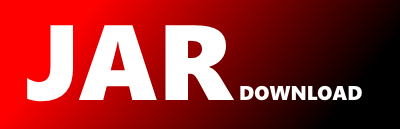
org.openscm.kundo.plugins.CoberturaInstrumentDelegate.groovy Maven / Gradle / Ivy
package org.openscm.kundo.plugins
/*
* Copyright (C) 2008 The Ultimate People Company Ltd ("UPCO").
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.openscm.kundo.plugins.context.BuildContext
/**
* Cobertura Instrument Delegate
* @author Ramesh Ramakrishnan
* @version 1.0.0
*
* Description: Perform Cobertura Instrumentation
*
*/
/*
* @ant-target doCoberturaInstrument
*/
class CoberturaInstrumentDelegate extends AbstractCoberturaDelegate {
/* Project related properties*/
protected Map projectProperties
/* Source Path related properties*/
protected List srcProjectProperties
/* Test Path related properties */
protected List testProjectProperties
/**
* Constructor sets ant and buildContext instances in super class
* @param ant AntBuilder instance
* @param buildContext BuildContext instance
*/
CoberturaInstrumentDelegate( AntBuilder ant, BuildContext buildContext ){
super( ant, buildContext)
}
/**
* Implementation of abstract method from AbstractPluginTargetDelegate
* to hold assertions that all global and local properties are available to the plugin.
* This method is automatically called prior to execution.
*/
void doCheck(){
assert enabled != null, "Error due to Null value of cobertura.enabled"
assert (enabled.equalsIgnoreCase("true") || enabled.equalsIgnoreCase("false")), "cobertura.enabled has to be a boolean value"
assert instrumentedDir != null, "Error due to Null value of cobertura.instrumented.dir"
assert datafileDir != null, "Error due to Null value of cobertura.datafile.dir"
assert datafile != null, "Error due to Null value of cobertura.datafile"
assert instrumentIgnore != null, "Error due to Null value of cobertura.instrument.ignore expression"
assert instrumentInclude != null, "Error due to Null value of cobertura.instrument.include expression"
assert maxMemory != null, "Error due to Null value of cobertura.max.memory"
assert instrumentExclude != null, "Error due to Null value of cobertura.instrument.exclude expression"
assert buildContext.get( "project.properties" ) != null, "Error due to Null value of project.properties"
assert buildContext.get( "build.dir" ) != null, "global.build.dir is null"
}
/**
* Implementation of abstract method from AbstractPluginTargetDelegate
* this method is automatically called.
*/
void doExecute(){
if (enabled.equalsIgnoreCase("true")) {
/* Task definition */
ant.'taskdef'( resource:"tasks.properties", classpathref:"kundo-cobertura-plugin.dependency.path")
/* Initialise required props */
projectProperties = buildContext.get( "project.properties" )
srcProjectProperties = projectProperties.get( "src" )
testProjectProperties = projectProperties.get( "test" )
/* Clean for a fresh start */
performClean()
/* Instrument the code */
performInstrument()
// Set up the build for the next phases
performSetup()
} else {
log.error ("Unable to execute Cobertura instrument operation as cobertura is disabled")
}
}
/**
* Private method for cleaning and creating new directory for instrumentation
*/
private void performClean() {
/* Clean up for a new start */
ant.delete( file : datafile)
ant.delete( dir : instrumentedDir)
ant.mkdir( dir : datafileDir)
}
/**
* Private method for instrumenting the code
* @see http://cobertura.sourceforge.net/anttaskreference.html
*/
private void performInstrument() {
log.info("Begin Instrumenting the source code")
def buildDir = ant.project.getProperty( "global.build.dir" )
/* load attributes */
def instrumentOptions = ["todir" : instrumentedDir,
"datafile" : datafile]
if (!maxMemory.equals(""))
instrumentOptions.put( "maxmemory" , maxMemory )
ant.'cobertura-instrument'( instrumentOptions ) {
/* Ignore source code having reference to log4j*/
instrumentIgnore.split(',').each() { ignoreExpression ->
ant.ignore(regex:ignoreExpression)
}
ant.fileset( dir:buildDir) {
ant.include ( name : instrumentInclude )
ant.exclude ( name : instrumentExclude )
}
}
}
/**
* Performs the setup in the build context/project that will configure the allow Cobertura to integrate to the test execution
*/
private void performSetup() {
// Set the sys arg's
ant.property(name : "junit.syskey.0.key" , value : "net.sourceforge.cobertura.datafile")
ant.property(name : "junit.syskey.0.file" , value : datafile )
// Set the pre-classpath
ant.path( id:"cobertura.instrumentation.dir", location:instrumentedDir )
ant.property( name:"junit.pre.classpath.id", value:"cobertura.instrumentation.dir" )
// Set the post-classpath to include the cobertura libraries.
ant.property(name:"junit.post.classpath.id", value:"kundo-cobertura-plugin.dependency.path")
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy