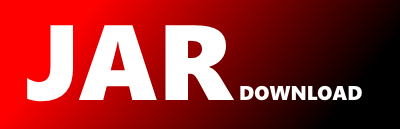
org.openscm.kundo.plugins.ReactorDelegate.groovy Maven / Gradle / Ivy
The newest version!
package org.openscm.kundo.plugins
/*
* Copyright (C) 2008 The Ultimate People Company Ltd ("UPCO").
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.openscm.kundo.plugins.context.BuildContext
import org.openscm.kundo.common.logging.SimpleLogger
import org.openscm.kundo.factory.ModuleStrategyFactory
import org.openscm.kundo.strategy.ModuleStrategy
/**
* Reactor Delegate
* @author Nigel Garner
* @version 1.0.0
*
* Description: A Reactor Delegate to manage the chaining of a build to multiple sub modules.
*
* The reactor delegate identifies an appropriate module strategy from from data within the BuildContext.
* The modules are subsequently loaded and the build is chained to the sub module in order of declaration.
*/
/*
* @ant-target doReactor
*/
class ReactorDelegate extends AbstractPluginTargetDelegate {
/** Whether the reactor build is enabled or not **/
private String disable
/** Default build target for child modules **/
private String defaultTarget
/** Profiles to be used **/
private String profiles
/** Whether the child builds will inherit the configuration of the parent **/
private String inheritConfiguration
/** Default build file for child modules **/
private String defaultBuildFile
/**
* Constructor sets ant and buildContext instances in super class
* @param ant AntBuilder instance
* @param buildContext BuildContext instance
*/
ReactorDelegate( AntBuilder ant, BuildContext buildContext ){
super( ant, buildContext)
}
/*
* The name of the default ant target to be called on the sub modules.
* @ant-property-name reactor.default.target
*/
void setDefaultTarget( String defaultTarget ){
this.defaultTarget = defaultTarget
}
/*
* The name of the default ant file to be called on the sub modules.
* @ant-property-name reactor.default.build.file
*/
void setDefaultBuildFile( String defaultBuildFile ){
this.defaultBuildFile = defaultBuildFile
}
/**
* Comma delimited list of profiles to be processed.
* See approriate ModuleStrategy implementation for profile support details.
* @ant-property-name reactor.profiles
*/
void setProfiles( String profiles ){
this.profiles = profiles
}
/*
* Whether the sub-module reactor build should inherit the configuration of the parent build.
* @ant-property-name reactor.inherit.configuration
*/
void setInheritConfiguration( String inheritConfiguration ) {
this.inheritConfiguration = inheritConfiguration
}
/*
* If true, the reactor build will be disabled. No sub-module will be called, even if they are declared.
* @ant-property-name reactor.disable
*/
void setDisable( String disable ){
this.disable = disable
}
/*
* Whether the reactor is disabled.
* @return boolean true if reactor disabled, false if reactor enabled.
*/
boolean isReactorDisabled(){
if(disable.equalsIgnoreCase( "true" )
|| disable.equalsIgnoreCase( "yes" )
|| disable.equalsIgnoreCase( "on" ) ) {
return true
}
else {
return false
}
}
/**
* Implementation of abstract method from AbstractPluginTargetDelegate
* to hold assertions that all global and local properties are available to the plugin.
* This method is automatically called prior to execution.
*/
void doCheck(){
assert disable != null
assert defaultTarget != null
assert inheritConfiguration != null
//assert buildContext.isSet( "helper.strategy" )
}
/**
* Concrete implementation of the AbstractDelegate.execute() method
*
* Obtains an appropriate ModuleStrategy and executes the build of the sub-module.
*/
void doExecute(){
// Check to see if this is a standalone build.
if ( isReactorDisabled() ){
log.info('Ignoring build reactor targets - reactor is disabled.')
return;
}
// Obtain the module strategy appropriate for this project
def msf = new ModuleStrategyFactory( ant )
ModuleStrategy modStrat = msf.getModuleStrategy( buildContext )
// Load the modules using the given Strategy.
modStrat.loadModules( buildContext, defaultBuildFile, defaultTarget , inheritConfiguration, profiles.split(',') )
def reactorTargets = findArgumentsToPropagate()
reactorTargets.each{ command_argument ->
// For each of the modules in a list, kick off a build of the sub module.
modStrat.modules.each { module ->
log.info( "Starting sub-module build for $module.name" )
if( log.isDebugEnabled() ){
log.info("module.buildFile : $module.buildFile")
log.info("module.dir : $module.dir")
log.info("module.target : $module.target")
log.info("module.inheritConfiguration : $module.inheritConfiguration")
}
/**
* TODO: need to replace this closure with our own Ant Task to call ant instead of creating a temp build file here
*/
def kundo_home = ant.project.getProperty( "env.KUNDO_HOME" )
def tempDir = ant.project.getProperty( "java.io.tmpdir" )
tempDir = "${tempDir}${File.separator}kundo${File.separator}${module.name}"
if( ! new File( "${module.dir}${File.separator}${module.buildFile}" ).exists() ){
ant.mkdir( dir : "$tempDir" )
ant.copy( file : "${kundo_home}${File.separator}conf${File.separator}kundo.build",
tofile : "${tempDir}${File.separator}${module.name}.build" ){
ant.filterset( begintoken : "name=\"", endtoken : "\"" ){
ant.filter( token : "kundo-build" , value : "name=\"${module.name}\"")
}
}
module.buildFile = "${tempDir}${File.separator}${module.name}.build"
}
/**
* This else statement copies a default build file and then adds the submodule build
* file as an import. This would be very handy as you don't need all of the Kundo guff
* in your build file, but it is not a backwardly compatible feature. Will be added in
* a later release, please don't delete it.
*
* This line also needs adding after the else outside of the test and removing from above
* module.buildFile = "${tempDir}${File.separator}${module.name}.build"
*/
/*else{
ant.mkdir( dir : "$tempDir" )
ant.copy( file : "${kundo_home}${File.separator}conf${File.separator}kundo.build",
tofile : "${tempDir}${File.separator}${module.name}.build",
overwrite : "true" ){
ant.filterset( begintoken : "name=\"", endtoken : "\"" ){
ant.filter( token : "kundo-build" , value : "name=\"${module.name}\"")
}
}
ant.replace( file : "${tempDir}${File.separator}${module.name}.build",
token : '',
value : " \n")
}*/
ant.ant( antfile: module.buildFile,
dir: module.dir,
target: command_argument,
inheritAll: module.inheritConfiguration){
if( buildContext.isSet( "helper.strategy" ) ){
ant.property( name : "global.helper.strategy", value : buildContext.get( "helper.strategy" ) )
}
}
log.info( "Finished sub-module build for $module.name" )
}
}
// Log a simple message to improve the user experience and testing..
if(modStrat.modules.isEmpty()) log.info( 'No modules available to build.' )
}
List findArgumentsToPropagate(){
def commands = ant.project.getProperty( "env.ANT_COMMAND_ARGUMENTS" )
def commandArray = commands.split( " " )
def minusCommands = []
def targets = []
commandArray.each{ arg ->
if( arg.startsWith( "-" ) ){
minusCommands.add( arg.trim() )
}else{
if( arg.indexOf( "${File.separator}" ) == -1 ){
targets.add( arg.trim() )
}
}
}
/*def returnList = []
targets.each{ target ->
def arguments = "$target"
minusCommands.each{ mincom ->
arguments = "$arguments $mincom"
}
returnList.add( arguments )
}*/
return targets
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy