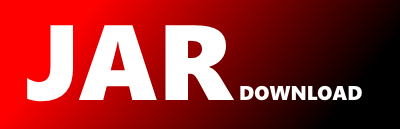
org.opensearch.migrations.replay.util.TrackedFutureJsonFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trafficReplayer Show documentation
Show all versions of trafficReplayer Show documentation
Everything opensearch migrations
package org.opensearch.migrations.replay.util;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.Lombok;
import lombok.NonNull;
import lombok.SneakyThrows;
public class TrackedFutureJsonFormatter {
static ObjectMapper objectMapper = new ObjectMapper();
private TrackedFutureJsonFormatter() {}
public static String format(TrackedFuture tf) {
return format(tf, x -> null);
}
public static String format(
TrackedFuture tf,
@NonNull Function, String> resultFormatter
) {
try {
return objectMapper.writeValueAsString(makeJson(tf, resultFormatter));
} catch (Exception e) {
throw Lombok.sneakyThrow(e);
}
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy