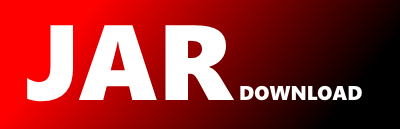
org.openscm.kundo.plugins.AbstractCoberturaDelegate.groovy Maven / Gradle / Ivy
package org.openscm.kundo.plugins
/*
* Copyright (C) 2008 The Ultimate People Company Ltd ("UPCO").
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.openscm.kundo.plugins.context.BuildContext
/**
* Abstract Cobertura Delegate
* @author Ramesh Ramakrishnan
* @version 1.0.0
*
* Description: Abstract class instantiating the required input parameters
*
*/
/*
* @ant-target doCoberturaInstrument, doCoberturaCheck
*/
abstract class AbstractCoberturaDelegate extends AbstractPluginTargetDelegate {
/*
* Property to determine if code coverage to be performed using Cobertura plugin
* @ant-property-name cobertura.enabled
*/
protected String enabled
/*
* Directory containing the generated coverage reports
* @ant-property-name cobertura.report.dir
*/
protected String reportDir
/*
* Directory containing the instrumented classes
* @ant-property-name cobertura.instrumented.dir
*/
protected String instrumentedDir
/*
* Directory containing cobertura data file
* @ant-property-name cobertura.datafile.dir
*/
protected String datafileDir
/*
* name of data file
* @ant-property-name cobertura.datafile
*/
protected String datafile
/*
* Specify the minimum acceptable branch coverage report required for each classes
* @ant-property-name cobertura.branch.rate
*/
protected String branchRate
/*
* Specify the minimum acceptable line coverage report required for each classes
* @ant-property-name cobertura.line.rate
*/
protected String lineRate
/*
* This property when set to true will fail the build if none of the coverage metrics is met by the project
* @ant-property-name cobertura.halt.on.failure
*/
protected String haltOnFailure
/*
* Specify the minimum acceptable branch coverage report for each package
* @ant-property-name cobertura.package.branch.rate
*/
protected String packageBranchRate
/*
* Specify the minimum acceptable line coverage report required for each package
* @ant-property-name cobertura.package.line.rate
*/
protected String packageLineRate
/*
* Specify the minimum acceptable branch coverage report for the whole project
* @ant-property-name cobertura.total.branch.rate
*/
protected String totalBranchRate
/*
* Specify the minimum acceptable line coverage report for the whole project
* @ant-property-name cobertura.total.line.rate
*/
protected String totalLineRate
/*
* Specify the amount of memory to be used by JVM when processing large number of classes.
* This property is used by both cobertura-instrument and cobertura-report tasks.
* @ant-property-name cobertura.max.memory
*/
protected String maxMemory
/*
* File pattern for Including files for instrument task
* @ant-property-name cobertura.instrument.include
*/
protected String instrumentInclude
/*
* File pattern for Excluding files for instrument task
* @ant-property-name cobertura.instrument.exclude
*/
protected String instrumentExclude
/*
* File pattern for Including files for cobertura report task
* @ant-property-name cobertura.report.include
*/
protected String reportInclude
/*
* File pattern for Excluding files for cobertura report task
* @ant-property-name cobertura.report.exclude
*/
protected String reportExclude
/*
* Comma separated list of format to generate Code coverage report using
* @ant-property-name cobertura.report.formats
*/
protected String reportFormats
/*
* Comma separated list of regular expression of packages to be ignored during Instrumentation
* @ant-property-name cobertura.instrument.ignore
*/
protected String instrumentIgnore
/*
* Boolean value to determine if a code coverage merge has to be performed
* @ant-property-name cobertura.perform.merge
*/
protected String performMerge
/*
* Location of coverage files to be merged with project's coverage file
* @ant-property-name cobertura.merge.datafile.location
*/
protected String mergeDatafileLocation
/*
* Name of datafile present in cobertura.merge.datafile.location
* @ant-property-name cobertura.merge.datafile
*/
protected String mergeDatafile
/**
* Constructor sets ant and buildContext instances in super class
* @param ant AntBuilder instance
* @param buildContext BuildContext instance
*/
AbstractCoberturaDelegate( AntBuilder ant, BuildContext buildContext ){
super( ant, buildContext)
}
/**
* Setter method for member variable enabled
* @param enabled
*/
void setEnabled( String enabled ){
this.enabled = enabled
}
/**
* Setter method for member variable reportDir
* @param reportDir
*/
void setReportDir( String reportDir ){
this.reportDir = reportDir
}
/**
* Setter method for member variable instrumentedDir
* @param instrumentedDir
*/
void setInstrumentedDir( String instrumentedDir ){
this.instrumentedDir = instrumentedDir
}
/**
* Setter method for member variable datafileDir
* @param datafileDir
*/
void setDatafileDir( String datafileDir ){
this.datafileDir = datafileDir
}
/**
* Setter method for member variable datafile
* @param datafile
*/
void setDatafile( String datafile ){
this.datafile = datafile
}
/**
* Setter method for member variable branchRate
* @param branchRate
*/
void setBranchRate( String branchRate ){
this.branchRate = branchRate
}
/**
* Setter method for member variable lineRate
* @param lineRate
*/
void setLineRate( String lineRate ){
this.lineRate = lineRate
}
/**
* Setter method for member variable haltOnFailure
* @param haltOnFailure
*/
void setHaltOnFailure( String haltOnFailure ){
this.haltOnFailure = haltOnFailure
}
/**
* Setter method for member variable packageBranchRate
* @param packageBranchRate
*/
void setPackageBranchRate( String packageBranchRate ){
this.packageBranchRate = packageBranchRate
}
/**
* Setter method for member variable packageLineRate
* @param packageLineRate
*/
void setPackageLineRate( String packageLineRate ){
this.packageLineRate = packageLineRate
}
/**
* Setter method for member variable totalBranchRate
* @param totalBranchRate
*/
void setTotalBranchRate( String totalBranchRate ){
this.totalBranchRate = totalBranchRate
}
/**
* Setter method for member variable totalLineRate
* @param totalLineRate
*/
void setTotalLineRate( String totalLineRate ){
this.totalLineRate = totalLineRate
}
/**
* Setter method for member variable maxMemory
* @param maxMemory
*/
void setMaxMemory( String maxMemory ){
this.maxMemory = maxMemory
}
/**
* Setter method for member variable instrumentInclude
* @param instrumentInclude
*/
void setInstrumentInclude( String instrumentInclude ){
this.instrumentInclude = instrumentInclude
}
/**
* Setter method for member variable instrumentExclude
* @param instrumentExclude
*/
void setInstrumentExclude( String instrumentExclude ){
this.instrumentExclude = instrumentExclude
}
/**
* Setter method for member variable reportInclude
* @param reportInclude
*/
void setReportInclude( String reportInclude ){
this.reportInclude = reportInclude
}
/**
* Setter method for member variable reportExclude
* @param reportExclude
*/
void setReportExclude( String reportExclude ){
this.reportExclude = reportExclude
}
/**
* Setter method for member variable reportFormats
* @param reportFormats
*/
void setReportFormats( String reportFormats ){
this.reportFormats = reportFormats
}
/**
* Setter method for member variable instrumentIgnore
* @param instrumentIgnore
*/
void setInstrumentIgnore( String instrumentIgnore ){
this.instrumentIgnore = instrumentIgnore
}
/**
* Setter method for member variable performMerge
* @param performMerge
*/
void setPerformMerge( String performMerge ){
this.performMerge = performMerge
}
/**
* Setter method for member variable mergeDatafileLocation
* @param mergeDatafileLocation
*/
void setMergeDatafileLocation( String mergeDatafileLocation ){
this.mergeDatafileLocation = mergeDatafileLocation
}
/**
* Setter method for member variable mergeDatafile
* @param mergeDatafile
*/
void setMergeDatafile( String mergeDatafile ){
this.mergeDatafile = mergeDatafile
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy