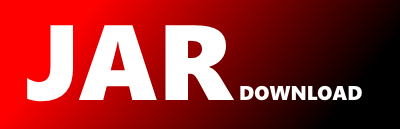
commonMain.dsl.shadestyle.ShadeStyleBuilder.kt Maven / Gradle / Ivy
package org.openrndr.orsl.shadergenerator.dsl.shadestyle
import org.openrndr.draw.*
import org.openrndr.draw.font.BufferAccess
import org.openrndr.draw.font.BufferFlag
import org.openrndr.orsl.shadergenerator.dsl.ShaderBuilder
import org.openrndr.orsl.shadergenerator.dsl.*
import org.openrndr.orsl.shadergenerator.phrases.*
import org.openrndr.orsl.shadergenerator.dsl.functions.FragmentDerivativeFunctions
import org.openrndr.draw.parameter as structParameter
import org.openrndr.math.Matrix44
import org.openrndr.math.Vector2
import org.openrndr.math.Vector3
import org.openrndr.math.Vector4
import kotlin.reflect.KProperty
open class ShadeStyleBuilder : ShaderBuilder(emptySet()), TransformPhrasesFunctions {
class ParameterProperty(val type: String, val register: (name: String) -> Unit = {}) {
operator fun provideDelegate(any: Any?, property: KProperty<*>): ParameterProperty {
register(property.name.replace("p_", ""))
return this
}
operator fun getValue(any: Any?, property: KProperty<*>) = symbol(property.name, type)
}
class BufferProperty(
val type: String,
val register: (name: String) -> Unit
) {
operator fun provideDelegate(any: Any?, property: KProperty<*>): BufferProperty {
register(property.name.replace("b_", ""))
return this
}
operator fun getValue(any: Any?, property: KProperty<*>) = symbol(property.name, type)
}
class ImageProperty(
val type: String,
val register: (name: String) -> Unit
) {
operator fun provideDelegate(any: Any?, property: KProperty<*>): ImageProperty {
register(property.name.replace("p_", ""))
return this
}
operator fun getValue(any: Any?, property: KProperty<*>) = symbol(property.name, type)
}
class ArrayImageProperty(
val type: String,
val length: Int,
val register: (name: String) -> Unit
) {
operator fun provideDelegate(any: Any?, property: KProperty<*>): ArrayImageProperty {
register(property.name.replace("p_", ""))
return this
}
operator fun getValue(any: Any?, property: KProperty<*>) = arraySymbol(property.name, length, type)
}
class ArrayParameter(val type: String, val length: Int) {
operator fun getValue(any: Any?, property: KProperty<*>) = arraySymbol(property.name, length, type)
}
inline fun parameter(): ParameterProperty {
return ParameterProperty(staticType())
}
inline fun vertexAttribute(): ParameterProperty {
return ParameterProperty(staticType())
}
inline fun instanceAttribute(): ParameterProperty {
return ParameterProperty(staticType())
}
inline fun > StyleParameters.parameter(value: T): ParameterProperty {
return ParameterProperty(staticType()) { name ->
(this@parameter).structParameter(name.replace("p_", ""), value)
}
}
inline fun > StyleParameters.parameter(value: Array): ParameterProperty {
return ParameterProperty(staticType()) { name ->
(this@parameter).structParameter(name.replace("p_", ""), value)
}
}
fun StyleParameters.parameter(value: Vector3): ParameterProperty {
return ParameterProperty(staticType()) { name ->
(this@parameter).parameter(name.replace("p_", ""), value)
}
}
fun StyleParameters.parameter(value: Double): ParameterProperty {
return ParameterProperty(staticType()) { name ->
(this@parameter).parameter(name.replace("p_", ""), value)
}
}
inline fun > StyleBufferBindings.buffer(
access: BufferAccess = BufferAccess.READ_WRITE,
vararg flags: BufferFlag
): BufferProperty {
return BufferProperty(staticType()) { name ->
registerStructuredBuffer(name, access, flags.toSet())
}
}
inline fun > StyleBufferBindings.buffer(
structuredBuffer: StructuredBuffer,
access: BufferAccess = BufferAccess.READ_WRITE,
vararg flags: BufferFlag
): BufferProperty {
return BufferProperty(staticType()) { name ->
registerStructuredBuffer(name, access, flags.toSet())
[email protected](name, structuredBuffer.ssbo)
}
}
/**
* Atomic counter buffer with default binding
*/
fun StyleBufferBindings.buffer(
atomicCounterBuffer: AtomicCounterBuffer,
access: BufferAccess = BufferAccess.READ_WRITE,
vararg flags: BufferFlag
): BufferProperty {
return BufferProperty(staticType()) { name ->
[email protected](name, atomicCounterBuffer)
}
}
inline fun StyleImageBindings.image(access: ImageAccess = ImageAccess.READ_WRITE, flags: Set = emptySet()): ImageProperty {
return ImageProperty(staticType()) { name ->
[email protected](name, access, flags)
}
}
inline fun StyleImageBindings.image(
colorBuffer: ColorBuffer,
level: Int = 0,
access: ImageAccess = ImageAccess.READ_WRITE,
flags: Set = emptySet()
): ImageProperty {
return ImageProperty(staticType()) { name ->
[email protected](name, access, flags)
[email protected](name, colorBuffer, level)
}
}
inline fun StyleImageBindings.images(
colorBuffers: Array,
levels: Array,
access: ImageAccess = ImageAccess.READ_WRITE,
flags: Set = emptySet()
): ArrayImageProperty {
return ArrayImageProperty(staticType(), colorBuffers.size) { name ->
[email protected](name, access, flags)
[email protected](name, colorBuffers, levels)
}
}
inline fun StyleImageBindings.image(
volumeTexture: VolumeTexture,
level: Int,
access: ImageAccess = ImageAccess.READ_WRITE,
flags: Set = emptySet()
): ImageProperty {
return ImageProperty(staticType()) { name ->
[email protected](name, access, flags)
[email protected](name, volumeTexture)
}
}
inline fun StyleImageBindings.images(
size: Int,
access: ImageAccess = ImageAccess.READ_WRITE,
flags: Set = emptySet()
): ArrayImageProperty {
return ArrayImageProperty(staticType(), size) { name ->
[email protected](name, access, flags)
}
}
/**
* Reference to an already declared image binding
*/
inline fun StyleImageBindings.images(): ArrayImageProperty {
return ArrayImageProperty(staticType(), 0) { name ->
require(imageAccess.containsKey(name.replace("p_", "")))
}
}
inline fun StyleImageBindings.images(
volumeTextures: Array,
levels: Array,
access: ImageAccess = ImageAccess.READ_WRITE,
flags: Set = emptySet()
): ArrayImageProperty {
return ArrayImageProperty(staticType(), volumeTextures.size) { name ->
[email protected](name, access, flags)
[email protected](name, volumeTextures, levels)
}
}
inline fun arrayParameter(length: Int): ArrayParameter {
return ArrayParameter(staticType(), length)
}
val c_boundsPosition by parameter()
val c_boundsSize by parameter()
val c_screenPosition by parameter()
val c_contourPosition by parameter()
val c_instance by parameter()
val c_element by parameter()
}
class VertexTransformBuilder() : ShadeStyleBuilder(){
var x_position by output()
var x_normal by output()
var x_viewMatrix by output()
var x_modelMatrix by output()
var x_modelNormalMatrix by output()
var x_viewNormalMatrix by output()
var x_projectionMatrix by output()
inline fun varyingOut(): VaryingOutProperty {
val glslType = staticType()
return VaryingOutProperty(this@VertexTransformBuilder, glslType)
}
}
class FragmentTransformBuilder() : ShadeStyleBuilder(), FragmentDerivativeFunctions {
var color by output()
var x_fill by output()
var x_stroke by output()
val v_worldNormal by parameter()
val v_viewNormal by parameter()
val v_worldPosition by parameter()
val v_viewPosition by parameter()
val v_clipPosition by parameter()
val u_viewMatrix by parameter()
val u_modelMatrix by parameter()
val u_modelNormalMatrix by parameter()
val u_viewNormalMatrix by parameter()
/**
* declare a varying in
*/
inline fun varyingIn(forceDefinition: Boolean = false): VaryingInProperty {
val glslType = staticType()
return VaryingInProperty(this@FragmentTransformBuilder, glslType, forceDefinition)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy