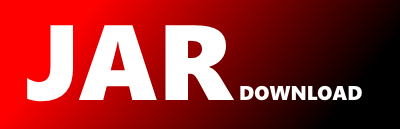
org.openrdf.sesame.sailimpl.sync.SyncRdfSchemaRepository Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.sailimpl.sync;
import org.openrdf.model.Resource;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import org.openrdf.sesame.sail.LiteralIterator;
import org.openrdf.sesame.sail.RdfSchemaRepository;
import org.openrdf.sesame.sail.Sail;
import org.openrdf.sesame.sail.SailInternalException;
import org.openrdf.sesame.sail.StackedSail;
import org.openrdf.sesame.sail.StatementIterator;
/**
* @author Arjohn Kampman
* @version $Revision: 1.4.4.2 $
*/
public class SyncRdfSchemaRepository
extends SyncRdfRepository
implements RdfSchemaRepository, StackedSail
{
private RdfSchemaRepository _rdfSchemaRepository;
public SyncRdfSchemaRepository() {
super();
}
public void setBaseSail(Sail sail) {
if (sail instanceof RdfSchemaRepository) {
super.setBaseSail(sail);
_rdfSchemaRepository = (RdfSchemaRepository)sail;
}
else {
throw new SailInternalException("base Sail should be an RdfSchemaRepository");
}
}
public StatementIterator getExplicitStatements(
Resource subj, URI pred, Value obj)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getExplicitStatements(subj, pred, obj);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean hasExplicitStatement(Resource subj, URI pred, Value obj)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.hasExplicitStatement(subj, pred, obj);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getClasses() {
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getClasses();
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isClass(Resource resource) {
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isClass(resource);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getProperties() {
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getProperties();
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isProperty(Resource resource) {
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isProperty(resource);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getSubClassOf(
Resource subClass, Resource superClass)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getSubClassOf(
subClass, superClass);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getDirectSubClassOf(
Resource subClass, Resource superClass)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getDirectSubClassOf(
subClass, superClass);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isSubClassOf(
Resource subClass, Resource superClass)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isSubClassOf(subClass, superClass);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isDirectSubClassOf(
Resource subClass, Resource superClass)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isDirectSubClassOf(
subClass, superClass);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getSubPropertyOf(
Resource subProperty, Resource superProperty)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getSubPropertyOf(
subProperty, superProperty);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getDirectSubPropertyOf(
Resource subProperty, Resource superProperty)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getDirectSubPropertyOf(
subProperty, superProperty);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isSubPropertyOf(
Resource subProperty, Resource superProperty)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isSubPropertyOf(
subProperty, superProperty);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isDirectSubPropertyOf(
Resource subProperty, Resource superProperty)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isDirectSubPropertyOf(
subProperty, superProperty);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getDomain(Resource prop, Resource domain) {
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getDomain(prop, domain);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getRange(Resource prop, Resource range) {
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getRange(prop, range);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getType(
Resource anInstance, Resource aClass)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getType(anInstance, aClass);
}
finally {
_releaseReadLock();
}
return result;
}
public StatementIterator getDirectType(
Resource anInstance, Resource aClass)
{
StatementIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getDirectType(anInstance, aClass);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isType(
Resource anInstance, Resource aClass)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isType(anInstance, aClass);
}
finally {
_releaseReadLock();
}
return result;
}
public boolean isDirectType(
Resource anInstance, Resource aClass)
{
boolean result = false;
_getReadLock();
try {
result = _rdfSchemaRepository.isDirectType(anInstance, aClass);
}
finally {
_releaseReadLock();
}
return result;
}
public LiteralIterator getLiterals(
String label, String language, URI datatype)
{
LiteralIterator result = null;
_getReadLock();
try {
result = _rdfSchemaRepository.getLiterals(label, language, datatype);
}
finally {
_releaseReadLock();
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy