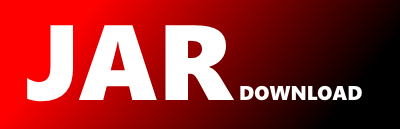
org.openrewrite.java.liberty.RemoveWas2LibertyNonPortableJndiLookup Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openrewrite.java.liberty;
import org.jspecify.annotations.Nullable;
import org.openrewrite.ExecutionContext;
import org.openrewrite.ScanningRecipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.java.JavaIsoVisitor;
import org.openrewrite.java.MethodMatcher;
import org.openrewrite.java.tree.Expression;
import org.openrewrite.java.tree.J;
import org.openrewrite.java.tree.JavaType;
import org.openrewrite.staticanalysis.RemoveUnusedLocalVariables;
import org.openrewrite.staticanalysis.RemoveUnusedPrivateFields;
import java.util.HashSet;
import java.util.Set;
public class RemoveWas2LibertyNonPortableJndiLookup extends ScanningRecipe> {
final private String INITIAL_PROPERTY = "java.naming.factory.initial";
final private String URL_PROPERTY = "java.naming.provider.url";
@Override
public String getDisplayName() {
return "Removes invalid JNDI properties";
}
@Override
public String getDescription() {
return "Remove the use of invalid JNDI properties from Hashtable.";
}
@Override
public Set getInitialValue(ExecutionContext ctx) {
return new HashSet<>();
}
@Override
public TreeVisitor, ExecutionContext> getScanner(Set acc) {
return new JavaIsoVisitor() {
@Override
public J.VariableDeclarations visitVariableDeclarations(J.VariableDeclarations vd, ExecutionContext ctx) {
for (J.VariableDeclarations.NamedVariable variable : vd.getVariables()) {
Expression initializer = variable.getInitializer();
if (initializer != null) {
checkForPropertiesVariable(variable.getVariableType(), initializer);
}
}
return vd;
}
@Override
public J.Assignment visitAssignment(J.Assignment assignment, ExecutionContext ctx) {
Expression assignmentVariable = assignment.getVariable();
if (!(assignmentVariable instanceof J.Identifier)) {
return assignment;
}
J.Identifier assignmentVariableIdentifier = (J.Identifier) assignmentVariable;
JavaType.Variable variable = assignmentVariableIdentifier.getFieldType();
if (!checkForPropertiesVariable(variable, assignment.getAssignment())) {
// If present, remove the variable from the accumulator since it was reassigned to an unrelated value
acc.remove(variable);
}
return assignment;
}
// Add a variable to the accumulator if it matches either property
private boolean checkForPropertiesVariable(JavaType.Variable variable, Expression value) {
if (value instanceof J.Literal) {
String stringValue = ((J.Literal) value).toString();
if (stringValue.equals(INITIAL_PROPERTY) || stringValue.equals(URL_PROPERTY)) {
acc.add(variable);
return true;
}
}
return false;
}
};
}
@Override
public TreeVisitor, ExecutionContext> getVisitor(Set acc) {
MethodMatcher methodMatcher = new MethodMatcher("java.util.Hashtable put(..)", false);
return new JavaIsoVisitor() {
@Override
public J.@Nullable MethodInvocation visitMethodInvocation(J.MethodInvocation mi, ExecutionContext ctx) {
// Return if this method does not match Hashtable.put()
if (!methodMatcher.matches(mi)) {
return mi;
}
Expression firstArgument = mi.getArguments().get(0);
if (firstArgument instanceof J.Literal) {
// Return if the first argument is a literal and does not match either property
String stringValue = ((J.Literal) firstArgument).toString();
if (!stringValue.equals(INITIAL_PROPERTY) && !stringValue.equals(URL_PROPERTY)) {
return mi;
}
} else if (firstArgument instanceof J.Identifier) {
// Return if the first argument is a variable and does not match the type of any collected variables
if (!acc.contains(((J.Identifier) firstArgument).getFieldType())) {
return mi;
} else {
// Remove the variable if this was the only use
doAfterVisit(new RemoveUnusedLocalVariables(null, null).getVisitor());
doAfterVisit(new RemoveUnusedPrivateFields().getVisitor());
}
}
return null;
}
};
}
}