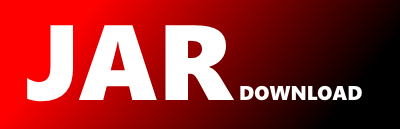
org.opensingular.lib.wicket.util.datatable.BSDataTableBuilder Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Singular Studios (a.k.a Atom Tecnologia) - www.opensingular.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.opensingular.lib.wicket.util.datatable;
import org.apache.wicket.extensions.markup.html.repeater.data.table.IColumn;
import org.apache.wicket.extensions.markup.html.repeater.data.table.ISortableDataProvider;
import org.apache.wicket.extensions.markup.html.repeater.tree.ISortableTreeProvider;
import org.apache.wicket.model.IModel;
import org.apache.wicket.model.Model;
import org.apache.wicket.model.ResourceModel;
import org.opensingular.lib.commons.lambda.IConsumer;
import org.opensingular.lib.commons.lambda.IFunction;
import org.opensingular.lib.wicket.util.datatable.column.BSActionColumn;
import org.opensingular.lib.wicket.util.datatable.column.BSPropertyActionColumn;
import org.opensingular.lib.wicket.util.datatable.column.BSPropertyColumn;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* @param Tipo de objeto que sera renderizado pelas celulas da coluna
* @param Propriedade de Ordenacao
* @param Coluna
*/
public class BSDataTableBuilder> implements Serializable {
public interface BSActionColumnCallback extends IConsumer> {
}
private final List extends IColumn> columns = new ArrayList<>();
private ISortableDataProvider dataProvider;
private ISortableTreeProvider treeProvider;
private Long rowsPerPage = null;
private boolean stripedRows = true;
private boolean hoverRows = true;
private boolean borderedTable = true;
private boolean advanceTable = false;
private boolean condensedTable = false;
private boolean showNoRecordsToolbar = true;
private IModel noRecordsMessage = new ResourceModel("datatable.no-records-found");
public BSDataTableBuilder() {
}
public BSDataTableBuilder(ISortableDataProvider dataProvider) {
setDataProvider(dataProvider);
}
public BSDataTableBuilder(ISortableTreeProvider treeProvider) {
setTreeProvider(treeProvider);
}
public static BSDataTableBuilder create() {
return new BSDataTableBuilder<>();
}
@SuppressWarnings("unchecked")
public static BSDataTableBuilder> create(Class beanType, Class sortType) {
return (BSDataTableBuilder>) create();
}
@SuppressWarnings({"unchecked", "rawtypes"})
public > BSDataTableBuilder appendColumn(C column) {
((List) columns).add(column);
return (BSDataTableBuilder) this;
}
@SuppressWarnings("unchecked")
public BSDataTableBuilder configurePreviousColumn(IConsumer columnConfig) {
columnConfig.accept((PREVCOL) columns.get(columns.size() - 1));
return this;
}
public BSDataTableBuilder> appendPropertyColumn(IModel displayModel, IFunction propertyFunction) {
return appendColumn(new BSPropertyColumn<>(displayModel, propertyFunction));
}
public BSDataTableBuilder> appendPropertyColumn(String headerTitle, IFunction propertyFunction) {
return appendPropertyColumn(Model.of(headerTitle), propertyFunction);
}
public BSDataTableBuilder> appendPropertyColumn(IModel displayModel, String propertyExpression) {
return appendColumn(new BSPropertyColumn<>(displayModel, propertyExpression));
}
public BSDataTableBuilder> appendPropertyColumn(String headerTitle, String propertyExpression) {
return appendPropertyColumn(Model.of(headerTitle), propertyExpression);
}
public BSDataTableBuilder> appendPropertyColumn(IModel displayModel, S sortProperty, IFunction propertyFunction) {
return appendColumn(new BSPropertyColumn<>(displayModel, sortProperty, propertyFunction));
}
public BSDataTableBuilder> appendPropertyColumn(String headerTitle, S sortProperty, IFunction propertyFunction) {
return appendPropertyColumn(Model.of(headerTitle), sortProperty, propertyFunction);
}
public BSDataTableBuilder> appendPropertyColumn(IModel displayModel, S sortProperty, String propertyExpression) {
return appendColumn(new BSPropertyColumn<>(displayModel, sortProperty, propertyExpression));
}
public BSDataTableBuilder> appendPropertyColumn(IModel displayModel, S sortProperty, String propertyExpression, String cssClass) {
return appendColumn(new BSPropertyColumn(displayModel, sortProperty, propertyExpression) {
@Override
public String getCssClass() {
return cssClass;
}
});
}
public BSDataTableBuilder> appendPropertyColumn(String headerTitle, S sortProperty, String propertyExpression) {
return appendPropertyColumn(Model.of(headerTitle), sortProperty, propertyExpression);
}
public BSDataTableBuilder> appendPropertyActionColumn(IModel displayModel,
IFunction propertyFunction) {
return appendColumn(new BSPropertyActionColumn<>(displayModel, propertyFunction));
}
public BSDataTableBuilder> appendActionColumn(IModel displayModel, BSActionColumnCallback callback) {
BSActionColumn column = new BSActionColumn<>(displayModel);
callback.accept(column);
return appendColumn(column);
}
public BSDataTableBuilder> appendStaticActionColumn(BSActionColumn column) {
return appendColumn(column);
}
public BSDataTableBuilder> appendActionColumn(String headerTitle, BSActionColumnCallback callback) {
return appendActionColumn(Model.of(headerTitle), callback);
}
public BSDataTableBuilder setDataProvider(ISortableDataProvider dataProvider) {
this.dataProvider = dataProvider;
return this;
}
public BSDataTableBuilder setTreeProvider(ISortableTreeProvider treeProvider) {
this.treeProvider = treeProvider;
return this;
}
public BSDataTableBuilder setStripedRows(boolean stripedRows) {
this.stripedRows = stripedRows;
return this;
}
public BSDataTableBuilder setHoverRows(boolean hoverRows) {
this.hoverRows = hoverRows;
return this;
}
public BSDataTableBuilder setAdvancedTable(boolean advanceTable) {
this.advanceTable = advanceTable;
return this;
}
public BSDataTableBuilder setBorderedTable(boolean borderedTable) {
this.borderedTable = borderedTable;
return this;
}
public BSDataTableBuilder setCondensedTable(boolean condensedTable) {
this.condensedTable = condensedTable;
return this;
}
public BSDataTableBuilder setRowsPerPage(long rowsPerPage) {
this.rowsPerPage = rowsPerPage;
return this;
}
public BSDataTableBuilder withNoRecordsToolbar() {
showNoRecordsToolbar = false;
return this;
}
public BSDataTableBuilder disablePagination() {
setRowsPerPage(Long.MAX_VALUE);
return this;
}
public IModel getNoRecordsMessage() {
return noRecordsMessage;
}
public BSDataTableBuilder setNoRecordsMessage(IModel noRecordsMessage) {
this.noRecordsMessage = noRecordsMessage;
return this;
}
public BSDataTable build(String id) {
return newDatatable(id, new ArrayList<>(columns), dataProvider)
.setRowsPerPage(rowsPerPage)
.setStripedRows(stripedRows)
.setHoverRows(hoverRows)
.setAdvanceTable(advanceTable)
.setBorderedTable(borderedTable)
.setCondensedTable(condensedTable)
.setShowNoRecordsToolbar(showNoRecordsToolbar);
}
protected BSDataTable newDatatable(String id, List extends IColumn> columns, ISortableDataProvider dataProvider) {
return new BSDataTable<>(id, new ArrayList<>(columns), dataProvider, noRecordsMessage);
}
public BSFlexDataTable buildFlex(String id) {
BSFlexDataTable table = new BSFlexDataTable<>(id, new ArrayList<>(columns), dataProvider);
table
.setRowsPerPage(rowsPerPage)
.setStripedRows(stripedRows)
.setHoverRows(hoverRows)
.setBorderedTable(borderedTable)
.setCondensedTable(condensedTable);
return table;
}
public BSTableTree buildTree(String id) {
return new BSTableTree<>(id, new ArrayList<>(columns), treeProvider)
.setRowsPerPage(rowsPerPage)
.setStripedRows(stripedRows)
.setHoverRows(hoverRows)
.setBorderedTable(borderedTable)
.setCondensedTable(condensedTable);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy