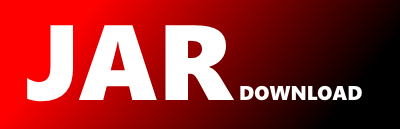
org.openrewrite.java.testing.jmockit.ArgumentMatchersRewriter Maven / Gradle / Ivy
Show all versions of rewrite-testing-frameworks Show documentation
/*
* Copyright 2024 the original author or authors.
*
* Licensed under the Moderne Source Available License (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://docs.moderne.io/licensing/moderne-source-available-license
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openrewrite.java.testing.jmockit;
import org.openrewrite.Cursor;
import org.openrewrite.ExecutionContext;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.tree.*;
import java.util.*;
class ArgumentMatchersRewriter {
private static final Set JMOCKIT_ARGUMENT_MATCHERS = new HashSet<>();
static {
JMOCKIT_ARGUMENT_MATCHERS.add("anyString");
JMOCKIT_ARGUMENT_MATCHERS.add("anyInt");
JMOCKIT_ARGUMENT_MATCHERS.add("anyLong");
JMOCKIT_ARGUMENT_MATCHERS.add("anyDouble");
JMOCKIT_ARGUMENT_MATCHERS.add("anyFloat");
JMOCKIT_ARGUMENT_MATCHERS.add("anyBoolean");
JMOCKIT_ARGUMENT_MATCHERS.add("anyByte");
JMOCKIT_ARGUMENT_MATCHERS.add("anyChar");
JMOCKIT_ARGUMENT_MATCHERS.add("anyShort");
JMOCKIT_ARGUMENT_MATCHERS.add("any");
}
private static final Map FQN_TO_MOCKITO_ARGUMENT_MATCHER = new HashMap<>();
static {
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.util.List", "anyList");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.util.Set", "anySet");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.util.Collection", "anyCollection");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.util.Iterable", "anyIterable");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.util.Map", "anyMap");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Integer", "anyInt");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Long", "anyLong");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Double", "anyDouble");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Float", "anyFloat");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Boolean", "anyBoolean");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Byte", "anyByte");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Character", "anyChar");
FQN_TO_MOCKITO_ARGUMENT_MATCHER.put("java.lang.Short", "anyShort");
}
private static final Map PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER = new HashMap<>();
static {
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Int, "anyInt");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Long, "anyLong");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Double, "anyDouble");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Float, "anyFloat");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Boolean, "anyBoolean");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Byte, "anyByte");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Char, "anyChar");
PRIMITIVE_TO_MOCKITO_ARGUMENT_MATCHER.put(JavaType.Primitive.Short, "anyShort");
}
private final JavaVisitor visitor;
private final ExecutionContext ctx;
private final J.Block expectationsBlock;
ArgumentMatchersRewriter(JavaVisitor visitor, ExecutionContext ctx, J.Block expectationsBlock) {
this.visitor = visitor;
this.ctx = ctx;
this.expectationsBlock = expectationsBlock;
}
J.Block rewriteJMockitBlock() {
List newStatements = new ArrayList<>(expectationsBlock.getStatements().size());
for (Statement expectationStatement : expectationsBlock.getStatements()) {
// for each statement, check if it's a method invocation and replace any argument matchers
if (!(expectationStatement instanceof J.MethodInvocation)) {
newStatements.add(expectationStatement);
continue;
}
newStatements.add(rewriteMethodInvocation((J.MethodInvocation) expectationStatement));
}
return expectationsBlock.withStatements(newStatements);
}
private J.MethodInvocation rewriteMethodInvocation(J.MethodInvocation invocation) {
if (invocation.getSelect() instanceof J.MethodInvocation) {
invocation = invocation.withSelect(rewriteMethodInvocation((J.MethodInvocation) invocation.getSelect()));
}
// in mockito, argument matchers must be used for all arguments or none
List arguments = invocation.getArguments();
// replace this.matcher with matcher, otherwise it's ignored
arguments.replaceAll(arg -> {
if (arg instanceof J.FieldAccess) {
J.FieldAccess fieldAccess = (J.FieldAccess) arg;
if (fieldAccess.getTarget() instanceof J.Identifier &&
"this".equals(((J.Identifier) fieldAccess.getTarget()).getSimpleName())) {
return fieldAccess.getName();
}
}
return arg;
});
// if there are no argument matchers, return the invocation as-is
if (arguments.stream().noneMatch(ArgumentMatchersRewriter::isJmockitArgumentMatcher)) {
return invocation;
}
// replace each argument with the appropriate argument matcher
List newArguments = new ArrayList<>(arguments.size());
for (Expression argument : arguments) {
newArguments.add(rewriteMethodArgument(argument));
}
return invocation.withArguments(newArguments);
}
private Expression rewriteMethodArgument(Expression methodArgument) {
String argumentMatcher = null, template = null;
List