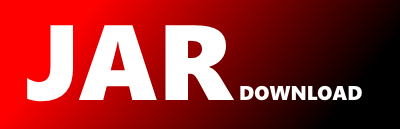
org.opensextant.annotations.DeepEyeData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opensextant-xponents-core Show documentation
Show all versions of opensextant-xponents-core Show documentation
An information extraction toolkit focused on geography and temporal entities
/*
* IIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIII
*
* OpenSextant/Xponents sub-project
* __
* ___/ /___ ___ ___ ___ __ __ ___
* / _ // -_)/ -_)/ _ \/ -_)/ // // -_)
* \_,_/ \__/ \__// .__/\__/ \_, / \__/
* /_/ /___/
*
* IIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIIII
* Copyright 2013, 2019 MITRE Corporation
*/
package org.opensextant.annotations;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import jodd.json.JsonArray;
import jodd.json.JsonObject;
/**
* A base class for Record, Annotation and other structures. Common fields include: id, value, and
* attributes, which may be empty.
*
* @author ubaldino
*/
public abstract class DeepEyeData {
/** A base class to keep all data organized */
public String id = null;
public JsonObject attrs = null;
public String value = null;
public void addAttribute(String k, Object v) {
if (attrs == null) {
this.newAttributes();
}
attrs.put(k, v);
}
public void newAttributes() {
if (this.attrs == null) {
this.attrs = new JsonObject();
}
}
/** Converts internal JSON store to a key/value map.
*/
public Map getAttributes() {
if (attrs == null) {
return null;
}
if (attrs.isEmpty()) {
return null;
}
return map(attrs);
}
public boolean isValue(Map, ?> v) {
if (v == null) {
return false;
}
return !v.isEmpty();
}
public boolean isValue(Collection> v) {
if (v == null) {
return false;
}
return !v.isEmpty();
}
/**
* utility -- get list from jsonarray.
*
* @param arr JSON array
* @return
*/
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy