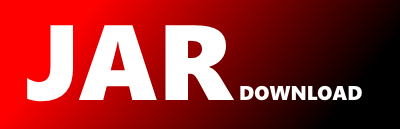
commonMain.dsl.functions.IntFunctions.kt Maven / Gradle / Ivy
The newest version!
package org.openrndr.orsl.shadergenerator.dsl.functions
import org.openrndr.orsl.shadergenerator.dsl.Symbol
import org.openrndr.orsl.shadergenerator.dsl.functionSymbol
import org.openrndr.orsl.shadergenerator.dsl.glsl
import org.openrndr.orsl.shadergenerator.dsl.symbol
import kotlin.jvm.JvmName
@Suppress("INAPPLICABLE_JVM_NAME")
interface IntFunctions {
@JvmName("absSi")
fun abs(x: Symbol): Symbol = functionSymbol(x, "abs($0)")
@JvmName("modSiSi")
fun mod(a: Symbol, b: Symbol): Symbol = functionSymbol(a, b, "mod($0, $1)")
@JvmName("modSiVi")
fun mod(a: Symbol, b: Int): Symbol = functionSymbol(a, b, "mod($0, $1)")
@JvmName("minSiSi")
fun min(a: Symbol, b: Symbol): Symbol = functionSymbol(a, b, "min($0, $1)")
@JvmName("maxSisi")
fun max(a: Symbol, b: Symbol): Symbol = functionSymbol(a, b, "max($0, $1)")
@JvmName("unaryMinusSi")
operator fun Symbol.unaryMinus(): Symbol =
functionSymbol(this, "(-$0)")
@JvmName("shlSiSi")
infix fun Symbol.shl(right: Symbol): Symbol = functionSymbol(this, right, "($0 << $1)")
@JvmName("shrSiSi")
infix fun Symbol.shr(right: Symbol): Symbol = functionSymbol(this, right, "($0 >> $1)")
@JvmName("plusSiSi")
operator fun Symbol.plus(right: Symbol): Symbol = functionSymbol(this, right, "($0 + $1)")
@JvmName("plusSiSd")
operator fun Symbol.plus(right: Symbol): Symbol = functionSymbol(this, right, "(float($0) + $1)")
@JvmName("plusSiVi")
operator fun Symbol.plus(right: Int): Symbol = functionSymbol(this, right, "($0 + $1)")
@JvmName("plusSiVd")
operator fun Symbol.plus(right: Double): Symbol = functionSymbol(this, right, "(float($0) + $1)")
@JvmName("minusSiSi")
operator fun Symbol.minus(right: Symbol): Symbol = functionSymbol(this, right, "($0 - $1)")
@JvmName("minusSiVi")
operator fun Symbol.minus(right: Int): Symbol = functionSymbol(this, right, "($0 - $1)")
@JvmName("timesSiSi")
operator fun Symbol.times(right: Symbol): Symbol = functionSymbol(this, right, "($0 * $1)")
@JvmName("timesSiVi")
operator fun Symbol.times(right: Int): Symbol = functionSymbol(this, right, "($0 * $1)")
@JvmName("timesSiVd")
operator fun Symbol.times(right: Double): Symbol = functionSymbol(this, right, "(float($0) * $1)")
@JvmName("divSiSi")
operator fun Symbol.div(right: Symbol): Symbol = functionSymbol(this, right, "($0 / $1)")
@JvmName("divSiSd")
operator fun Symbol.div(right: Symbol): Symbol = functionSymbol(this, right, "(float($0) / $1)")
@JvmName("divSiVd")
operator fun Symbol.div(right: Double): Symbol = functionSymbol(this, right, "(float($0) / $1)")
@JvmName("eqSiSi")
infix fun Symbol.eq(right: Symbol): Symbol = functionSymbol(this, right, "($0 == $1)")
@JvmName("eqSiVi")
infix fun Symbol.eq(right: Int): Symbol = functionSymbol(this, right, "($0 == $1)")
@JvmName("neqSiSi")
infix fun Symbol.neq(right: Symbol): Symbol = functionSymbol(this, right, "($0 != $1)")
@JvmName("neqSiVi")
infix fun Symbol.neq(right: Int): Symbol = functionSymbol(this, right, "($0 != $1)")
@JvmName("gteSiSi")
infix fun Symbol.gte(right: Symbol): Symbol = functionSymbol(this, right, "($0 >= $1)")
@JvmName("gteSiVi")
infix fun Symbol.gte(right: Int): Symbol = functionSymbol(this, right, "($0 >= $1)")
@JvmName("gtSiSi")
infix fun Symbol.gt(right: Symbol): Symbol = functionSymbol(this, right, "($0 > $1)")
@JvmName("gtSiVi")
infix fun Symbol.gt(right: Int): Symbol = functionSymbol(this, right, "($0 > $1)")
@JvmName("ltSiSi")
infix fun Symbol.lt(right: Symbol): Symbol = functionSymbol(this, right, "($0 < $1)")
@JvmName("ltSiVi")
infix fun Symbol.lt(right: Int): Symbol = functionSymbol(this, right, "($0 < $1)")
@JvmName("lteSiSi")
infix fun Symbol.lte(right: Symbol): Symbol = functionSymbol(this, right, "($0 <= $1)")
@JvmName("lteSiVi")
infix fun Symbol.lte(right: Int): Symbol = functionSymbol(this, right, "($0 <= $1)")
/**
* find the index of the most significant bit set to 1 in an integer
* @since GLSL 4.00
*/
@JvmName("findMSBSi")
fun Symbol.findMSB(): Symbol = functionSymbol(this, "findMSB($0)")
/**
* find the index of the least significant bit set to 1 in an integer
* @since GLSL 4.00
*/
@JvmName("findLSBSi")
fun Symbol.findLSB(): Symbol = functionSymbol(this, "findLSB($0)")
/**
* produce a floating point using an encoding supplied as an integer
* @since GLSL 3.30
*/
@JvmName("bitsToFloatSi")
fun Symbol.bitsToFloat(): Symbol = functionSymbol(this, "intBitsToFloat($0)")
/**
* counts the number of 1-bits in an integer
* @since GLSL 4.00
*/
@JvmName("bitCountSi")
fun Symbol.bitCount(): Symbol = functionSymbol(this, "bitCount($0)")
/**
* extract a range of bits from an integer
* @param offset Specifies the index of the first bit to extract.
* @param bits Specifies the number of bits to extract.
* @since GLSL 4.00
*/
@JvmName("bitfieldExtractSiSiSi")
fun Symbol.bitfieldExtract(offset: Symbol, bits: Symbol): Symbol =
functionSymbol(this, offset, bits, "bitfieldExtract($0, $1, $2)")
/**
* insert a range of bits into an integer
* @param insert Specifies the value of the bits to insert.
* @param offset Specifies the index of the first bit to insert.
* @param bits Specifies the number of bits to insert.
* @since GLSL 4.00
*/
@JvmName("bitfieldInsertSiSiSiSi")
fun Symbol.bitfieldInsert(insert: Symbol, offset: Symbol, bits: Symbol): Symbol =
functionSymbol(this, insert, offset, bits, "bitfieldInsert($0)")
/**
* reverse the order of bits in an integer
* @since GLSL 4.00
*/
@JvmName("bitfieldReverseSi")
fun Symbol.bitfieldReverse(): Symbol =
functionSymbol(this, "bitfieldReverse($0)")
val Symbol.uint: Symbol
@JvmName("intSi")
get() = functionSymbol(this, "uint($0)")
val Symbol.double: Symbol
@JvmName("doubleSi")
get() = functionSymbol(this, "float($0)")
}
val Int.symbol: Symbol
get() = symbol(glsl(this)!!)
© 2015 - 2024 Weber Informatics LLC | Privacy Policy