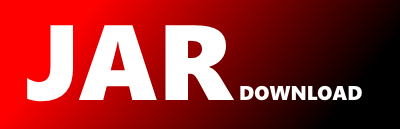
com.google.refine.grel.ControlDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of main Show documentation
Show all versions of main Show documentation
OpenRefine is a free, open source power tool for working with messy data and improving it
// CHECKSTYLE:OFF
package com.google.refine.grel;
import org.jvnet.localizer.Localizable;
import org.jvnet.localizer.ResourceBundleHolder;
/**
* Generated localization support class.
*
*/
@SuppressWarnings({
"",
"PMD",
"all"
})
public class ControlDescription {
/**
* The resource bundle reference
*
*/
private final static ResourceBundleHolder holder = ResourceBundleHolder.get(ControlDescription.class);
/**
* Key {@code foreach_desc}: {@code Evaluates expression a to an array.
* Then for each array element, binds its value to variable name v,
* evaluates expression e, and pushes the result onto the result array.}.
*
* @return
* {@code Evaluates expression a to an array. Then for each array
* element, binds its value to variable name v, evaluates expression e,
* and pushes the result onto the result array.}
*/
public static String foreach_desc() {
return holder.format("foreach_desc");
}
/**
* Key {@code foreach_desc}: {@code Evaluates expression a to an array.
* Then for each array element, binds its value to variable name v,
* evaluates expression e, and pushes the result onto the result array.}.
*
* @return
* {@code Evaluates expression a to an array. Then for each array
* element, binds its value to variable name v, evaluates expression e,
* and pushes the result onto the result array.}
*/
public static Localizable _foreach_desc() {
return new Localizable(holder, "foreach_desc");
}
/**
* Key {@code is_not_null_desc}: {@code Returns whether o is not null}.
*
* @return
* {@code Returns whether o is not null}
*/
public static String is_not_null_desc() {
return holder.format("is_not_null_desc");
}
/**
* Key {@code is_not_null_desc}: {@code Returns whether o is not null}.
*
* @return
* {@code Returns whether o is not null}
*/
public static Localizable _is_not_null_desc() {
return new Localizable(holder, "is_not_null_desc");
}
/**
* Key {@code is_non_blank_desc}: {@code Returns whether o is not null
* and not an empty string}.
*
* @return
* {@code Returns whether o is not null and not an empty string}
*/
public static String is_non_blank_desc() {
return holder.format("is_non_blank_desc");
}
/**
* Key {@code is_non_blank_desc}: {@code Returns whether o is not null
* and not an empty string}.
*
* @return
* {@code Returns whether o is not null and not an empty string}
*/
public static Localizable _is_non_blank_desc() {
return new Localizable(holder, "is_non_blank_desc");
}
/**
* Key {@code for_non_blank_desc}: {@code Evaluates expression o. If it
* is non-blank, binds its value to variable name v, evaluates expression
* eNonBlank and returns the result. Otherwise (if o evaluates to blank),
* evaluates expression eBlank and returns that result instead.}.
*
* @return
* {@code Evaluates expression o. If it is non-blank, binds its value to
* variable name v, evaluates expression eNonBlank and returns the
* result. Otherwise (if o evaluates to blank), evaluates expression
* eBlank and returns that result instead.}
*/
public static String for_non_blank_desc() {
return holder.format("for_non_blank_desc");
}
/**
* Key {@code for_non_blank_desc}: {@code Evaluates expression o. If it
* is non-blank, binds its value to variable name v, evaluates expression
* eNonBlank and returns the result. Otherwise (if o evaluates to blank),
* evaluates expression eBlank and returns that result instead.}.
*
* @return
* {@code Evaluates expression o. If it is non-blank, binds its value to
* variable name v, evaluates expression eNonBlank and returns the
* result. Otherwise (if o evaluates to blank), evaluates expression
* eBlank and returns that result instead.}
*/
public static Localizable _for_non_blank_desc() {
return new Localizable(holder, "for_non_blank_desc");
}
/**
* Key {@code is_test_desc}: {@code Returns whether o can represent a
* number}.
*
* @return
* {@code Returns whether o can represent a number}
*/
public static String is_test_desc() {
return holder.format("is_test_desc");
}
/**
* Key {@code is_test_desc}: {@code Returns whether o can represent a
* number}.
*
* @return
* {@code Returns whether o can represent a number}
*/
public static Localizable _is_test_desc() {
return new Localizable(holder, "is_test_desc");
}
/**
* Key {@code is_blank_desc}: {@code Returns whether o is null or an
* empty string}.
*
* @return
* {@code Returns whether o is null or an empty string}
*/
public static String is_blank_desc() {
return holder.format("is_blank_desc");
}
/**
* Key {@code is_blank_desc}: {@code Returns whether o is null or an
* empty string}.
*
* @return
* {@code Returns whether o is null or an empty string}
*/
public static Localizable _is_blank_desc() {
return new Localizable(holder, "is_blank_desc");
}
/**
* Key {@code filter_desc}: {@code Evaluates expression a to an array.
* Then for each array element, binds its value to variable name v,
* evaluates expression test which should return a boolean. If the
* boolean is true, pushes v onto the result array.}.
*
* @return
* {@code Evaluates expression a to an array. Then for each array
* element, binds its value to variable name v, evaluates expression test
* which should return a boolean. If the boolean is true, pushes v onto
* the result array.}
*/
public static String filter_desc() {
return holder.format("filter_desc");
}
/**
* Key {@code filter_desc}: {@code Evaluates expression a to an array.
* Then for each array element, binds its value to variable name v,
* evaluates expression test which should return a boolean. If the
* boolean is true, pushes v onto the result array.}.
*
* @return
* {@code Evaluates expression a to an array. Then for each array
* element, binds its value to variable name v, evaluates expression test
* which should return a boolean. If the boolean is true, pushes v onto
* the result array.}
*/
public static Localizable _filter_desc() {
return new Localizable(holder, "filter_desc");
}
/**
* Key {@code if_desc}: {@code Evaluates expression o. If it is true,
* evaluates expression eTrue and returns the result. Otherwise,
* evaluates expression eFalse and returns that result instead.}.
*
* @return
* {@code Evaluates expression o. If it is true, evaluates expression
* eTrue and returns the result. Otherwise, evaluates expression eFalse
* and returns that result instead.}
*/
public static String if_desc() {
return holder.format("if_desc");
}
/**
* Key {@code if_desc}: {@code Evaluates expression o. If it is true,
* evaluates expression eTrue and returns the result. Otherwise,
* evaluates expression eFalse and returns that result instead.}.
*
* @return
* {@code Evaluates expression o. If it is true, evaluates expression
* eTrue and returns the result. Otherwise, evaluates expression eFalse
* and returns that result instead.}
*/
public static Localizable _if_desc() {
return new Localizable(holder, "if_desc");
}
/**
* Key {@code is_error_desc}: {@code Returns whether o is an error}.
*
* @return
* {@code Returns whether o is an error}
*/
public static String is_error_desc() {
return holder.format("is_error_desc");
}
/**
* Key {@code is_error_desc}: {@code Returns whether o is an error}.
*
* @return
* {@code Returns whether o is an error}
*/
public static Localizable _is_error_desc() {
return new Localizable(holder, "is_error_desc");
}
/**
* Key {@code is_null_desc}: {@code Returns whether o is null}.
*
* @return
* {@code Returns whether o is null}
*/
public static String is_null_desc() {
return holder.format("is_null_desc");
}
/**
* Key {@code is_null_desc}: {@code Returns whether o is null}.
*
* @return
* {@code Returns whether o is null}
*/
public static Localizable _is_null_desc() {
return new Localizable(holder, "is_null_desc");
}
/**
* Key {@code is_numeric_desc}: {@code Returns whether o can represent a
* number}.
*
* @return
* {@code Returns whether o can represent a number}
*/
public static String is_numeric_desc() {
return holder.format("is_numeric_desc");
}
/**
* Key {@code is_numeric_desc}: {@code Returns whether o can represent a
* number}.
*
* @return
* {@code Returns whether o can represent a number}
*/
public static Localizable _is_numeric_desc() {
return new Localizable(holder, "is_numeric_desc");
}
/**
* Key {@code with_desc}: {@code Evaluates expression o and binds its
* value to variable name v. Then evaluates expression e and returns that
* result}.
*
* @return
* {@code Evaluates expression o and binds its value to variable name v.
* Then evaluates expression e and returns that result}
*/
public static String with_desc() {
return holder.format("with_desc");
}
/**
* Key {@code with_desc}: {@code Evaluates expression o and binds its
* value to variable name v. Then evaluates expression e and returns that
* result}.
*
* @return
* {@code Evaluates expression o and binds its value to variable name v.
* Then evaluates expression e and returns that result}
*/
public static Localizable _with_desc() {
return new Localizable(holder, "with_desc");
}
/**
* Key {@code isempty_string_desc}: {@code Returns whether o is an empty
* string}.
*
* @return
* {@code Returns whether o is an empty string}
*/
public static String isempty_string_desc() {
return holder.format("isempty_string_desc");
}
/**
* Key {@code isempty_string_desc}: {@code Returns whether o is an empty
* string}.
*
* @return
* {@code Returns whether o is an empty string}
*/
public static Localizable _isempty_string_desc() {
return new Localizable(holder, "isempty_string_desc");
}
/**
* Key {@code for_range_desc}: {@code Iterates over the variable v
* starting at "from", incrementing by "step" each time while less than
* "to". At each iteration, evaluates expression e, and pushes the result
* onto the result array.}.
*
* @return
* {@code Iterates over the variable v starting at "from", incrementing
* by "step" each time while less than "to". At each iteration, evaluates
* expression e, and pushes the result onto the result array.}
*/
public static String for_range_desc() {
return holder.format("for_range_desc");
}
/**
* Key {@code for_range_desc}: {@code Iterates over the variable v
* starting at "from", incrementing by "step" each time while less than
* "to". At each iteration, evaluates expression e, and pushes the result
* onto the result array.}.
*
* @return
* {@code Iterates over the variable v starting at "from", incrementing
* by "step" each time while less than "to". At each iteration, evaluates
* expression e, and pushes the result onto the result array.}
*/
public static Localizable _for_range_desc() {
return new Localizable(holder, "for_range_desc");
}
/**
* Key {@code foreach_index_desc}: {@code Evaluates expression a to an
* array. Then for each array element, binds its index to variable i and
* its value to variable name v, evaluates expression e, and pushes the
* result onto the result array.}.
*
* @return
* {@code Evaluates expression a to an array. Then for each array
* element, binds its index to variable i and its value to variable name
* v, evaluates expression e, and pushes the result onto the result
* array.}
*/
public static String foreach_index_desc() {
return holder.format("foreach_index_desc");
}
/**
* Key {@code foreach_index_desc}: {@code Evaluates expression a to an
* array. Then for each array element, binds its index to variable i and
* its value to variable name v, evaluates expression e, and pushes the
* result onto the result array.}.
*
* @return
* {@code Evaluates expression a to an array. Then for each array
* element, binds its index to variable i and its value to variable name
* v, evaluates expression e, and pushes the result onto the result
* array.}
*/
public static Localizable _foreach_index_desc() {
return new Localizable(holder, "foreach_index_desc");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy