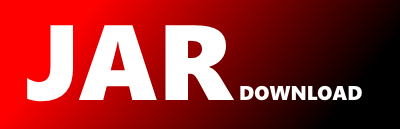
nl.wietmazairac.bimql.get.attribute.GetAttributeSubIfcShapeAspect Maven / Gradle / Ivy
package nl.wietmazairac.bimql.get.attribute;
import java.util.ArrayList;
import org.bimserver.models.ifc2x3tc1.IfcShapeAspect;
public class GetAttributeSubIfcShapeAspect {
// fields
private Object object;
private String string;
// constructors
public GetAttributeSubIfcShapeAspect(Object object, String string) {
this.object = object;
this.string = string;
}
// methods
public Object getObject() {
return object;
}
public void setObject(Object object) {
this.object = object;
}
public String getString() {
return string;
}
public void setString(String string) {
this.string = string;
}
public ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy