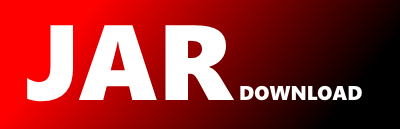
org.openrewrite.kubernetes.tree.K8S Maven / Gradle / Ivy
Show all versions of rewrite-kubernetes Show documentation
/*
* Copyright 2021 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openrewrite.kubernetes.tree;
import lombok.AccessLevel;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.With;
import lombok.experimental.FieldDefaults;
import org.jspecify.annotations.Nullable;
import org.openrewrite.Cursor;
import org.openrewrite.kubernetes.resource.ResourceLimit;
import org.openrewrite.yaml.JsonPathMatcher;
import org.openrewrite.yaml.tree.Yaml;
import java.util.*;
import java.util.regex.Pattern;
import static java.util.Collections.emptySet;
import static org.openrewrite.Tree.randomId;
/**
* @deprecated Likely better served by {@link org.openrewrite.kubernetes.trait.Traits}.
*/
@Deprecated
public interface K8S {
static boolean inKind(String kind, Cursor cursor) {
Yaml.Document doc = cursor.firstEnclosing(Yaml.Document.class);
if (doc == null) {
return false;
}
Resource r = K8S.asResource((Yaml.Mapping) doc.getBlock());
return kind.equals(r.getKind());
}
static boolean inPod(Cursor cursor) {
return inKind("Pod", cursor);
}
static boolean inDaemonSet(Cursor cursor) {
return inKind("DaemonSet", cursor);
}
static boolean inStatefulSet(Cursor cursor) {
return inKind("StatefulSet", cursor);
}
static boolean inDeployment(Cursor cursor) {
return inKind("Deployment", cursor);
}
static boolean inService(Cursor cursor) {
return inKind("Service", cursor);
}
static Resource asResource(Yaml.Mapping m) {
String apiVersion = null;
String kind = null;
for (Yaml.Mapping.Entry e : m.getEntries()) {
Yaml.Block value = e.getValue();
if ("apiVersion".equals(e.getKey().getValue())) {
apiVersion = ((Yaml.Scalar) value).getValue();
} else if ("kind".equals(e.getKey().getValue())) {
kind = ((Yaml.Scalar) value).getValue();
}
}
return new Resource(randomId(), apiVersion, kind);
}
@SuppressWarnings("ConstantConditions")
static Metadata asMetadata(Yaml.Mapping m) {
String namespace = null;
String name = "";
Annotations annotations = null;
Labels labels = null;
for (Yaml.Mapping.Entry e : m.getEntries()) {
String key = e.getKey().getValue();
Object value;
if (e.getValue() instanceof Yaml.Scalar) {
value = ((Yaml.Scalar) e.getValue()).getValue();
} else {
value = e.getValue();
}
switch (key) {
case "namespace":
namespace = (String) value;
break;
case "name":
name = (String) value;
break;
case "labels":
labels = asLabels((Yaml.Mapping) value);
break;
case "annotations":
annotations = asAnnotations((Yaml.Mapping) value);
break;
}
}
return new Metadata(randomId(), namespace, name, annotations, labels);
}
static Annotations asAnnotations(Yaml.@Nullable Mapping m) {
if (m == null) {
return new Annotations(randomId(), emptySet());
}
Set keys = new HashSet<>();
for (Yaml.Mapping.Entry e : m.getEntries()) {
keys.add(e.getKey().getValue());
}
return new Annotations(randomId(), keys);
}
static Labels asLabels(Yaml.@Nullable Mapping m) {
if (m == null) {
return new Labels(randomId(), emptySet());
}
Set keys = new HashSet<>();
for (Yaml.Mapping.Entry e : m.getEntries()) {
keys.add(e.getKey().getValue());
}
return new Labels(randomId(), keys);
}
static ResourceLimits asResourceLimits(Yaml.@Nullable Scalar s) {
if (null == s) {
return new ResourceLimits(randomId(), null);
} else {
return new ResourceLimits(randomId(), new ResourceLimit(s.getValue()));
}
}
static @Nullable Service asService(Yaml.@Nullable Mapping m) {
if (m == null) {
return null;
}
return new Service(randomId(), m.getEntries().stream()
.filter(e -> "type".equals(e.getKey().getValue()))
.findFirst()
.map(e -> ((Yaml.Scalar) e.getValue()).getValue())
.orElse("ClusterIP"));
}
static boolean inMappingEntry(String jsonPath, @Nullable Cursor cursor) {
return inMappingEntry(new JsonPathMatcher(jsonPath), cursor);
}
static boolean inMappingEntry(JsonPathMatcher jsonPath, @Nullable Cursor cursor) {
return firstEnclosingEntryMatching(jsonPath, cursor).isPresent();
}
static Optional firstEnclosingEntryMatching(String jsonPath, @Nullable Cursor cursor) {
return firstEnclosingEntryMatching(new JsonPathMatcher(jsonPath), cursor);
}
static Optional firstEnclosingEntryMatching(JsonPathMatcher jsonPath, @Nullable Cursor cursor) {
if (cursor == null) {
return Optional.empty();
}
Yaml.Mapping.Entry e = cursor.getValue() instanceof Yaml.Mapping.Entry ? cursor.getValue() : cursor.firstEnclosing(Yaml.Mapping.Entry.class);
if (e == null || e.getKey() == cursor.getValue()) {
return Optional.empty();
}
return jsonPath.find(cursor)
.flatMap(found -> {
if (found instanceof Yaml.Mapping) {
return ((Yaml.Mapping) found).getEntries().stream()
.map(o -> {
if (o.getValue() == cursor.getValue()) {
return cursor;
}
return null;
}).filter(Objects::nonNull)
.findFirst();
} else if (found instanceof List) {
//noinspection unchecked
return ((List