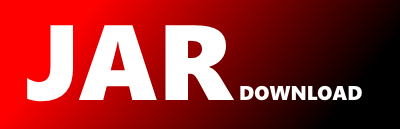
org.opensearch.test.rest.yaml.ClientYamlDocsTestClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of framework Show documentation
Show all versions of framework Show documentation
OpenSearch subproject :test:framework
/*
* SPDX-License-Identifier: Apache-2.0
*
* The OpenSearch Contributors require contributions made to
* this file be licensed under the Apache-2.0 license or a
* compatible open source license.
*/
/*
* Licensed to Elasticsearch under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/*
* Modifications Copyright OpenSearch Contributors. See
* GitHub history for details.
*/
package org.opensearch.test.rest.yaml;
import org.apache.http.HttpEntity;
import org.apache.http.HttpHost;
import org.opensearch.Version;
import org.opensearch.client.NodeSelector;
import org.opensearch.client.Request;
import org.opensearch.client.Response;
import org.opensearch.client.ResponseException;
import org.opensearch.client.RestClient;
import org.opensearch.client.RestClientBuilder;
import org.opensearch.common.CheckedSupplier;
import org.opensearch.test.rest.yaml.restspec.ClientYamlSuiteRestSpec;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* Used to execute REST requests according to the docs snippets that need to be tests. Wraps a
* {@link RestClient} instance used to send the REST requests. Holds the {@link ClientYamlSuiteRestSpec} used to translate api calls into
* REST calls. Supports raw requests besides the usual api calls based on the rest spec.
*/
public final class ClientYamlDocsTestClient extends ClientYamlTestClient {
public ClientYamlDocsTestClient(
final ClientYamlSuiteRestSpec restSpec,
final RestClient restClient,
final List hosts,
final Version esVersion,
final Version clusterManagerVersion,
final CheckedSupplier clientBuilderWithSniffedNodes
) {
super(restSpec, restClient, hosts, esVersion, clusterManagerVersion, clientBuilderWithSniffedNodes);
}
@Override
public ClientYamlTestResponse callApi(
String apiName,
Map params,
HttpEntity entity,
Map headers,
NodeSelector nodeSelector
) throws IOException {
if ("raw".equals(apiName)) {
// Raw requests don't use the rest spec at all and are configured entirely by their parameters
Map queryStringParams = new HashMap<>(params);
String method = Objects.requireNonNull(queryStringParams.remove("method"), "Method must be set to use raw request");
String path = "/" + Objects.requireNonNull(queryStringParams.remove("path"), "Path must be set to use raw request");
Request request = new Request(method, path);
// All other parameters are url parameters
for (Map.Entry param : queryStringParams.entrySet()) {
request.addParameter(param.getKey(), param.getValue());
}
request.setEntity(entity);
setOptions(request, headers);
try {
Response response = getRestClient(nodeSelector).performRequest(request);
return new ClientYamlTestResponse(response);
} catch (ResponseException e) {
throw new ClientYamlTestResponseException(e);
}
}
return super.callApi(apiName, params, entity, headers, nodeSelector);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy