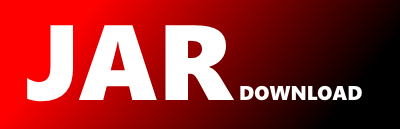
org.openrdf.sesame.server.http.ClearRepositoryServlet Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.sesame.server.http;
import java.io.IOException;
import java.util.Map;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.openrdf.util.http.HttpServerUtil;
import org.openrdf.util.log.ThreadLog;
import org.openrdf.sesame.admin.AdminListener;
import org.openrdf.sesame.admin.HtmlAdminMsgWriter;
import org.openrdf.sesame.admin.XmlAdminMsgWriter;
import org.openrdf.sesame.config.AccessDeniedException;
import org.openrdf.sesame.config.UnknownRepositoryException;
import org.openrdf.sesame.constants.AdminResultFormat;
import org.openrdf.sesame.repository.SesameRepository;
import org.openrdf.sesame.repository.local.LocalService;
import org.openrdf.sesame.repository.remote.HTTPErrorType;
import org.openrdf.sesame.server.SesameServer;
public class ClearRepositoryServlet extends SesameServlet {
/*------------------------------------------+
| Methods |
+------------------------------------------*/
// Implements SesameServlet._doPost(...)
protected void _doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
if (HttpServerUtil.isMultipartFormRequest(request)) {
_handleMultipartFormRequest(request, response);
}
else {
_handleFormURLEncodedRequest(request, response);
}
}
private void _handleFormURLEncodedRequest(HttpServletRequest request, HttpServletResponse response)
throws IOException
{
// Get request parameters (x-www-form-urlencoded)
String repository = HttpServerUtil.getParameter(request, "repository");
String resultFormatStr = HttpServerUtil.getParameter(request, "resultFormat", AdminResultFormat.XML.toString());
_handleRequest(request, response, repository, resultFormatStr);
}
private void _handleMultipartFormRequest(HttpServletRequest request, HttpServletResponse response)
throws IOException
{
// Get request parameters (multipart/form-data)
Map fileItemMap = HttpServerUtil.parseMultipartFormRequest(request);
String repository = HttpServerUtil.getParameter(fileItemMap, "repository");
String resultFormatStr = HttpServerUtil.getParameter(fileItemMap, "resultFormat", AdminResultFormat.XML.toString());
_handleRequest(request, response, repository, resultFormatStr);
}
private void _handleRequest(HttpServletRequest request, HttpServletResponse response,
String repository, String resultFormatStr)
throws IOException
{
SesameServer.setThreadLogFileForRepository(repository);
_logIP(request);
ThreadLog.log(">>> clear repository");
// Log parameters
ThreadLog.trace("repository = " + repository);
ThreadLog.trace("resultFormat = " + resultFormatStr);
// Check the repository parameter
if (repository == null) {
_sendBadRequest("No repository specified", response);
return;
}
// Check the result format and create an AdminListener
HTTPOutputStream httpOut = new HTTPOutputStream(response);
httpOut.setCacheableResult(false);
AdminResultFormat resultFormat = AdminResultFormat.forValue(resultFormatStr);
AdminListener report;
if (resultFormat == AdminResultFormat.XML) {
httpOut.setContentType("text/xml");
report = new XmlAdminMsgWriter(httpOut);
}
else if (resultFormat == AdminResultFormat.HTML) {
httpOut.setContentType("text/html");
report = new HtmlAdminMsgWriter(httpOut);
}
else {
_sendBadRequest("Unknown result format: " + resultFormatStr, response);
return;
}
try {
LocalService service = SesameServer.getLocalService();
_login(service);
SesameRepository rep = service.getRepository(repository);
// Clear the repository
long startTime = System.currentTimeMillis();
rep.clear(report);
long endTime = System.currentTimeMillis();
ThreadLog.trace("Repository " + repository +
" cleared in " + (endTime - startTime) + "ms");
}
catch (UnknownRepositoryException e) {
_sendBadRequest(HTTPErrorType.UNKNOWN_REPOSITORY, "Unknown repository", response);
}
catch (AccessDeniedException e) {
_sendForbidden("Access denied", response);
}
catch (Exception e) {
ThreadLog.error("Error while clearing repository", e);
report.error("Error while clearing repository: " + e.getMessage(), -1, -1, null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy