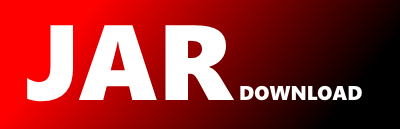
org.openrdf.tools.cmdline.SesameClient Maven / Gradle / Ivy
Go to download
Sesame is an open source Java framework for storing, querying and reasoning with RDF.
The newest version!
/* Command line tool for Sesame
* Copyright (C) 2004 CWI
*
* Contact:
* CWI
* Kruislaan 413
* P.O. Box 94079
* 1090 GB AMSTERDAM
* The Netherlands
* http://www.cwi.nl/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.tools.cmdline;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.URL;
import org.openrdf.sesame.Sesame;
import org.openrdf.sesame.admin.AdminListener;
import org.openrdf.sesame.admin.XmlAdminMsgWriter;
import org.openrdf.sesame.config.AccessDeniedException;
import org.openrdf.sesame.constants.QueryLanguage;
import org.openrdf.sesame.constants.RDFFormat;
import org.openrdf.sesame.query.MalformedQueryException;
import org.openrdf.sesame.query.QueryEvaluationException;
import org.openrdf.sesame.query.XmlQueryResultWriter;
import org.openrdf.sesame.repository.SesameRepository;
import org.openrdf.sesame.repository.SesameService;
/** Simple Sesame command line client
* Basic usage: java \
* -Dserver=URL \
* -Drepository=name \
* -Dcommand=command \
* org.openrdf.tools.cmdline.SesameClient
*
* Optional:
* -Duser=username
* -Dpasswd=passwd
*
* Currently supported commands:
* clear (user/passwd required)
* upload (-Dfile and -Dbase required)
* "construct ..." (full Sesame SeRQL construct query)
* "select ..." (full Sesame SeRQL select query)
*
* Example:
* java -Dserver=http://www.openrdf.org/sesame \
* -Drepository=museum \
* -Dcommand="select * from {s} p {v}"
* org.openrdf.tools.cmdline.SesameClient
*
* @author [email protected]
* @version $Revision: 1.9 $
*
**/
class SesameClient {
/*-------------+
| Variables |
+-------------*/
protected SesameRepository _repository = null;
/*-------------+
| Constructors |
+-------------*/
public SesameClient(URL serverURL, String repository)
throws Exception
{
SesameService service = Sesame.getService(serverURL);
String user = System.getProperty("user");
String passwd = System.getProperty("passwd");
if (user != null && passwd != null) {
service.login(user,passwd);
}
_repository = service.getRepository(repository);
}
public void constructQuery(String q)
throws IOException, MalformedQueryException, AccessDeniedException, QueryEvaluationException
{
// we have to listen to the results of a SeRQL construct query
StreamedGraphWriter l = new StreamedGraphWriter(System.out);
_repository.performGraphQuery(QueryLanguage.SERQL, q, l);
}
public void selectQuery(String q)
throws IOException, MalformedQueryException, AccessDeniedException, QueryEvaluationException
{
// we have to listen to the results of a SeRQL select query
XmlQueryResultWriter l = new XmlQueryResultWriter(System.out);
_repository.performTableQuery(QueryLanguage.SERQL, q, l);
}
public void clear()
throws IOException, AccessDeniedException
{
AdminListener report = new XmlAdminMsgWriter(System.err);
_repository.clear(report);
}
public void upload(String file, String baseURI)
throws IOException, AccessDeniedException
{
FileInputStream is = new FileInputStream(file);
String base = baseURI;
RDFFormat format = RDFFormat.RDFXML;
boolean verify = true;
AdminListener report = new XmlAdminMsgWriter(System.err);
_repository.addData(is, base, format, verify, report);
}
public static void main(String[] args)
throws Exception
{
// Get URL to sesame server:
String server = System.getProperty("server");
if (server == null || server.equals("")) {
throw new IOException("No -Dserver= option provided");
}
URL serverURL = new URL(server);
// Get repository id:
String repository = System.getProperty("repository");
if (repository == null || repository.equals("")) {
throw new IOException("No -Drepository= option provided");
}
// what command do we send the client?
String command = System.getProperty("command");
if (command == null || command.equals("")) {
throw new IOException("No -Dcommand= provided");
}
// set up the client:
SesameClient client = new SesameClient(serverURL, repository);
if (command.startsWith("construct")) {
client.constructQuery(command);
}
else if (command.startsWith("select")) {
client.selectQuery(command);
}
else if (command.startsWith("clear")) {
client.clear();
}
else if (command.startsWith("upload")) {
String RDFfile = System.getProperty("file");
if (RDFfile == null || RDFfile.equals("")) {
throw new IOException("upload but no -Dfile= provided");
}
String BaseURL = System.getProperty("base");
if (BaseURL == null || BaseURL.equals("")) {
throw new IOException("upload but no -Dbase= provided");
}
client.upload(RDFfile, BaseURL);
}
else {
throw new IOException("Unknown command: " + command);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy