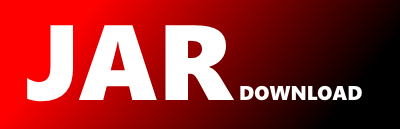
org.opensearch.common.util.CollectionUtils Maven / Gradle / Ivy
/*
* SPDX-License-Identifier: Apache-2.0
*
* The OpenSearch Contributors require contributions made to
* this file be licensed under the Apache-2.0 license or a
* compatible open source license.
*/
/*
* Licensed to Elasticsearch under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/*
* Modifications Copyright OpenSearch Contributors. See
* GitHub history for details.
*/
package org.opensearch.common.util;
import com.carrotsearch.hppc.ObjectArrayList;
import org.apache.lucene.util.BytesRef;
import org.apache.lucene.util.BytesRefArray;
import org.apache.lucene.util.BytesRefBuilder;
import org.apache.lucene.util.InPlaceMergeSorter;
import org.apache.lucene.util.IntroSorter;
import org.opensearch.common.Strings;
import org.opensearch.common.collect.Iterators;
import java.nio.file.Path;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
import java.util.RandomAccess;
import java.util.Set;
/** Collections-related utility methods. */
public class CollectionUtils {
/**
* Checks if the given array contains any elements.
*
* @param array The array to check
*
* @return false if the array contains an element, true if not or the array is null.
*/
public static boolean isEmpty(Object[] array) {
return array == null || array.length == 0;
}
/**
* Return a rotated view of the given list with the given distance.
*/
public static List rotate(final List list, int distance) {
if (list.isEmpty()) {
return list;
}
int d = distance % list.size();
if (d < 0) {
d += list.size();
}
if (d == 0) {
return list;
}
return new RotatedList<>(list, d);
}
public static void sortAndDedup(final ObjectArrayList array) {
int len = array.size();
if (len > 1) {
sort(array);
int uniqueCount = 1;
for (int i = 1; i < len; ++i) {
if (!Arrays.equals(array.get(i), array.get(i - 1))) {
array.set(uniqueCount++, array.get(i));
}
}
array.elementsCount = uniqueCount;
}
}
public static void sort(final ObjectArrayList array) {
new IntroSorter() {
byte[] pivot;
@Override
protected void swap(int i, int j) {
final byte[] tmp = array.get(i);
array.set(i, array.get(j));
array.set(j, tmp);
}
@Override
protected int compare(int i, int j) {
return compare(array.get(i), array.get(j));
}
@Override
protected void setPivot(int i) {
pivot = array.get(i);
}
@Override
protected int comparePivot(int j) {
return compare(pivot, array.get(j));
}
private int compare(byte[] left, byte[] right) {
for (int i = 0, j = 0; i < left.length && j < right.length; i++, j++) {
int a = left[i] & 0xFF;
int b = right[j] & 0xFF;
if (a != b) {
return a - b;
}
}
return left.length - right.length;
}
}.sort(0, array.size());
}
public static int[] toArray(Collection ints) {
Objects.requireNonNull(ints);
return ints.stream().mapToInt(s -> s).toArray();
}
/**
* Deeply inspects a Map, Iterable, or Object array looking for references back to itself.
* @throws IllegalArgumentException if a self-reference is found
* @param value The object to evaluate looking for self references
* @param messageHint A string to be included in the exception message if the call fails, to provide
* more context to the handler of the exception
*/
public static void ensureNoSelfReferences(Object value, String messageHint) {
Iterable> it = convert(value);
if (it != null) {
ensureNoSelfReferences(it, value, Collections.newSetFromMap(new IdentityHashMap<>()), messageHint);
}
}
private static Iterable> convert(Object value) {
if (value == null) {
return null;
}
if (value instanceof Map) {
Map, ?> map = (Map, ?>) value;
return () -> Iterators.concat(map.keySet().iterator(), map.values().iterator());
} else if ((value instanceof Iterable) && (value instanceof Path == false)) {
return (Iterable>) value;
} else if (value instanceof Object[]) {
return Arrays.asList((Object[]) value);
} else {
return null;
}
}
private static void ensureNoSelfReferences(
final Iterable> value,
Object originalReference,
final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy