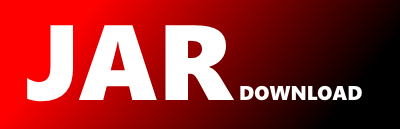
org.opensourcebim.bcf.visinfo.VisualizationInfo Maven / Gradle / Ivy
Show all versions of bcf Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.04.12 at 08:24:56 AM CEST
//
package org.opensourcebim.bcf.visinfo;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Components" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Component" type="{}Component" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="OrthogonalCamera" type="{}OrthogonalCamera" minOccurs="0"/>
* <element name="PerspectiveCamera" type="{}PerspectiveCamera" minOccurs="0"/>
* <element name="Lines" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Line" type="{}Line" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="ClippingPlanes" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ClippingPlane" type="{}ClippingPlane" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Bitmaps" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Bitmap" type="{}BitmapFormat"/>
* <element name="Reference" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Location" type="{}Point"/>
* <element name="Normal" type="{}Direction"/>
* <element name="Up" type="{}Direction"/>
* <element name="Height" type="{http://www.w3.org/2001/XMLSchema}double"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"components",
"orthogonalCamera",
"perspectiveCamera",
"lines",
"clippingPlanes",
"bitmaps"
})
@XmlRootElement(name = "VisualizationInfo")
public class VisualizationInfo {
@XmlElement(name = "Components")
protected VisualizationInfo.Components components;
@XmlElement(name = "OrthogonalCamera")
protected OrthogonalCamera orthogonalCamera;
@XmlElement(name = "PerspectiveCamera")
protected PerspectiveCamera perspectiveCamera;
@XmlElement(name = "Lines")
protected VisualizationInfo.Lines lines;
@XmlElement(name = "ClippingPlanes")
protected VisualizationInfo.ClippingPlanes clippingPlanes;
@XmlElement(name = "Bitmaps")
protected List bitmaps;
/**
* Gets the value of the components property.
*
* @return
* possible object is
* {@link VisualizationInfo.Components }
*
*/
public VisualizationInfo.Components getComponents() {
return components;
}
/**
* Sets the value of the components property.
*
* @param value
* allowed object is
* {@link VisualizationInfo.Components }
*
*/
public void setComponents(VisualizationInfo.Components value) {
this.components = value;
}
/**
* Gets the value of the orthogonalCamera property.
*
* @return
* possible object is
* {@link OrthogonalCamera }
*
*/
public OrthogonalCamera getOrthogonalCamera() {
return orthogonalCamera;
}
/**
* Sets the value of the orthogonalCamera property.
*
* @param value
* allowed object is
* {@link OrthogonalCamera }
*
*/
public void setOrthogonalCamera(OrthogonalCamera value) {
this.orthogonalCamera = value;
}
/**
* Gets the value of the perspectiveCamera property.
*
* @return
* possible object is
* {@link PerspectiveCamera }
*
*/
public PerspectiveCamera getPerspectiveCamera() {
return perspectiveCamera;
}
/**
* Sets the value of the perspectiveCamera property.
*
* @param value
* allowed object is
* {@link PerspectiveCamera }
*
*/
public void setPerspectiveCamera(PerspectiveCamera value) {
this.perspectiveCamera = value;
}
/**
* Gets the value of the lines property.
*
* @return
* possible object is
* {@link VisualizationInfo.Lines }
*
*/
public VisualizationInfo.Lines getLines() {
return lines;
}
/**
* Sets the value of the lines property.
*
* @param value
* allowed object is
* {@link VisualizationInfo.Lines }
*
*/
public void setLines(VisualizationInfo.Lines value) {
this.lines = value;
}
/**
* Gets the value of the clippingPlanes property.
*
* @return
* possible object is
* {@link VisualizationInfo.ClippingPlanes }
*
*/
public VisualizationInfo.ClippingPlanes getClippingPlanes() {
return clippingPlanes;
}
/**
* Sets the value of the clippingPlanes property.
*
* @param value
* allowed object is
* {@link VisualizationInfo.ClippingPlanes }
*
*/
public void setClippingPlanes(VisualizationInfo.ClippingPlanes value) {
this.clippingPlanes = value;
}
/**
* Gets the value of the bitmaps property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the bitmaps property.
*
*
* For example, to add a new item, do as follows:
*
* getBitmaps().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VisualizationInfo.Bitmaps }
*
*
*/
public List getBitmaps() {
if (bitmaps == null) {
bitmaps = new ArrayList();
}
return this.bitmaps;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Bitmap" type="{}BitmapFormat"/>
* <element name="Reference" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Location" type="{}Point"/>
* <element name="Normal" type="{}Direction"/>
* <element name="Up" type="{}Direction"/>
* <element name="Height" type="{http://www.w3.org/2001/XMLSchema}double"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"bitmap",
"reference",
"location",
"normal",
"up",
"height"
})
public static class Bitmaps {
@XmlElement(name = "Bitmap", required = true)
@XmlSchemaType(name = "string")
protected BitmapFormat bitmap;
@XmlElement(name = "Reference", required = true)
protected String reference;
@XmlElement(name = "Location", required = true)
protected Point location;
@XmlElement(name = "Normal", required = true)
protected Direction normal;
@XmlElement(name = "Up", required = true)
protected Direction up;
@XmlElement(name = "Height")
protected double height;
/**
* Gets the value of the bitmap property.
*
* @return
* possible object is
* {@link BitmapFormat }
*
*/
public BitmapFormat getBitmap() {
return bitmap;
}
/**
* Sets the value of the bitmap property.
*
* @param value
* allowed object is
* {@link BitmapFormat }
*
*/
public void setBitmap(BitmapFormat value) {
this.bitmap = value;
}
/**
* Gets the value of the reference property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReference() {
return reference;
}
/**
* Sets the value of the reference property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReference(String value) {
this.reference = value;
}
/**
* Gets the value of the location property.
*
* @return
* possible object is
* {@link Point }
*
*/
public Point getLocation() {
return location;
}
/**
* Sets the value of the location property.
*
* @param value
* allowed object is
* {@link Point }
*
*/
public void setLocation(Point value) {
this.location = value;
}
/**
* Gets the value of the normal property.
*
* @return
* possible object is
* {@link Direction }
*
*/
public Direction getNormal() {
return normal;
}
/**
* Sets the value of the normal property.
*
* @param value
* allowed object is
* {@link Direction }
*
*/
public void setNormal(Direction value) {
this.normal = value;
}
/**
* Gets the value of the up property.
*
* @return
* possible object is
* {@link Direction }
*
*/
public Direction getUp() {
return up;
}
/**
* Sets the value of the up property.
*
* @param value
* allowed object is
* {@link Direction }
*
*/
public void setUp(Direction value) {
this.up = value;
}
/**
* Gets the value of the height property.
*
*/
public double getHeight() {
return height;
}
/**
* Sets the value of the height property.
*
*/
public void setHeight(double value) {
this.height = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ClippingPlane" type="{}ClippingPlane" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"clippingPlane"
})
public static class ClippingPlanes {
@XmlElement(name = "ClippingPlane")
protected List clippingPlane;
/**
* Gets the value of the clippingPlane property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the clippingPlane property.
*
*
* For example, to add a new item, do as follows:
*
* getClippingPlane().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ClippingPlane }
*
*
*/
public List getClippingPlane() {
if (clippingPlane == null) {
clippingPlane = new ArrayList();
}
return this.clippingPlane;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Component" type="{}Component" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"component"
})
public static class Components {
@XmlElement(name = "Component", required = true)
protected List component;
/**
* Gets the value of the component property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the component property.
*
*
* For example, to add a new item, do as follows:
*
* getComponent().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Component }
*
*
*/
public List getComponent() {
if (component == null) {
component = new ArrayList();
}
return this.component;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Line" type="{}Line" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"line"
})
public static class Lines {
@XmlElement(name = "Line", required = true)
protected List line;
/**
* Gets the value of the line property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the line property.
*
*
* For example, to add a new item, do as follows:
*
* getLine().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Line }
*
*
*/
public List getLine() {
if (line == null) {
line = new ArrayList();
}
return this.line;
}
}
}