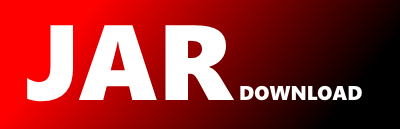
org.opensingular.app.commons.pdf.FlyingSaucerConverter Maven / Gradle / Ivy
package org.opensingular.app.commons.pdf;
import com.itextpdf.text.DocumentException;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
import org.opensingular.lib.commons.dto.HtmlToPdfDTO;
import org.opensingular.lib.commons.pdf.HtmlToPdfConverter;
import org.w3c.tidy.Configuration;
import org.w3c.tidy.Tidy;
import org.xhtmlrenderer.pdf.ITextRenderer;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.UUID;
import static org.apache.logging.log4j.util.Strings.EMPTY;
/**
* Class for converting html files into PDF using the Java only Flying Saucer library.
*/
public class FlyingSaucerConverter implements HtmlToPdfConverter {
/**
* Toggle the page counter in the right lower corner of the generated PDF pages.
*/
private boolean showPageNumber;
/**
* These fields pageLabel and ofLabel are displayed at the end of the PDF page
* in the page counter like this: "pageLabel X ofLabel Y".
* Where X is the current page and Y the total amount of pages.
* e.g. "Página 4 de 10" - by default.
* e.g. "pág. 4 / 10".
* e.g. "Page 4 of 10".
* Can be customized by it's setter methods.
*/
private String pageLabel = "Página";
private String ofLabel = "de";
public FlyingSaucerConverter() {
this.showPageNumber = true;
}
public FlyingSaucerConverter(boolean showPageNumber) {
this.showPageNumber = showPageNumber;
}
public void setShowPageNumber(boolean showPageNumber) {
this.showPageNumber = showPageNumber;
}
public void setPageLabel(String pageLabel) {
this.pageLabel = pageLabel;
}
public void setOfLabel(String ofLabel) {
this.ofLabel = ofLabel;
}
/**
* Generates the File from the HtmlToPdfDTO.
*
* @param htmlToPdfDTO the HtmlToPdfDTO.
* @return Optional of File with the PDF.
*/
@Override
public Optional convert(HtmlToPdfDTO htmlToPdfDTO) {
InputStream in = convertStream(htmlToPdfDTO);
if (in != null) {
return Optional.ofNullable(convertHtmlToPdf(in));
}
return Optional.empty();
}
/**
* Converts the html into a PDF file using Flying Saucer library via ITextRenderer.
*
* @param in InputStream with the PDF information (html).
* @return PDF File.
* @apiNote even though the Flying Saucer api does not support scripts,
* if the html contains any