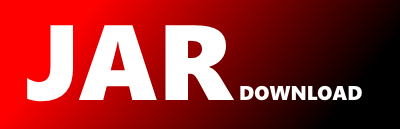
org.opensingular.lib.wicket.util.modal.OpenModalEvent Maven / Gradle / Ivy
package org.opensingular.lib.wicket.util.modal;
import java.io.Serializable;
import java.util.Optional;
import org.apache.wicket.Component;
import org.apache.wicket.ajax.AjaxRequestTarget;
import org.apache.wicket.ajax.markup.html.AjaxLink;
import org.apache.wicket.ajax.markup.html.form.AjaxButton;
import org.apache.wicket.markup.html.form.Form;
import org.apache.wicket.model.IModel;
import org.apache.wicket.model.Model;
import org.opensingular.lib.commons.lambda.IFunction;
import org.opensingular.lib.commons.lambda.IPredicate;
import org.opensingular.lib.commons.lambda.ITriConsumer;
import org.opensingular.lib.wicket.util.modal.BSModalBorder.ButtonStyle;
import org.opensingular.lib.wicket.util.util.WicketUtils;
public class OpenModalEvent implements IOpenModalEvent {
public static interface ConfigureCallback extends Serializable {
void configure(ModalDelegate delegate);
}
protected static interface ActionComponentFactory extends Serializable {
C create(String id, ModalDelegate delegate, Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy