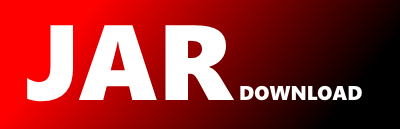
org.opensourcebim.bcf.markup.Topic Maven / Gradle / Ivy
Show all versions of bcf Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.04.12 at 08:24:55 AM CEST
//
package org.opensourcebim.bcf.markup;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for Topic complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Topic">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ReferenceLink" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Title" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Priority" type="{}Priority" minOccurs="0"/>
* <element name="Index" type="{http://www.w3.org/2001/XMLSchema}integer" minOccurs="0"/>
* <element name="Labels" type="{}TopicLabel" maxOccurs="unbounded" minOccurs="0"/>
* <element name="CreationDate" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="CreationAuthor" type="{}UserIdType"/>
* <element name="ModifiedDate" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="ModifiedAuthor" type="{}UserIdType" minOccurs="0"/>
* <element name="AssignedTo" type="{}UserIdType" minOccurs="0"/>
* <element name="Description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="BimSnippet" type="{}BimSnippet" minOccurs="0"/>
* <element name="DocumentReferences" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ReferencedDocument" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* <attGroup ref="{}DocumentReference"/>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="RelatedTopics" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="Guid" use="required" type="{}Guid" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* <attribute name="Guid" use="required" type="{}Guid" />
* <attribute name="TopicType" type="{}TopicType" />
* <attribute name="TopicStatus" type="{}TopicStatus" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Topic", propOrder = {
"referenceLink",
"title",
"priority",
"index",
"labels",
"creationDate",
"creationAuthor",
"modifiedDate",
"modifiedAuthor",
"assignedTo",
"description",
"bimSnippet",
"documentReferences",
"relatedTopics"
})
public class Topic {
@XmlElement(name = "ReferenceLink")
protected String referenceLink;
@XmlElement(name = "Title", required = true)
protected String title;
@XmlElement(name = "Priority")
protected String priority;
@XmlElement(name = "Index")
protected BigInteger index;
@XmlElement(name = "Labels")
protected List labels;
@XmlElement(name = "CreationDate", required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar creationDate;
@XmlElement(name = "CreationAuthor", required = true)
protected String creationAuthor;
@XmlElement(name = "ModifiedDate")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar modifiedDate;
@XmlElement(name = "ModifiedAuthor")
protected String modifiedAuthor;
@XmlElement(name = "AssignedTo")
protected String assignedTo;
@XmlElement(name = "Description")
protected String description;
@XmlElement(name = "BimSnippet")
protected BimSnippet bimSnippet;
@XmlElement(name = "DocumentReferences")
protected List documentReferences;
@XmlElement(name = "RelatedTopics")
protected List relatedTopics;
@XmlAttribute(name = "Guid", required = true)
protected String guid;
@XmlAttribute(name = "TopicType")
protected String topicType;
@XmlAttribute(name = "TopicStatus")
protected String topicStatus;
/**
* Gets the value of the referenceLink property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReferenceLink() {
return referenceLink;
}
/**
* Sets the value of the referenceLink property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReferenceLink(String value) {
this.referenceLink = value;
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the priority property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPriority() {
return priority;
}
/**
* Sets the value of the priority property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPriority(String value) {
this.priority = value;
}
/**
* Gets the value of the index property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getIndex() {
return index;
}
/**
* Sets the value of the index property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setIndex(BigInteger value) {
this.index = value;
}
/**
* Gets the value of the labels property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the labels property.
*
*
* For example, to add a new item, do as follows:
*
* getLabels().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getLabels() {
if (labels == null) {
labels = new ArrayList();
}
return this.labels;
}
/**
* Gets the value of the creationDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getCreationDate() {
return creationDate;
}
/**
* Sets the value of the creationDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setCreationDate(XMLGregorianCalendar value) {
this.creationDate = value;
}
/**
* Gets the value of the creationAuthor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCreationAuthor() {
return creationAuthor;
}
/**
* Sets the value of the creationAuthor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCreationAuthor(String value) {
this.creationAuthor = value;
}
/**
* Gets the value of the modifiedDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getModifiedDate() {
return modifiedDate;
}
/**
* Sets the value of the modifiedDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setModifiedDate(XMLGregorianCalendar value) {
this.modifiedDate = value;
}
/**
* Gets the value of the modifiedAuthor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getModifiedAuthor() {
return modifiedAuthor;
}
/**
* Sets the value of the modifiedAuthor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setModifiedAuthor(String value) {
this.modifiedAuthor = value;
}
/**
* Gets the value of the assignedTo property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAssignedTo() {
return assignedTo;
}
/**
* Sets the value of the assignedTo property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAssignedTo(String value) {
this.assignedTo = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the bimSnippet property.
*
* @return
* possible object is
* {@link BimSnippet }
*
*/
public BimSnippet getBimSnippet() {
return bimSnippet;
}
/**
* Sets the value of the bimSnippet property.
*
* @param value
* allowed object is
* {@link BimSnippet }
*
*/
public void setBimSnippet(BimSnippet value) {
this.bimSnippet = value;
}
/**
* Gets the value of the documentReferences property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the documentReferences property.
*
*
* For example, to add a new item, do as follows:
*
* getDocumentReferences().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Topic.DocumentReferences }
*
*
*/
public List getDocumentReferences() {
if (documentReferences == null) {
documentReferences = new ArrayList();
}
return this.documentReferences;
}
/**
* Gets the value of the relatedTopics property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relatedTopics property.
*
*
* For example, to add a new item, do as follows:
*
* getRelatedTopics().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Topic.RelatedTopics }
*
*
*/
public List getRelatedTopics() {
if (relatedTopics == null) {
relatedTopics = new ArrayList();
}
return this.relatedTopics;
}
/**
* Gets the value of the guid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGuid() {
return guid;
}
/**
* Sets the value of the guid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGuid(String value) {
this.guid = value;
}
/**
* Gets the value of the topicType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTopicType() {
return topicType;
}
/**
* Sets the value of the topicType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTopicType(String value) {
this.topicType = value;
}
/**
* Gets the value of the topicStatus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTopicStatus() {
return topicStatus;
}
/**
* Sets the value of the topicStatus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTopicStatus(String value) {
this.topicStatus = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ReferencedDocument" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* <attGroup ref="{}DocumentReference"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"referencedDocument",
"description"
})
public static class DocumentReferences {
@XmlElement(name = "ReferencedDocument")
protected String referencedDocument;
@XmlElement(name = "Description")
protected String description;
@XmlAttribute(name = "Guid")
protected String guid;
@XmlAttribute(name = "isExternal")
protected Boolean isExternal;
/**
* Gets the value of the referencedDocument property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReferencedDocument() {
return referencedDocument;
}
/**
* Sets the value of the referencedDocument property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReferencedDocument(String value) {
this.referencedDocument = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the guid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGuid() {
return guid;
}
/**
* Sets the value of the guid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGuid(String value) {
this.guid = value;
}
/**
* Gets the value of the isExternal property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public boolean isIsExternal() {
if (isExternal == null) {
return false;
} else {
return isExternal;
}
}
/**
* Sets the value of the isExternal property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsExternal(Boolean value) {
this.isExternal = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="Guid" use="required" type="{}Guid" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class RelatedTopics {
@XmlAttribute(name = "Guid", required = true)
protected String guid;
/**
* Gets the value of the guid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGuid() {
return guid;
}
/**
* Sets the value of the guid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGuid(String value) {
this.guid = value;
}
}
}