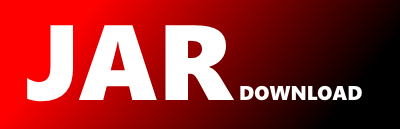
org.openscm.kundo.plugins.CoberturaCheckDelegate.groovy Maven / Gradle / Ivy
package org.openscm.kundo.plugins
/*
* Copyright (C) 2008 The Ultimate People Company Ltd ("UPCO").
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.openscm.kundo.plugins.context.BuildContext
/**
* Cobertura Check Delegate
* @author Ramesh Ramakrishnan
* @version 1.0.0
*
* Description: Perform Adherance Check on the quality metrics, generate coverage Report (supports both XML and HTML)
*
*/
/*
* @ant-target doCoberturaCheck
*/
class CoberturaCheckDelegate extends AbstractCoberturaDelegate {
/* Project related properties*/
protected Map projectProperties
/* Source Path related properties*/
protected List srcProjectProperties
/* Test Path related properties */
protected List testProjectProperties
/* cobertura check project properties */
protected List checkProjectProperties
/* A set of scopes and destination directories for dependencies to be copied to */
protected Map indexedProps
/**
* Setter method for member variable indexedProps
* @param indexedProps Map of indexed properties to be injected
*/
void setIndexedProps( Map indexedProps ){
this.indexedProps = indexedProps
}
/**
* Constructor sets ant and buildContext instances in super class
* @param ant AntBuilder instance
* @param buildContext BuildContext instance
*/
CoberturaCheckDelegate( AntBuilder ant, BuildContext buildContext ){
super( ant, buildContext)
}
/**
* Implementation of abstract method from AbstractPluginTargetDelegate
* to hold assertions that all global and local properties are available to the plugin.
* This method is automatically called prior to execution.
*/
void doCheck(){
assert enabled != null, "Error due to Null value of cobertura.enabled"
assert (enabled.equalsIgnoreCase("true") || enabled.equalsIgnoreCase("false")), "cobertura.enabled has to be a boolean value"
assert reportDir != null, "Error due to Null value of cobertura.report.dir"
assert instrumentedDir != null, "Error due to Null value of cobertura.instrumented.dir"
assert datafileDir != null, "Error due to Null value of cobertura.datafile.dir"
assert datafile != null, "Error due to Null value of cobertura.datafile"
assert branchRate != null, "Error due to Null value of cobertura.branch.rate"
assert lineRate != null, "Error due to Null value of cobertura.line.rate"
assert haltOnFailure != null, "Error due to Null value of cobertura.halt.on.failure"
assert (haltOnFailure.equalsIgnoreCase("true") || haltOnFailure.equalsIgnoreCase("false")), "cobertura.halt.on.failure has to be a boolean value"
assert packageBranchRate != null, "Error due to Null value of cobertura.package.branch.rate"
assert packageLineRate != null, "Error due to Null value of cobertura.package.line.rate"
assert totalBranchRate != null, "Error due to Null value of cobertura.total.branch.rate"
assert totalLineRate != null, "Error due to Null value of cobertura.total.line.rate"
assert maxMemory != null, "Error due to Null value of cobertura.max.memory"
assert instrumentInclude != null, "Error due to Null value of cobertura.instrument.include"
assert instrumentExclude != null, "Error due to Null value of cobertura.instrument.exclude"
assert reportFormats != null, "Error due to Null value of cobertura.report.formats"
assert reportInclude != null, "Error due to Null value of cobertura.report.include"
assert reportExclude != null, "Error due to Null value of cobertura.report.exclude"
assert indexedProps != null, "Error due to Null value of indexedProps"
assert buildContext.get( "project.properties" ) != null, "Error due to Null value of cobertura."
assert buildContext.get( "build.dir" ) != null, "global.build.dir is null"
/* Merge function assertion */
assert performMerge != null, "Error due to Null value of cobertura.perform.merge"
assert (performMerge.equalsIgnoreCase("true") || performMerge.equalsIgnoreCase("false")), "cobertura.perform.merge has to be a boolean value"
assert mergeDatafileLocation != null, "Error due to Null value of cobertura.merge.datafile.location"
assert mergeDatafile != null, "Error due to Null value of cobertura.merge.datafile"
}
/**
* Implementation of abstract method from AbstractPluginTargetDelegate
* this method is automatically called.
*/
void doExecute(){
if (enabled.equalsIgnoreCase("true")) {
/* Task definition */
ant.'taskdef'( resource:"tasks.properties", classpathref:"kundo-cobertura-plugin.dependency.path")
/* Initialise required props */
projectProperties = buildContext.get( "project.properties" )
srcProjectProperties = projectProperties.get( "src" )
testProjectProperties = projectProperties.get( "test" )
checkProjectProperties = indexedProps.get( "check" )
/* Check quality adherence */
performAdherenceCheck()
/* Generate code coverage Report*/
reportFormats.split(',').each() { format ->
generateReport(format)
}
/* Code coverage Merge */
if (performMerge.equalsIgnoreCase("true"))
coberturaMerge()
} else {
log.error ("Unable to execute cobertura check operation as cobertura is disabled")
}
}
/**
* Private method to check if Project adheres to defined quality metrics
*
* Metrics can be customised at package level.
* This is achieved by providing packageName, branchrate and linerate in the properties in the following format.
* [cobertura].[check].[index].[packageName]
* [cobertura].[check].[index].[linerate]
* [cobertura].[check].[index].[branchrate]
*
* @see http://cobertura.sourceforge.net/anttaskreference.html
*/
private void performAdherenceCheck() {
/* Load cobertura-check task attributes */
def checkOptions = [ "datafile" : datafile,
"haltonfailure" : haltOnFailure,
"branchrate" : branchRate,
"linerate" : lineRate,
"totalbranchrate" : totalBranchRate,
"totallinerate" : totalLineRate,
"packagebranchrate" : packageBranchRate,
"packagelinerate" : packageLineRate]
log.info("Performing adherance check using cobertura-check task")
ant.'cobertura-check'( checkOptions ) {
checkProjectProperties.each { checkPackageProp ->
def pkgOptions = ["pattern" : checkPackageProp.packageName,
"linerate":checkPackageProp.linerate,
"branchrate":checkPackageProp.branchrate]
ant.regex( pkgOptions )
}
}
}
/**
* Private method for generating code coverage report
* @see http://cobertura.sourceforge.net/anttaskreference.html
*/
private void generateReport(String format) {
log.info("Generating Cobertura coverage report in "+format+" format")
/* Load report generate attributes */
def reportOptions = [ "destdir":reportDir+format,
"datafile":datafile,
"format":format]
if (!maxMemory.equals(""))
reportOptions.put( "maxmemory" , maxMemory )
/* Begin report generation */
ant.'cobertura-report'( reportOptions ) {
srcProjectProperties.each() { srcProjectProperty ->
ant.fileset( dir : srcProjectProperty.dir) {
ant.include ( name : reportInclude )
ant.exclude ( name : reportExclude )
}
}
}
}
/**
* Private method for merging cobertura coverage file
* @see http://cobertura.sourceforge.net/anttaskreference.html
*/
private void coberturaMerge() {
/* Merge all the coverage files */
def mergeOptions = ["datafile":datafile]
if (!maxMemory.equals(""))
mergeOptions.put( "maxmemory" , maxMemory)
log.info("Preparing to merge Cobertura coverage file")
ant.'cobertura-merge' (mergeOptions) {
mergeDatafileLocation.split(',').each() { fileLocation ->
ant.fileset(dir:fileLocation) {
ant.include(name:mergeDatafile)
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy