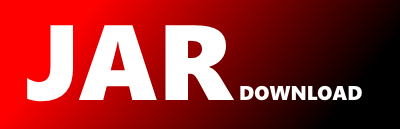
org.openrdf.sesame.sailimpl.rdbms.RdbmsInferenceServices Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2003 OntoText Lab, Sirma AI OOD
*
* Contact:
* Sirma AI OOD, OntoText Lab.
* 38A, Christo Botev Blvd.
* 1000 Sofia, Bulgaria
* tel. +359(2)981 00 18
* fax. +359(2)981 90 58
* [email protected]
*
* http://www.ontotext.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.sailimpl.rdbms;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Map;
/**
* Title: Custom Inference Rules
* Description:
* Copyright: Copyright (c) 2003
* Company: Ontotext Lab. Sirma AI
* @author Damyan Ognyanoff
* @version 1.0
*/
public class RdbmsInferenceServices implements InferenceServices {
/*----------------+
| Variables |
+----------------*/
RdfSchemaRepository _sail = null;
RdfMTDependencyInferencer _depInferencer = null;
RdfMTInferencer _rdfMTInferencer = null;
boolean _useDepInferencer;
/*----------------+
| Constructors |
+----------------*/
public RdbmsInferenceServices() {
_useDepInferencer = true;
}
public RdbmsInferenceServices(boolean useDepInferencer) {
_useDepInferencer = useDepInferencer;
}
/*----------------+
| Methods |
+----------------*/
public void setDependencyInferencer(boolean useDependencyInferencer) {
_useDepInferencer = useDependencyInferencer;
}
public void initialize(RdfSchemaRepository sail, Map configParams) {
_sail = sail;
}
public void initRdfSchema() {
// @todo: initialize
if (_useDepInferencer) {
_depInferencer = new RdfMTDependencyInferencer(_sail, _sail._rdbms);
}
_rdfMTInferencer = new RdfMTInferencer(_sail, _sail._rdbms);
_rdfMTInferencer.initialize();
}
public void doInferencing() {
_rdfMTInferencer.doInferencing();
}
public void removeExpiredStatements()
throws SQLException
{
if (_useDepInferencer) {
_makeExpiredStatementsInferred();
_determineGroundedStatements();
// Get the IDs of statements that are no longer grounded.
Connection con = _sail._rdbms.getConnection();
java.sql.Statement st = con.createStatement();
ResultSet rs = st.executeQuery(
" SELECT t.id FROM " + TRIPLES_TABLE + " t" +
" LEFT JOIN " + GROUNDED_TRIPLES_TABLE + " g" +
" ON t.id = g.id" +
" WHERE g.id IS NULL");
String[] idChunks = _sail._chunkIdSet(rs, 3500);
rs.close();
st.close();
// Delete these statements from TRIPLES_TABLE and DEPEND_TABLE.
con.setAutoCommit(false);
st = con.createStatement();
for (int i = 0; i < idChunks.length; i++) {
st.executeUpdate(
"DELETE FROM " + TRIPLES_TABLE +
" WHERE id IN " + idChunks[i]);
st.executeUpdate(
"DELETE FROM " + DEPEND_TABLE +
" WHERE id IN " + idChunks[i]);
st.executeUpdate(
"DELETE FROM " + DEPEND_TABLE +
" WHERE dep1 IN " + idChunks[i]);
st.executeUpdate(
"DELETE FROM " + DEPEND_TABLE +
" WHERE dep2 IN " + idChunks[i]);
_sail._processChunkFromRemoveExpiredStatements(idChunks[i]);
}
con.commit();
st.close();
con.close();
_sail._rdbms.clearTable(GROUNDED_TRIPLES_TABLE);
_sail._rdbms.clearTable(NEW_GROUNDED_TRIPLES_TABLE);
}
else { // don't use dependency inferencer
// mark all expired statements as inferred
_makeExpiredStatementsInferred();
// remove all inferred statements
_removeAllInferred();
// copy all TRIPLES to the NEW_TRIPLES table
_sail._rdbms.copyRows(TRIPLES_TABLE, NEW_TRIPLES_TABLE);
// clean the TRIPLES table.
_sail._rdbms.clearTable(TRIPLES_TABLE);
// re-compute the closure
_rdfMTInferencer.initialize();
}
}
public void processNewStatements()
throws SQLException
{
if (_useDepInferencer) {
_depInferencer.processNewStatements();
}
}
public void markAxioms()
throws SQLException
{
if (_useDepInferencer) {
_depInferencer.markAxioms();
}
}
/**
* Set the 'explicit' flag to 'false' for all statements whose ID
* is in the EXPIRED_TRIPLES_TABLE.
**/
protected void _makeExpiredStatementsInferred()
throws SQLException
{
Connection con = _sail._rdbms.getConnection();
java.sql.Statement st = con.createStatement();
ResultSet rs = st.executeQuery(
"SELECT DISTINCT id FROM " + EXPIRED_TRIPLES_TABLE);
String[] idChunks = _sail._chunkIdSet(rs, 3500);
rs.close();
st.close();
// Mark all expired statements to be inferred. The statements
// cannot just be removed, as it is possible that they can also
// be inferred from other statements.
con.setAutoCommit(false);
st = con.createStatement();
for (int i = 0; i < idChunks.length; i++) {
st.executeUpdate(
"UPDATE " + TRIPLES_TABLE +
" SET explicit = " + _sail._rdbms.FALSE +
" WHERE id IN " + idChunks[i]);
}
con.commit();
st.close();
con.close();
}
private void _removeAllInferred()
throws SQLException
{
_sail._rdbms.executeUpdate(
"DELETE FROM " + TRIPLES_TABLE +
" WHERE explicit = " + _sail._rdbms.FALSE);
}
protected void _determineGroundedStatements()
throws SQLException
{
// Statement with ID '0' is grounded. '0' is used in dependencies
// for axioms (two '0' values), and statements that are dependent
// of only one statement (dep2 is '0').
_sail._rdbms.executeUpdate("INSERT INTO " + GROUNDED_TRIPLES_TABLE + " VALUES(0)");
// All explicit statements are grounded:
_sail._rdbms.executeUpdate(
"INSERT INTO " + GROUNDED_TRIPLES_TABLE +
" SELECT id FROM " + TRIPLES_TABLE +
" WHERE explicit = " + _sail._rdbms.TRUE);
while (true) {
int count = _sail._rdbms.executeUpdate(
"INSERT INTO " + NEW_GROUNDED_TRIPLES_TABLE +
" SELECT DISTINCT d.id" +
" FROM " + DEPEND_TABLE + " d" +
" LEFT JOIN " + GROUNDED_TRIPLES_TABLE + " g1 ON d.dep1 = g1.id" +
" LEFT JOIN " + GROUNDED_TRIPLES_TABLE + " g2 ON d.dep2 = g2.id" +
" LEFT JOIN " + GROUNDED_TRIPLES_TABLE + " g3 ON d.id = g3.id" +
" WHERE g1.id IS NOT NULL" +
" AND g2.id IS NOT NULL" +
" AND g3.id IS NULL");
if (count == 0) {
// No more grounded statements found
break;
}
_sail._rdbms.copyRows(NEW_GROUNDED_TRIPLES_TABLE, GROUNDED_TRIPLES_TABLE);
_sail._rdbms.clearTable(NEW_GROUNDED_TRIPLES_TABLE);
_sail._rdbms.optimizeTable(GROUNDED_TRIPLES_TABLE);
}
}
public void createDependenciesTable()
throws SQLException
{
_sail._rdbms.executeUpdate(
"CREATE TABLE " + DEPEND_TABLE + " (" +
"id " + _sail._rdbms.ID_INT + " NOT NULL, " +
"dep1 " + _sail._rdbms.ID_INT + " NOT NULL, " +
"dep2 " + _sail._rdbms.ID_INT + " NOT NULL)");
_sail._rdbms.createIndex(DEPEND_TABLE, new String[] {"id", "dep1", "dep2"}, false);
_sail._rdbms.createIndex(DEPEND_TABLE, new String[] {"dep1", "dep2"}, false);
}
public void afterInitialize() {
// no-op
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy