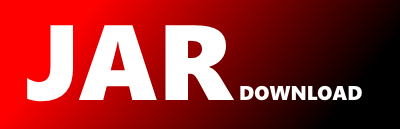
org.opensearch.migrations.replay.datahandlers.JsonAccumulator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trafficReplayer Show documentation
Show all versions of trafficReplayer Show documentation
Everything opensearch migrations
package org.opensearch.migrations.replay.datahandlers;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Deque;
import java.util.LinkedHashMap;
import java.util.Map;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.async.ByteBufferFeeder;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
/**
* Consume data, building the json object tree as it goes. This returns null until the top-level
* object or array has been built, in which case that value will be returned.
*/
@Slf4j
public class JsonAccumulator {
private final JsonParser parser;
/**
* This stack will contain JSON Objects, FieldName tokens, and ArrayLists.
* ArrayLists will be converted into arrays upon popping them from the stack.
* FieldName tokens, once bound with a child (a scalar, object or array) will
* be popped and added to the object that is situated directly above the Field
* Name in the stack.
*/
private final Deque
© 2015 - 2025 Weber Informatics LLC | Privacy Policy