removeUser(String login) {
User user = (User)usersByLogin.get(login);
if (null!=user) {
usersByLogin.remove(login);
users.remove(user);
}
}
public Set getUsers() {
return users;
}
public User getUser(String login) {
return (User)usersByLogin.get(login);
}
public User getUser(int id) {
return (User)usersById.get(new Integer(id));
}
public Role createRole(int id, String name, String description, Set parents) {
try {
Role role = new RoleImpl(id,name,description);
role.setParentRoles(parents);
roles.add(role);
rolesByName.put(name,role);
return role;
} catch (Exception e) {
throw new SailInternalException(e);
}
}
public void removeRole(String name) {
Role role = (Role)rolesByName.get(name);
if(null!=role) {
rolesById.remove(new Integer(role.getId()));
rolesByName.remove(name);
roles.remove(role);
}
}
public void removeRole(int id) {
Role role = (Role)rolesById.get(new Integer(id));
if(null!=role) {
rolesById.remove(new Integer(id));
rolesByName.remove(role.getName());
roles.remove(role);
}
}
public Set getRoles() {
return roles;
}
public Role getRole(String name) {
return (Role)rolesByName.get(name);
}
public Role getRole(int id) {
return (Role)rolesById.get(new Integer(id));
} // main
public Restriction createRestriction(
int id, int type, String name, String description) throws NullParameterException{
Restriction restr = null;
switch(type){
case Restriction.REPOSITORY : {
restr = Restriction.createRepositoryRestriction(id,name,description);
break;
}
case Restriction.SCHEMA : {
restr = Restriction.createSchemaRestriction(id,name,description);
break;
}
case Restriction.QUERY : {
restr = Restriction.createQueryRestriction(id,name,description);
break;
}
case Restriction.INSTANCES : {
restr = Restriction.createInstancesRestriction(id,name,description);
break;
}
case Restriction.PATTERN : {
restr = Restriction.createPatternRestriction(id,name,description);
break;
}
case Restriction.PROPERTIES : {
restr = Restriction.createPropertiesRestriction(id,name,description);
break;
}
case Restriction.CLASSES : {
restr = Restriction.createClassesRestriction(id,name,description);
break;
}
case Restriction.CLASSES_OVER_SCHEMA : {
restr = Restriction.createClassesOverSchemaRestriction(id,name,description);
break;
}
default: {
throw new SailInternalException("Unknown restriction type ["+type+"].");
}
} // switch
restrictionsById.put(new Integer(id),restr);
return restr;
}// createRestriction(int,int,string,string)
public Restriction getRestriction(int id) {
return (Restriction) restrictionsById.get(new Integer(id));
}
/**
* Exports the Security Policy to a Sail. To be used with an In-Memory sail
* for example (org.openrdf.sesame.sail.memory.RdfRepository).
*
* @param repos the RdfRepository to export to
*/
public void exportPolicy(RdfRepository repos){
// definitions of resources used to describe the policy
URI proId = new URIImpl(BASE_KCS_URI+"id");
URI proName = new URIImpl(BASE_KCS_URI+"name");
URI proDescr = new URIImpl(BASE_KCS_URI+"descritpion");
URI proSuperRole = new URIImpl(BASE_KCS_URI+"superRole");
URI proIncludeRule = new URIImpl(BASE_KCS_URI+"includeRule");
URI proRuleRestriction = new URIImpl(BASE_KCS_URI+"ruleRestriction");
URI proRightsGranted = new URIImpl(BASE_KCS_URI+"rightsGranted");
URI proRestrictionType = new URIImpl(BASE_KCS_URI+"restrictionType");
URI proIncludeProperty = new URIImpl(BASE_KCS_URI+"includeProperty");
URI proSubjectRestr = new URIImpl(BASE_KCS_URI+"subjectRestr");
URI proPredicateRestr = new URIImpl(BASE_KCS_URI+"predicateRestr");
URI proObjectRestr = new URIImpl(BASE_KCS_URI+"objectRestr");
URI proRestrOnQuery = new URIImpl(BASE_KCS_URI+"restrOnQuery");
URI proIncludeResource = new URIImpl(BASE_KCS_URI+"includeResource");
URI proRdfType = new URIImpl(RDF.TYPE);
URI proHasRole = new URIImpl(BASE_KCS_URI+"hasRole");
URI proHasRule = new URIImpl(BASE_KCS_URI+"hasRule");
URI resRole = new URIImpl(BASE_KCS_URI+"Role");
URI resRule = new URIImpl(BASE_KCS_URI+"SecurityRule");
URI resRepositRestr = new URIImpl(BASE_KCS_URI+"RepositoryRestriction");
URI resSchemaRestr = new URIImpl(BASE_KCS_URI+"SchemaRestriction");
URI resClassesRestr = new URIImpl(BASE_KCS_URI+"ClassesRestriction");
URI resInstancesRestr = new URIImpl(BASE_KCS_URI+"InstancesRestriction");
URI resClassesOverSchemaRestr = new URIImpl(BASE_KCS_URI+"ClassesOverSchemaRestriction");
URI resPaternRestr = new URIImpl(BASE_KCS_URI+"PatternRestriction");
URI resPropertiesRestr = new URIImpl(BASE_KCS_URI+"PropertiesRestriction");
URI resQueryRestr = new URIImpl(BASE_KCS_URI+"QueryRestriction");
URI resUser = new URIImpl(BASE_KCS_URI+"User");
URI resRestriction = new URIImpl(BASE_KCS_URI+"Restriction");
repos.startTransaction();
try {
repos.clearRepository();
}
catch (SailUpdateException e) {
throw new RuntimeException(e);
}
repos.commitTransaction();
ArrayList userz = new ArrayList(users);
ArrayList queList = new ArrayList();
ArrayList rolz = new ArrayList(roles);
Set rulez = new HashSet(RoleImpl.getRules(roles,false));
try {
for ( int ui = 0; uirestriction.length()) {
*
* int id = Integer.parseInt(
* model.listObjectsOfProperty(subj,proId).next().toString() );
*
* String name = model.listObjectsOfProperty(subj,proName).next().toString();
* String descr = model.listObjectsOfProperty(subj,proDescr).next().toString();
*
*
* int intType = Restriction.type2Int(type);
*
* Restriction restr = (Restriction)uriVsRestr.get(subj.getURI()); if (null==
* restr) { restr = Restriction.createRestriction(intType,id); }
* restr.setName(name); restr.setDescription(descr);
* uriVsRestr.put(subj.getURI(),restr);
*
* switch (intType) { case Restriction.INSTANCES : {
* com.hp.hpl.mesa.rdf.jena.model.NodeIterator clses =
* model.listObjectsOfProperty(subj,proIncludeResource); Set resSet = new
* HashSet(); while (clses.hasNext()) { Resource cls = new Resource(
* ((com.hp.hpl.mesa.rdf.jena.model.Resource ) clses.next()).getURI());
* baseRdfRepository.addStatement(cls,resRdfType,resRdfResource); }
* ((ResourceRestriction)restr).setResources(resSet); break; } case
* Restriction.PROPERTIES : { com.hp.hpl.mesa.rdf.jena.model.NodeIterator pies =
* model.listObjectsOfProperty(subj,proIncludeProperty); Set propList = new
* HashSet(); while (pies.hasNext()) { Resource py = new Resource(
* ((com.hp.hpl.mesa.rdf.jena.model.Resource ) pies.next()).getURI());
* baseRdfRepository.addStatement(py,resRdfType,resRdfResource);
* propList.add(py); } ((PropertiesRestriction)restr).setProperties(propList);
* break; }
*
* case Restriction.CLASSES : { com.hp.hpl.mesa.rdf.jena.model.NodeIterator
* clses = model.listObjectsOfProperty(subj,proIncludeResource); Set resSet =
* new HashSet(); while (clses.hasNext()) { Resource cls = new Resource(
* ((com.hp.hpl.mesa.rdf.jena.model.Resource ) clses.next()).getURI());
* baseRdfRepository.addStatement(cls,resRdfType,resRdfResource);
*
* resSet.add(cls); } ((ResourceRestriction)restr).setResources(resSet); break; }
* case Restriction.CLASSES_OVER_SCHEMA : {
* com.hp.hpl.mesa.rdf.jena.model.NodeIterator clses =
* model.listObjectsOfProperty(subj,proIncludeResource); Set resSet = new
* HashSet(); while (clses.hasNext()) { Resource cls = new Resource(
* ((com.hp.hpl.mesa.rdf.jena.model.Resource ) clses.next()).getURI());
* baseRdfRepository.addStatement(cls,resRdfType,resRdfResource);
*
* resSet.add(cls); } ((ResourceRestriction)restr).setResources(resSet); break; }
* case Restriction.PATTERN : { // subject restrictions
* com.hp.hpl.mesa.rdf.jena.model.NodeIterator subjI =
* model.listObjectsOfProperty(subj,proSubjectRestr); while (subjI.hasNext()) {
*
* com.hp.hpl.mesa.rdf.jena.model.Resource rdfRes =
* ((com.hp.hpl.mesa.rdf.jena.model.Resource ) subjI.next()); Resource cls = new
* Resource(rdfRes.getURI());
* baseRdfRepository.addStatement(cls,resRdfType,resRdfResource);
*
* com.hp.hpl.mesa.rdf.jena.model.NodeIterator restrTypesI =
* model.listObjectsOfProperty(rdfRes,proRdfType); String rType = ""; while
* (restrTypesI.hasNext()){ rType = restrTypesI.next().toString(); if
* (rType.indexOf(restriction)!=-1) break; }
*
* Restriction sRestr = (Restriction)uriVsRestr.get(cls.getURI()); if
* (sRestr==null) { rType =
* model.listObjectsOfProperty(rdfRes,proRestrictionType).next().toString(); int
* sRestrId = Integer.parseInt(
* model.listObjectsOfProperty(rdfRes,proId).next().toString() );
*
* sRestr = Restriction.createRestriction(Restriction.type2Int(rType),sRestrId );
* uriVsRestr.put(cls.getURI(),sRestr); } ((PatternRestriction)
* restr).addSubjectRestriction((ResourceRestriction)sRestr);
* }
* // predicate restrs com.hp.hpl.mesa.rdf.jena.model.NodeIterator predI =
* model.listObjectsOfProperty(subj,proPredicateRestr); Set predRestrSet = new
* HashSet(); while (predI.hasNext()) { com.hp.hpl.mesa.rdf.jena.model.Resource
* rdfRes = ((com.hp.hpl.mesa.rdf.jena.model.Resource ) predI.next()); Resource
* cls = new Resource(rdfRes.getURI());
* baseRdfRepository.addStatement(cls,resRdfType,resRdfResource);
*
* com.hp.hpl.mesa.rdf.jena.model.NodeIterator restrTypesI =
* model.listObjectsOfProperty(rdfRes,proRdfType); String rType = ""; while
* (restrTypesI.hasNext()){ rType = restrTypesI.next().toString(); if
* (rType.indexOf(restriction)!=-1) break; }
*
* Restriction sRestr = (Restriction)uriVsRestr.get(cls.getURI()); if
* (sRestr==null) { rType =
* model.listObjectsOfProperty(rdfRes,proRestrictionType).next().toString(); int
* sRestrId = Integer.parseInt(
* model.listObjectsOfProperty(rdfRes,proId).next().toString() ); sRestr =
* Restriction.createRestriction(Restriction.type2Int(rType),sRestrId);
* uriVsRestr.put(cls.getURI(),sRestr); } ((PatternRestriction)
* restr).addPredicateRestriction((PropertiesRestriction)sRestr); }
* // object restrictions com.hp.hpl.mesa.rdf.jena.model.NodeIterator objI =
* model.listObjectsOfProperty(subj,proObjectRestr); Set objRestrSet = new
* HashSet(); while (objI.hasNext()) {
*
* com.hp.hpl.mesa.rdf.jena.model.Resource rdfRes =
* ((com.hp.hpl.mesa.rdf.jena.model.Resource ) objI.next()); Resource cls = new
* Resource(rdfRes.getURI());
* baseRdfRepository.addStatement(cls,resRdfType,resRdfResource);
*
* objRestrSet.add(cls);
*
* com.hp.hpl.mesa.rdf.jena.model.NodeIterator restrTypesI =
* model.listObjectsOfProperty(rdfRes,proRdfType); String rType = ""; while
* (restrTypesI.hasNext()){ rType = restrTypesI.next().toString(); if
* (rType.indexOf(restriction)!=-1) break; }
*
* Restriction sRestr = (Restriction)uriVsRestr.get(cls.getURI()); if
* (sRestr==null) { rType =
* model.listObjectsOfProperty(rdfRes,proRestrictionType).next().toString(); int
* sRestrId = Integer.parseInt(
* model.listObjectsOfProperty(rdfRes,proId).next().toString() );
*
* sRestr = Restriction.createRestriction(Restriction.type2Int(rType),sRestrId);
* uriVsRestr.put(cls.getURI(),sRestr); } ((PatternRestriction)
* restr).addObjectRestriction((ResourceRestriction)sRestr); } break; }
*
* case Restriction.QUERY : { com.hp.hpl.mesa.rdf.jena.model.NodeIterator queryI =
* model.listObjectsOfProperty(subj,proRestrOnQuery); Set qSet = new HashSet();
* while (queryI.hasNext()) { String q = queryI.next().toString();
* ((QueryRestriction)restr).addQuery(q); } break; }
* } } // Restriction
* } //.. if node is resource } // for all types } // while there are subjects
*
* // refresh the security maps rulesById = new HashMap(); ArrayList vlist =
* new ArrayList(uriVsRule.values()); for ( int i = 0 ; i < vlist.size(); i++ ) {
* Rule r = (Rule) vlist.get(i); rulesById.put(new Integer(r.getId()),r); }
*
* restrictionsById = new HashMap(); vlist = new ArrayList(uriVsRestr.values());
* for ( int i = 0 ; i < vlist.size(); i++ ) { Restriction r = (Restriction)
* vlist.get(i); restrictionsById.put(new Integer(r.getId()),r); }
*
* rolesByName = new HashMap(); rolesById = new HashMap(); roles = new
* HashSet(); vlist = new ArrayList(uriVsRole.values()); for ( int i = 0 ; i <
* vlist.size(); i++ ) { Role r = (Role) vlist.get(i); rolesById.put(new
* Integer(r.getId()),r); rolesByName.put(r.getName(),r); roles.add(r); }
* } catch (com.hp.hpl.mesa.rdf.jena.model.RDFException ex) { throw new
* SailInternalException(ex); } catch ( Exception x) { throw new
* SailInternalException(x); } catch ( Exception x) { throw new
* SailInternalException( "Wrong format of the imported RDF reader",x); }
* }
*/
/* < SecurityServices implementation */
/* > TableQueryResultListener implementation */
public void startTableQueryResult() throws IOException {
}
public void startTableQueryResult(String[] columnHeaders)
throws IOException {
queryResult = new ArrayList();
isQueryReady = false;
}
public void endTableQueryResult() throws IOException {
isQueryReady = true;
}
public void startTuple() throws IOException {
}
public void endTuple() throws IOException {
}
public void tupleValue(Value value) throws IOException {
queryResult.add(value);
}
public void error(QueryErrorType errType, String msg) throws IOException {
throw new SailInternalException(msg);
}
/* < TableQueryResultListener implementation */
/* > Addition of Statements Handling */
/**
* Adds transient statement. The added statements will be revised on commit
* of the current transaction. Then their relevance to the security setup
* will be tested and the irrelevant will be deleted in a new transaction.
*
* @param subject
* @param predicate
* @param object
*/
private void addTransient(Resource subject, URI predicate, Value object) {
org.openrdf.model.Statement st = new org.openrdf.model.impl.StatementImpl(
subject, predicate, object);
transients.add(st);
} // addTransient(Resource,URI,Value)
/**
* @return true if there are transient statements for the current
* transaction
*/
private boolean areThereTransients() {
return transients.size() != 0;
}
/**
* Removes the irrelevant transient statements. This to be called after the
* inference took place. Clears the transients member.
*
* @return dump of the added and filtered statements : to be used for the
* status reporter.
*/
private String removeIrrelevantTransients() {
org.openrdf.model.Statement st;
StringBuffer added = new StringBuffer();
StringBuffer filtered = new StringBuffer();
added.append("\nThe following statements were ADDed: \n");
filtered.append("\nThe following statements were FILTERed: \n");
boolean isAdded = false;
boolean isFiltered = false;
for (int ix = 0; ix < transients.size(); ix++) {
st = (org.openrdf.model.Statement) transients.get(ix);
Resource subject = st.getSubject();
URI predicate = st.getPredicate();
Value object = st.getObject();
if (!isStatementAccessible(subject, predicate, object, Right.ADD)) {
try {
if (baseRdfRepository.removeStatements(subject, predicate, object) > 0) {
filtered.append("\n").append(st.toString()).append("\n");
isFiltered = true;
}
}
catch (SailUpdateException e) {
throw new SailInternalException(
"Cannot finish the filtering of Tranisient Added Statements.\n"
+ "Caused by :" + e.getClass() + "\n"
+ e.getMessage());
}
}
else {
added.append("\n").append(st.toString()).append("\n");
isAdded = true;
}
} // for
transients = new ArrayList();
return (isAdded ? added.toString() : "\nNo Statements ADDed.\n")
+ (isFiltered ? filtered.toString() : "\nNo FILTERed Statements.\n");
} //removeIrrelevantTransients();
/* < Addition of Statements Handling */
/**
* Loads the Literals table.
*
* @param con connection to be used.
* @param st statement to be used.
* @throws SQLException if something goes wrong during the execution of this
* method.
*/
private void loadLiteralsTable(Connection con, java.sql.Statement st)
throws SQLException {
ResultSet result = st.executeQuery(SELECT + LITERALS_TABLE);
while (result.next()) {
int id = result.getInt("id");
String lang = result.getString("language");
String value = result.getString("label");
Literal literal = new LiteralImpl(value, lang);
literalById.put(new Integer(id), literal);
idByLiteral.put(literal, new Integer(id));
} // while
ThreadLog.trace(LITERALS_TABLE + " LOADED");
}
/**
* Loads the namespaces table.
*
* @param con connection to be used.
* @param st statement to be used.
* @throws SQLException if something goes wrong during the execution of this
* method.
*/
private void loadNameSpaceTable(Connection con, java.sql.Statement st)
throws SQLException {
ResultSet result = st.executeQuery(SELECT + NAMESPACES_TABLE);
while (result.next()) {
int id = result.getInt(1);
String prefix = result.getString(2);
String name = result.getString(3);
RdbmsNamespace ns = new RdbmsNamespace(id, prefix, name, false);
namespaces.put(new Integer(id), ns);
} // while
ThreadLog.trace(NAMESPACES_TABLE + " LOADED");
}
/**
* Loads the Resources table.
*
* @param con connection to be used.
* @param st statement to be used.
* @throws SQLException if something goes wrong during the execution of this
* method.
*/
private void loadResourcesTable(Connection con, java.sql.Statement st)
throws SQLException {
ResultSet result = st.executeQuery(SELECT + RESOURCES_TABLE);
ValueFactory factory = new ValueFactoryImpl();
while (result.next()) {
int id = result.getInt(1);
if (id > lastResId)
lastResId = id;
int namespace = result.getInt(2);
String name = result.getString(3);
RdbmsNamespace ns = (RdbmsNamespace) namespaces.get(new Integer(
namespace));
if (null == ns)
throw new SailInternalException(
"[Unknown] resource [namespace]. Namespace [id]="
+ namespace);
Resource rs = null;
if (namespace != 0)
rs = new URIImpl(ns.getName(), name);
else
// FIXME this is probably not correct, since it does not take
// the bNode ID into account!
rs = factory.createBNode();
resById.put(new Integer(id), rs);
idByRes.put(rs, new Integer(id));
if (id > lastResourceId) {
lastResourceId = id;
}
} // while
ThreadLog.trace(RESTRICTIONS_TABLE + " LOADED");
}
/** Loads Security Setup from database */
private void loadSecuritySetup() {
ThreadLog.trace(">>>Loading of Security Setup");
Connection con = null;
java.sql.Statement st = null;
try {
con = conPool.getConnection();
st = con.createStatement();
loadNameSpaceTable(con, st);
loadResourcesTable(con, st);
loadLiteralsTable(con, st);
loadRestrictionsTable(con, st);
loadSecurityRulesTable(con, st);
loadRolesTable(con, st);
loadRolesHierarchyTable(con, st);
loadRolesRulesTable(con, st);
loadResourceOrPropertyRestrictionsTable(con, st);
loadQueryRestrictionsTable(con, st);
loadPatternRestrictionsTable(con, st);
loadUsersRolesTable(con, st);
loadUsersRulesTable(con, st);
}
catch (SQLException sqle) {
throw new SailInternalException(sqle);
}
finally {
try {
st.close();
con.close();
}
catch (SQLException e) {
throw new SailInternalException(e);
}
} // finally
ThreadLog.trace("<<>>initializing Database");
Connection con = null;
java.sql.Statement st = null;
try {
con = conPool.getConnection();
st = con.createStatement();
createSecurityTables(con, st);
ThreadLog.trace("<<
resPerson = new URIImpl(PERSON);
ResourceRestriction rsPersons = (ResourceRestriction) Restriction.createClassesRestriction("Persons", "Persons");
{
Set rSet = rsPersons.getResources();
rSet.add(resPerson);
rsPersons.setResources(rSet);
}
resHasPosition = new URIImpl(HAS_POSITION);
PropertiesRestriction rsHasPosition = (PropertiesRestriction) Restriction.createPropertiesRestriction("hasPosition", "hasPosition");
{
Set rSet = rsHasPosition.getProperties();
rSet.add(resHasPosition);
rsHasPosition.setProperties(rSet);
}
PatternRestriction rsPersonHasPosition = (PatternRestriction) ResourceRestriction.createPatternRestriction("PersonHasPosition", "PersonHasPosition");
rsPersonHasPosition.addSubjectRestriction(rsPersons);
rsPersonHasPosition.addPredicateRestriction(rsHasPosition);
Rule ruPersonHasPosition = new RuleImpl(
"PersonHasPositionRead", "PersonHasPositionRead");
ruPersonHasPosition.setRestriction(rsPersonHasPosition);
ruPersonHasPosition.setReadRight(true);
//< scSkill, pSubClassOf, _ >
resSubClassOf = new URIImpl(SUB_CLASS_OF);
PropertiesRestriction rsSubClassOf = (PropertiesRestriction) Restriction.createPropertiesRestriction("subClassOf", "subClassOf");
{
Set props = rsSubClassOf.getProperties();
props.add(resSubClassOf);
rsSubClassOf.setProperties(props);
}
PatternRestriction rsSkillSubClassOf = (PatternRestriction) Restriction.createPatternRestriction("SkillSubClassOf", "rsSkillSubClassOf");
{
rsSkillSubClassOf.addSubjectRestriction(rsSkillsSchema);
rsSkillSubClassOf.addPredicateRestriction(rsSubClassOf);
}
Rule ruSkillSubClassOf = new RuleImpl("SkillSubClassOfRead",
"SkillSubClassOfRead");
ruSkillSubClassOf.setRestriction(rsSkillSubClassOf);
ruSkillSubClassOf.setReadRight(true);
//< iPerson, pHasSkill, iTechnicalSkill >
resHasSkill = new URIImpl(HAS_SKILL);
PropertiesRestriction rsHasSkill = (PropertiesRestriction) Restriction.createPropertiesRestriction("HasSkill", "HasSkill");
{
Set props = rsHasSkill.getProperties();
props.add(resHasSkill);
rsHasSkill.setProperties(props);
}
PatternRestriction rsPersonHasTechSkill = (PatternRestriction) Restriction.createPatternRestriction("PersonHasTechSkill", "PersonHasTechSkill");
{
rsPersonHasTechSkill.addSubjectRestriction(rsPersons);
rsPersonHasTechSkill.addPredicateRestriction(rsHasSkill);
rsPersonHasTechSkill.addObjectRestriction(rsTSkills);
}
Rule ruPersonHasTechSkill = new RuleImpl(
"PersonHasTechSkillRead", "PersonHasTechSkillRead");
ruPersonHasTechSkill.setRestriction(rsPersonHasTechSkill);
ruPersonHasTechSkill.setReadRight(true);
Set rulz = uEmp.getRules();
rulz.add(ruPersonHasPosition);
rulz.add(ruSkillSubClassOf);
rulz.add(ruPersonHasTechSkill);
uEmp.setRules(rulz);
}
//QueryTester
{
QueryRestriction rsQuSchema = (QueryRestriction) Restriction.createQueryRestriction("QuSchema", "QuSchema");
rsQuSchema.addQuery(HAS_POSITION_QUERY);
Rule ruQuReadSchema = new RuleImpl("QuReadSchema",
"QuReadSchema");
ruQuReadSchema.setRestriction(rsQuSchema);
ruQuReadSchema.setReadRight(true);
Set rulz = uQue.getRules();
rulz.add(ruQuReadSchema);
uQue.setRules(rulz);
}
//Dimitar
URI resMitac = new URIImpl(MITAC);
{
InstancesRestriction rsMitac = (InstancesRestriction) Restriction.createInstancesRestriction("Mitac", "Mitac");
Set resz = rsMitac.getResources();
resz.add(resMitac);
rsMitac.setResources(resz);
Rule ruMitac = new RuleImpl("MitacRead", "MitacRead");
ruMitac.setReadRight(true);
ruMitac.setRestriction(rsMitac);
Set rulz = uMitac.getRules();
rulz.add(ruMitac);
uMitac.setRules(rulz);
}
//check all resources and store them if they do not exist
{
ArrayList rez = new ArrayList();
rez.add(resHasPosition);
rez.add(resHasSkill);
rez.add(resPerson);
rez.add(resSkill);
rez.add(resSubClassOf);
rez.add(resTechSkill);
rez.add(resMitac);
storeMissingResources(rez);
loadNameSpaceTable(con, st);
loadResourcesTable(con, st);
}
}
catch (SQLException sqle) {
throw new SailInternalException(sqle);
}
catch (Exception npe) {
throw new SailInternalException(npe);
}
} // upload sample setup
/** Uploads sample security setup data via direct sql queries */
private void uploadSampleSetupDirectSQL() {
Connection con = null;
java.sql.Statement st = null;
try {
con = conPool.getConnection();
st = con.createStatement();
dropSecurityTables(con, st);
createSecurityTables(con, st);
Map resIdsByUri = new HashMap();
/* load resources */
ResultSet rset = st.executeQuery(" select r.id, concat(n.name, r.localname) "
+ " from namespaces n, resources r "
+ " where n.id = r.namespace ");
while (rset.next()) {
Integer id = new Integer(rset.getInt(1));
String uri = rset.getString(2);
resIdsByUri.put(uri, id);
}
/** uses these through out the uploading as last ids */
int restrId = 0;
int ruleId = 0;
int schemaRestrId;
int reposRestrId;
int readSchemaRuleId;
int doEverythingRuleId;
/** schema read for all users */
/* SCHEMA RESTR */
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "( "
+ (schemaRestrId = ++restrId) + "," + Restriction.SCHEMA + ","
+ "'Schema'" + "," + "'Schema Restriction'" + " );");
/* REPOSITORY RESTR */
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "( "
+ (reposRestrId = ++restrId) + "," + Restriction.REPOSITORY
+ "," + "'Repository'" + "," + "'Repository Restriction'"
+ " );");
/* READ SCHEMA RULE */
st.executeUpdate(INSERT + SECURITY_RULES_TABLE + VALUES + "( "
+ (readSchemaRuleId = ++ruleId) + "," + "'ReadSchema'" + ","
+ "'Allows reading of the Schema of the repository.'" + ","
+ schemaRestrId + ",1, 0,0,0,0);");
/* DO EVERYTHING WITH THE REPOSITROY RULE */
st.executeUpdate(INSERT + SECURITY_RULES_TABLE + VALUES + "( "
+ (doEverythingRuleId = ++ruleId) + "," + "'DoEverything'"
+ "," + "'Allows all actions over the whole repository'" + ","
+ reposRestrId + ",1,1,1,1,1);");
/* READ the REPOSITROY RULE */
int readRepositoryRuleId = 0;
st.executeUpdate(INSERT + SECURITY_RULES_TABLE + VALUES + "( "
+ (readRepositoryRuleId = ++ruleId) + "," + "'ReadRepository'"
+ "," + "'Allows readind over the whole repository'" + ","
+ reposRestrId + ",1,0,0,0,0);");
/* ASSIGN DOEVERYTHING RULE TO ADMIN */
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 1
+ "," + doEverythingRuleId + ");");
/* ASSIGN READ SCHMEA RULE TO TESTUSER */
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 3
+ "," + readSchemaRuleId + ");");
/* read everything from the repository for HRManager and RandD */
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 4
+ "," + readRepositoryRuleId + ");");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 5
+ "," + readRepositoryRuleId + ");");
/*---HRManager Rules: allowed: editing of skills hier-----*/
boolean skipSkillsRules = false;
int skillId = 0;
int skillRestrictionId = 0;
int skillLevelId = 0;
try {
skillId = ((Integer) resIdsByUri.get(SKILL)).intValue();
skillLevelId = ((Integer) resIdsByUri.get(SKILL_LEVEL)).intValue();
}
catch (NullPointerException npe) {
skipSkillsRules = true;
}
if (!skipSkillsRules) {
int hRCOSRestrId = 0;
int hRSkills1Rule = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (hRCOSRestrId = ++restrId) + ","
+ Restriction.CLASSES_OVER_SCHEMA + ","
+ "'SkillsHierarchy','Skills Hierarchy');");
// not so local
skillRestrictionId = hRCOSRestrId;
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (hRSkills1Rule = ++ruleId)
+ ","
+ "'EditSkillsHierarchy','Allows Editing of the Skill Hierarchy'"
+ "," + hRCOSRestrId + ",1,1,1,0,0);");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hRCOSRestrId + "," + skillId + ");");
int hRClassRestrId = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (hRClassRestrId = ++restrId) + "," + Restriction.CLASSES
+ "," + "'SkillsInstances','Skills Instances');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hRClassRestrId + "," + skillId + ");");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hRClassRestrId + "," + skillLevelId + ");");
int hRSkills1Rule2 = 0;
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (hRSkills1Rule2 = ++ruleId)
+ ","
+ "'EditSkillsInstances','Allows Editing of the Skill Instances'"
+ "," + hRClassRestrId + ",1,1,1,0,0);");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 4
+ "," + hRSkills1Rule + ");");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 4
+ "," + hRSkills1Rule2 + ");");
} // do not skipSkillsRules
skipSkillsRules = false;
/* RandDManager : allowed :editing of TechSkills */
skillId = 0;
try {
skillId = ((Integer) resIdsByUri.get(TECH_SKILL)).intValue();
skillLevelId = ((Integer) resIdsByUri.get(SKILL_LEVEL)).intValue();
}
catch (NullPointerException npe) {
skipSkillsRules = true;
}
if (!skipSkillsRules) {
int hrCOSRestrId = 0;
int hRSkills1Rule = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (hrCOSRestrId = ++restrId) + ","
+ Restriction.CLASSES_OVER_SCHEMA + ","
+ "'TechSkillsHierarchy','Technical Skills Hierarchy');");
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (hRSkills1Rule = ++ruleId)
+ ","
+ "'EditTechSkillsHierarchy','Allows Editing of the Tech Skill Hierarchy'"
+ "," + hrCOSRestrId + ",1,1,1,0,0);");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hrCOSRestrId + "," + skillId + ");");
int hRClassRestrId = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (hRClassRestrId = ++restrId) + "," + Restriction.CLASSES
+ ","
+ "'TechSkillsInstances','Technical Skills Instances');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hRClassRestrId + "," + skillId + ");");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hRClassRestrId + "," + skillLevelId + ");");
int hRSkills1Rule2 = 0;
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (hRSkills1Rule2 = ++ruleId)
+ ","
+ "'EditSkillsInstances','Allows Editing of the Skill Instances'"
+ "," + hRClassRestrId + ",1,1,1,0,0);");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 5
+ "," + hRSkills1Rule + ");");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 5
+ "," + hRSkills1Rule2 + ");");
} // do not skipSkillsRules
int techSkillId = 0;
skillId = 0;
int personId = 0;
int subClassOfId = 0;
int hasPositionId = 0;
int hasSkillId = 0;
boolean skip = false;
try {
skillId = ((Integer) resIdsByUri.get(SKILL)).intValue();
techSkillId = ((Integer) resIdsByUri.get(TECH_SKILL)).intValue();
personId = ((Integer) resIdsByUri.get(PERSON)).intValue();
subClassOfId = ((Integer) resIdsByUri.get(SUB_CLASS_OF)).intValue();
hasSkillId = ((Integer) resIdsByUri.get(HAS_SKILL)).intValue();
hasPositionId = ((Integer) resIdsByUri.get(HAS_POSITION)).intValue();
}
catch (NullPointerException npe) {
skip = true;
}
if (!skip) {
int personRstr = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (personRstr = ++restrId) + "," + Restriction.CLASSES
+ "," + "'Persons','Persons Restriction');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ personRstr + "," + personId + ");");
int hasPositionRestr = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (hasPositionRestr = ++restrId) + ","
+ Restriction.PROPERTIES + ","
+ "'hasPosition','hasPosition Restriction');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hasPositionRestr + "," + hasPositionId + ");");
int subClassRestr = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (subClassRestr = ++restrId) + ","
+ Restriction.PROPERTIES + ","
+ "'SubClassOf','SubClassOf Restriction');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ subClassRestr + "," + subClassOfId + ");");
int hasSkillRestr = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (hasSkillRestr = ++restrId) + ","
+ Restriction.PROPERTIES + ","
+ "'hasSkill','hasSkill Restriction');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ hasSkillRestr + "," + hasSkillId + ");");
int techSkillsRestr = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (techSkillsRestr = ++restrId) + "," + Restriction.CLASSES
+ "," + "'TechSkillInstances','TechSkillInstances');");
st.execute(INSERT + RES_PROP_RESTRS_TABLE + VALUES + "("
+ techSkillsRestr + "," + techSkillId + ");");
// pattern restrictions
int ptrnPersonPositionsId = 0;
st.executeUpdate(INSERT + RESTRICTIONS_TABLE + VALUES + "("
+ (ptrnPersonPositionsId = ++restrId) + ","
+ Restriction.PATTERN + ","
+ "'Person Positions','Person Positions Restriction');");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnPersonPositionsId + "," + personRstr + ","
+ PatternRestriction.SPO_SUBJECT + ");");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnPersonPositionsId + "," + hasPositionRestr + ","
+ PatternRestriction.SPO_PREDICATE + ");");
int personPositionsRule = 0;
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (personPositionsRule = ++ruleId)
+ ","
+ "'Read Person Positions Rule','Read Person Positions Rule'"
+ "," + ptrnPersonPositionsId + ",1,0,0,0,0);");
int ptrnSkillsHierarchyRstr = 0;
st.executeUpdate(INSERT
+ RESTRICTIONS_TABLE
+ VALUES
+ "("
+ (ptrnSkillsHierarchyRstr = ++restrId)
+ ","
+ Restriction.PATTERN
+ ","
+ "'Skills Hierarchy Pattern','Skills Hierarchy Pattern Restriction');");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnSkillsHierarchyRstr + "," + skillRestrictionId + ","
+ PatternRestriction.SPO_SUBJECT + ");");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnSkillsHierarchyRstr + "," + subClassRestr + ","
+ PatternRestriction.SPO_PREDICATE + ");");
int readSkillsHierarchyRule = 0;
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (readSkillsHierarchyRule = ++ruleId)
+ ","
+ "'Read Skills Hierarchy Rule','Read Skills Hierarchy Rule'"
+ "," + ptrnSkillsHierarchyRstr + ",1,0,0,0,0);");
int ptrnTechSkillInstancesRstr = 0;
st.executeUpdate(INSERT
+ RESTRICTIONS_TABLE
+ VALUES
+ "("
+ (ptrnTechSkillInstancesRstr = ++restrId)
+ ","
+ Restriction.PATTERN
+ ","
+ "'Technical Skill Instances Pattern','Technical Skill Instances Pattern Restriction');");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnTechSkillInstancesRstr + "," + personRstr + ","
+ PatternRestriction.SPO_SUBJECT + ");");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnTechSkillInstancesRstr + "," + hasSkillRestr + ","
+ PatternRestriction.SPO_PREDICATE + ");");
st.executeUpdate(INSERT + PATTERN_RESTRS_TABLE + VALUES + "("
+ ptrnTechSkillInstancesRstr + "," + techSkillsRestr + ","
+ PatternRestriction.SPO_OBJECT + ");");
int readTechSkillsRule = 0;
st.executeUpdate(INSERT
+ SECURITY_RULES_TABLE
+ VALUES
+ "("
+ (readTechSkillsRule = ++ruleId)
+ ","
+ "'Read Tech Skills Hierarchy Rule','Read Skills Hierarchy Rule'"
+ "," + ptrnTechSkillInstancesRstr + ",1,0,0,0,0);");
//assign rules to employee
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 6
+ "," + personPositionsRule + ");");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 6
+ "," + readSkillsHierarchyRule + ");");
st.executeUpdate(INSERT + USERS_RULES_TABLE + VALUES + "(" + 6
+ "," + readTechSkillsRule + ");");
} // employee rules
ThreadLog.trace("Sample Security Setup UPLOADED.");
}
catch (SQLException sqle) {
throw new SailInternalException(sqle);
}
catch (Exception npe) {
throw new SailInternalException(npe);
} //catch
finally {
try {
st.close();
con.close();
}
catch (SQLException e) {
throw new SailInternalException(e);
}
} // finally
} // UploadSampleSecuritySetup()
/**
* Stores the security setup cia update sql queries to the repository.
*/
private void storeSecuritySetup() {
Connection con = null;
java.sql.Statement st = null;
try {
ThreadLog.trace("Store Security Setup ...");
con = conPool.getConnection();
st = con.createStatement();
/* load resources */
ResultSet rset = st.executeQuery(" select r.id, concat(n.name, r.localname) "
+ " from namespaces n, resources r "
+ " where n.id = r.namespace ");
while (rset.next()) {
Integer id = new Integer(rset.getInt(1));
String uri = rset.getString(2);
resIdsByUri.put(uri, id);
}
dropSecurityTables(con, st);
createSecurityTables(con, st);
ArrayList userz = new ArrayList(users);
Set queSet = new HashSet();
ArrayList queList = new ArrayList();
ArrayList rolez = new ArrayList(roles);
Set rulez = new HashSet(RoleImpl.getRules(roles, false));
for (int ui = 0; ui < userz.size(); ui++) {
User usr = (User) userz.get(ui);
queSet.addAll(usr.toSql());
rulez.addAll(usr.getRules());
} // for userz
ArrayList rolz = new ArrayList(rolez);
for (int roi = 0; roi < rolz.size(); roi++) {
Role role = (Role) rolz.get(roi);
rulez.addAll(role.getRules(true));
queSet.addAll(role.toSql());
} // for rolz
ArrayList rulz = new ArrayList(rulez);
for (int rui = 0; rui < rulz.size(); rui++) {
Rule rule = (Rule) rulz.get(rui);
queSet.addAll(rule.toSql());
} // for rulz
ArrayList restrz = new ArrayList(Restriction.getRestrictions());
for (int ri = 0; ri < restrz.size(); ri++) {
Restriction r = (Restriction) restrz.get(ri);
queSet.addAll(r.toSql(idByLiteral, idByRes));
} // for restrictions
// execute queries
queList = new ArrayList(queSet);
for (int qi = 0; qi < queList.size(); qi++) {
String q = queList.get(qi).toString();
int result;
try {
result = st.executeUpdate(q);
}
catch (Exception x) {
result = st.executeUpdate(q);
}
if (result <= 0)
throw new SecurityException(
"The following query did not change the repository :\n"
+ q);
} // for queries
ThreadLog.trace("Security Setup STORED.");
}
catch (SQLException sqle) {
throw new SailInternalException(sqle);
}
catch (SecurityException se) {
throw new SailInternalException(se);
}
catch (Exception npe) {
throw new SailInternalException(npe);
}
finally {
try {
st.close();
con.close();
}
catch (SQLException e) {
throw new SailInternalException(e);
}
} // finally
} // storeSecuritySetup()
/** stores the missing resources from the security setup */
private void storeMissingResources(ArrayList resourcez) {
try {
baseRdfRepository.startTransaction();
URI resType = new URIImpl(RDF_TYPE);
URI resResource = new URIImpl(RESOURCE);
for (int i = 0; i < resourcez.size(); i++) {
Resource res = (Resource) resourcez.get(i);
if (idByRes.get(res) == null) {
baseRdfRepository.addStatement(res, resType, resResource);
}
}
baseRdfRepository.commitTransaction();
}
catch (SailUpdateException e) {
throw new SailInternalException(e);
}
}
/**
* Answers the question : is this resource accessible through this query restriction.
* @param rst
* @param res
* @return
* @todo : make it private
* @todo implement it to work both for literals(instances restr) and resources
*/
boolean isQuAccessible(QueryRestriction rst, Resource res) {
boolean is = false;
String query;
ArrayList qus = new ArrayList(rst.getQueries());
for (int i = 0; i < qus.size(); i++) {
query = qus.get(i).toString();
queryResult = (ArrayList) queryResults.get(query);
if (queryResult == null) {
// execute query
try {
org.openrdf.sesame.query.rql.RqlEngine queryEngine = new org.openrdf.sesame.query.rql.RqlEngine(
baseRdfSchemaSource);
org.openrdf.sesame.query.rql.model.Query queryModel = null;
queryModel = queryEngine.parseQuery(query);
queryEngine.evaluateQuery(queryModel, this);
}
catch (MalformedQueryException me) {
// Incorrect query
throw new SailInternalException(me);
}
catch (org.openrdf.sesame.query.QueryEvaluationException qee) {
// Error during evaluation
throw new SailInternalException(qee);
}
catch (IOException ioe) {
throw new SailInternalException(ioe);
}
while (!isQueryReady) {
int k = 0;
}
// for (int j = 0 ; j < queryResult.size() ; j++){
// System.out.println(queryResult.get(j).toString());
// }
queryResults.put(query, queryResult);
} // if null query result
for (int ri = 0; ri < queryResult.size(); ri++) {
Value v = (Value) queryResult.get(ri);
URI rr;
Literal lit;
if (v instanceof URI) {
rr = (URI) v;
// FIXME cast to URI appropriate?
if (((URI) res).getLocalName().equals(rr.getLocalName())
&& ((URI) res).getNamespace().equals(rr.getNamespace())) {
is = true;
break;
}
}
}
if (is)
break;
}
return is;
}
/* (non-Javadoc)
* @see org.openrdf.sesame.sail.RdfRepository#addListener(org.openrdf.sesame.sail.SailChangedListener)
*/
public void addListener(SailChangedListener listener) {
baseRdfRepository.addListener(listener);
}
/* (non-Javadoc)
* @see org.openrdf.sesame.sail.RdfRepository#removeListener(org.openrdf.sesame.sail.SailChangedListener)
*/
public void removeListener(SailChangedListener listener) {
baseRdfRepository.removeListener(listener);
}
/** main method for test purposes */
/*
* public static void main(String[] args) {
* // sesame startup initialization via a config file and security setup
* test //------------------------------ try {
* SesameStartup.initialize("system.conf");
* RequestRouter.login("Dimitar","mitac"); Sail sail =
* SystemConfigCenter.getSail("mysql-omm","Dimitar","mitac",true,false);
* SecurityServices security = (SecuritySail) QuerySailStack
* .queryInterface(sail,"org.openrdf.sesame.omm.SecurityServices");
*
* System.out.println(SessionContext.getContext().userID);
* System.out.println("Statements"); StatementIterator sti
* =((RdfSchemaRepository)security).getStatements(null,null,null); while
* (sti.hasNext()) { System.out.println(sti.next()); }
*
* } catch (Exception x) { x.printStackTrace(); }
*
*
*
* // ---------------------- // initialize sails and test them
* // VersioningTmsMySQLSail verSail= new VersioningTmsMySQLSail(); // //
* SecuritySail securitySail = new SecuritySail(); //
* //securitySail.setBaseSail(verSail); // // HashMap map = new HashMap(); //
* map.put("jdbcDriver", "org.gjt.mm.mysql.Driver"); // map.put("jdbcUrl",
* "jdbc:mysql://localhost:3306/ommdemo"); // map.put("user", "sesame"); //
* map.put("password", "sesame"); // map.put("security_setup",
* "http://www.ontotext.com/otk/2002/05/SecurityDemo/DemoSecuritySetup.rdf"); // //
* try { // SessionContext context = new SessionContext(); // context.user =
* "admin"; // context.pass = "admin"; // context.userID=1; //
* context.baseUrl =
* "http://www.ontotext.com/otk/2002/05/SecurityDemo/newSwingSkill.rdf"; //
* context.repository = "mysql-omm"; // SessionContext.put("",context); //
* SessionContext.setContext(context); // // verSail.initialize(map); // // }
* catch (Exception e) { // e.printStackTrace(System.out); //
* System.out.println(e.getMessage()); // } // verSail.shutDown(); }
*/
} // class SecuritySail
© 2015 - 2025 Weber Informatics LLC | Privacy Policy