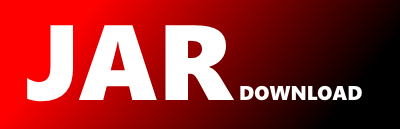
org.opensaml.saml.metadata.resolver.impl.FunctionDrivenDynamicHTTPMetadataResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opensaml-saml-impl Show documentation
Show all versions of opensaml-saml-impl Show documentation
SAML Provider Implementations
/*
* Licensed to the University Corporation for Advanced Internet Development,
* Inc. (UCAID) under one or more contributor license agreements. See the
* NOTICE file distributed with this work for additional information regarding
* copyright ownership. The UCAID licenses this file to You under the Apache
* License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.opensaml.saml.metadata.resolver.impl;
import java.util.Timer;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import net.shibboleth.utilities.java.support.component.ComponentSupport;
import net.shibboleth.utilities.java.support.logic.Constraint;
import net.shibboleth.utilities.java.support.primitive.StringSupport;
import net.shibboleth.utilities.java.support.resolver.CriteriaSet;
import org.apache.http.client.HttpClient;
import org.opensaml.core.criterion.EntityIdCriterion;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Function;
/**
* Simple implementation of an HTTP-based dynamic metadata resolver which builds the request URL
* to process based on a {@link Function} instance.
*
*
* The function defaults to an instance of {@link HTTPEntityIDRequestURLBuilder}, thereby implementing
* the "well-known location" resolution mechanism defined in the SAML 2 metadata specification if the entity ID
* is an HTTP or HTTPS URL.
*
*/
public class FunctionDrivenDynamicHTTPMetadataResolver extends AbstractDynamicHTTPMetadataResolver {
/** Logger. */
private final Logger log = LoggerFactory.getLogger(FunctionDrivenDynamicHTTPMetadataResolver.class);
/** Function for building the request URL. */
private Function requestURLBuilder;
/**
* Constructor.
*
* @param client the instance of {@link HttpClient} used to fetch remote metadata
*/
public FunctionDrivenDynamicHTTPMetadataResolver(HttpClient client) {
this(null, client);
}
/**
* Constructor.
*
* @param backgroundTaskTimer the {@link Timer} instance used to run resolver background managment tasks
* @param client the instance of {@link HttpClient} used to fetch remote metadata
*/
public FunctionDrivenDynamicHTTPMetadataResolver(@Nullable Timer backgroundTaskTimer, @Nonnull HttpClient client) {
super(backgroundTaskTimer, client);
setRequestURLBuilder(new HTTPEntityIDRequestURLBuilder());
}
/**
* Get the function which builds the request URL.
*
* Defaults to an instance of {@link HTTPEntityIDRequestURLBuilder}.
*
* @return the request URL builder function instance
*/
@Nonnull public Function getRequestURLBuilder() {
return requestURLBuilder;
}
/**
*
* Set the function which builds the request URL.
*
* Defaults to an instance of {@link HTTPEntityIDRequestURLBuilder}.
*
* @param builder the reqeust URL builder function instance
*/
public void setRequestURLBuilder(@Nonnull final Function builder) {
ComponentSupport.ifInitializedThrowUnmodifiabledComponentException(this);
ComponentSupport.ifDestroyedThrowDestroyedComponentException(this);
requestURLBuilder = Constraint.isNotNull(builder, "Request URL builder function was null");
}
/** {@inheritDoc} */
@Nullable protected String buildRequestURL(@Nonnull CriteriaSet criteria) {
String entityID = StringSupport.trimOrNull(criteria.get(EntityIdCriterion.class).getEntityId());
if (entityID == null) {
return null;
}
String url = getRequestURLBuilder().apply(entityID);
log.debug("URL generated by request builder was: {}", url);
return url;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy