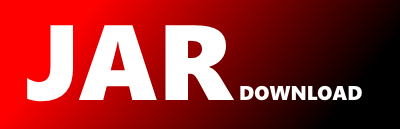
net.sourceforge.osexpress.parser.express.g Maven / Gradle / Ivy
header {
package net.sourceforge.osexpress.parser;
import java.io.*;
import java.util.Vector;
import java.util.Hashtable;
}
/**
* The Express grammar written as ANTLR productions.
* Peter Denno, [email protected]
* Stephane Lardet, [email protected]
*
* Development of this software was funded by the United States Government,
* and is not subject to copyright.
*
* DISCLAIMER: Recipients of this software assume all responsibility
* associated with its operation, modification, maintenance, and subsequent
* re-distribution.
*/
/* This grammar is the result of merging 2 grammars.
The first one is a
degenerative grammar used to record ids, the second is the actual grammar
generating the parse tree. */
class ExpressParser extends Parser;
options {
k=1;
buildAST=true;
}
tokens {
/* id types */
CONSTANT_IDENT;
ENTITY_IDENT;
FUNCTION_IDENT;
PROCEDURE_IDENT;
PARAMETER_IDENT;
SCHEMA_IDENT;
TYPE_IDENT;
VARIABLE_IDENT;
ENUMERATION_IDENT;
ATTRIBUTE_IDENT;
ENTITY_ATTR_IDENT;
TYPE_ATTR_IDENT;
ENTITY_VAR_IDENT;
TYPE_VAR_IDENT;
ENTITY_PARAM_IDENT;
TYPE_PARAM_IDENT;
/* id types Express V2 */
SUBTYPE_CONSTRAINT_ID;
/* nodes types */
ACTUAL_PARAMETER_LIST; ADD_LIKE_OP; AGGREGATE_INITIALIZER;
AGGREGATE_SOURCE; AGGREGATE_TYPE; AGGREGATION_TYPES; ALGORITHM_HEAD;
ALIAS_STMT; ARRAY_TYPE; ASSIGNMENT_STMT; BAG_TYPE; BASE_TYPE;
BINARY_TYPE; BOOLEAN_TYPE; BOUND_1; BOUND_2; BOUND_SPEC;
BUILT_IN_CONSTANT; BUILT_IN_FUNCTION; BUILT_IN_PROCEDURE; CASE_ACTION;
CASE_LABEL; CASE_STMT; COMPOUND_STMT; CONSTANT_BODY; CONSTANT_DECL;
CONSTANT_FACTOR; CONSTANT_ID; DECLARATION; DOMAIN_RULE; ELEMENT;
ENTITY_HEAD; ENTITY_DECL; ENTITY_BODY; SUBSUPER; SUPERTYPE_CONSTRAINT;
ABSTRACT_SUPERTYPE_DECLARATION; SUBTYPE_DECLARATION; EXPLICIT_ATTR;
ATTRIBUTE_DECL; ATTRIBUTE_ID; QUALIFIED_ATTRIBUTE; DERIVE_CLAUSE;
DERIVED_ATTR; INVERSE_CLAUSE; INVERSE_ATTR; UNIQUE_CLAUSE; UNIQUE_RULE;
REFERENCED_ATTRIBUTE; ENTITY_CONSTRUCTOR; ENTITY_ID;
ENUMERATION_REFERENCE; ESCAPE_STMT; EXPRESSION; FACTOR;
FORMAL_PARAMETER; ATTRIBUTE_QUALIFIER; FUNCTION_CALL; FUNCTION_DECL;
FUNCTION_HEAD; FUNCTION_ID; GENERALIZED_TYPES;
GENERAL_AGGREGATION_TYPES; GENERAL_ARRAY_TYPE; GENERAL_BAG_TYPE;
GENERAL_LIST_TYPE; GENERAL_REF; GENERAL_SET_TYPE; GENERIC_TYPE;
GROUP_QUALIFIER; IF_STMT; INCREMENT; INCREMENT_CONTROL; INDEX; INDEX_1;
INDEX_2; INDEX_QUALIFIER; INTEGER_TYPE; INTERVAL; INTERVAL_HIGH;
INTERVAL_ITEM; INTERVAL_LOW; INTERVAL_OP; LABEL; LIST_TYPE; LITERAL;
REAL_LITERAL; INTEGER_LITERAL; STRING_LITERAL;
LOCAL_DECL; LOCAL_VARIABLE; LOGICAL_EXPRESSION; LOGICAL_LITERAL;
LOGICAL_TYPE; MULTIPLICATION_LIKE_OP; NAMED_TYPES; NULL_STMT;
NUMBER_TYPE; NUMERIC_EXPRESSION; ONE_OF; PARAMETER; PARAMETER_ID;
PARAMETER_TYPE; POPULATION; PRECISION_SPEC; PRIMARY;
PROCEDURE_CALL_STMT; PROCEDURE_DECL; PROCEDURE_HEAD; PROCEDURE_ID;
QUALIFIABLE_FACTOR; QUALIFIER; QUERY_EXPRESSION; REAL_TYPE;
REFERENCE_CLAUSE; REL_OP; REL_OP_EXTENDED; REPEAT_CONTROL; REPEAT_STMT;
REPETITION; RESOURCE_OR_RENAME; RESOURCE_REF; RETURN_STMT; RULE_DECL;
RULE_HEAD; RULE_ID; SCHEMA_ID; SCHEMA_BODY; SCHEMA_DECL;
INTERFACE_SPECIFICATION; USE_CLAUSE; NAMED_TYPE_OR_RENAME; SELECTOR;
SET_TYPE; SIMPLE_EXPRESSION; SIMPLE_FACTOR; SIMPLE_TYPES; SKIP_STMT;
STMT; STRING_TYPE; SUBTYPE_CONSTRAINT; SUPERTYPE_EXPRESSION;
SUPERTYPE_FACTOR; SUPERTYPE_RULE; SUPERTYPE_TERM; SYNTAX; TERM;
TYPE_DECL; UNDERLYING_TYPE; CONSTRUCTED_TYPES; ENUMERATION_TYPE;
ENUMERATION_ID; SELECT_TYPE; TYPE_ID; TYPE_LABEL; TYPE_LABEL_ID;
UNARY_OP; UNTIL_CONTROL; VARIABLE_ID; WHERE_CLAUSE; WHILE_CONTROL;
WIDTH; WIDTH_SPEC; ENTITY_REF; TYPE_REF; ENUMERATION_REF;
ATTRIBUTE_REF; CONSTANT_REF; FUNCTION_REF; PARAMETER_REF; VARIABLE_REF;
SCHEMA_REF; TYPE_LABEL_REF; PROCEDURE_REF; SIMPLE_ID; ELSE_CLAUSE;
RENAME_ID;
/* Express amendment nodes */
ENUMERATION_ITEMS; ENUMERATION_EXTENSION;
SELECT_LIST; SELECT_EXTENSION;
REDECLARED_ATTRIBUTE;
SUBTYPE_CONSTRAINT_DECL; SUBTYPE_CONSTRAINT_HEAD; SUBTYPE_CONSTRAINT_BODY;
ABSTRACT_SUPERTYPE; TOTAL_OVER;
CONCRETE_TYPES;
GENERIC_ENTITY_TYPE;
SCHEMA_VERSION_ID;
LANGUAGE_VERSION_ID;
}
{
public Scope rootScope;
public Scope currentScope;
private Scope lastCreatedScope;
/* We keep track of scopes defined by schemas. It is useful when
multiple schemas are parsed, to deal with external elements */
private Hashtable schemas;
public boolean isFirst=true; /* is the first pass running ? */
public void newScope() {
if (isFirst) newScope1();
else newScope2();
}
public void newScope1() {
/* creates a new Scope when entering a rule defining
a scope in the grammar. */
Scope ns;
ns = new Scope(currentScope);
currentScope=ns;
lastCreatedScope.setNext(ns);
lastCreatedScope=ns;
}
public void newScope2() {
/* retrieve the scope created in the first pass when
entering the same rule.
See comments in the lexer's IDENT rule definition */
currentScope=lastCreatedScope.next;
lastCreatedScope=currentScope;
}
public void upScope() {
/* when exiting a scope */
currentScope=currentScope.parent;
}
private void newSchemaScope(String id) {
/* we record schema scopes so as to retrieve external
elements when parsing multiple schemas */
newScope();
if (isFirst) schemas.put(id,currentScope);
}
private void newEntityScope(String id) {
/* entity case: additional information is recorded to build
the entity inheritance tree */
newScope();
if (isFirst) {
currentScope.setEntity();
currentScope.parent.addEntityScope(id,currentScope);
}
}
private void addId(String id, int type) {
/* record an id in the current scope */
currentScope.addId(id,type);
}
private void addSuper(String name) {
/* add superentity in the current entity scope */
currentScope.addSuperEntity(name);
}
public void setRootScope(Scope rs) {
rootScope=rs;
currentScope=rootScope;
lastCreatedScope=rootScope;
isFirst=false;
}
public void addExternal(ExternalId ei) {
/* add an element referenced or used from another schema */
currentScope.addExternal(ei);
}
public void addAllReferenceExternals(String schema) {
/* when all elements of another schema are referenced */
currentScope.addAllReferenceExternals(schema);
}
public void addAllUseExternals(String schema) {
/* when all elements of another schema are used */
currentScope.addAllUseExternals(schema);
}
public void processExternals() {
/* after the first pass, adds external ids to schema scopes */
int i,sz;
Object[] sa;
sa=schemas.values().toArray();
sz=sa.length;
for (i=0;i'
':'
;
COMMA
options {
paraphrase = ",";
}
: ','
;
DOT
options {
paraphrase = ".";
}
: '.'
;
ASSIGN
options {
paraphrase = "=";
}
: '='
;
LT
options {
paraphrase = "<";
}
: '<'
;
GT
options {
paraphrase = ">";
}
: '>'
;
LE
options {
paraphrase = "<=";
}
: '<' '='
;
GE
options {
paraphrase = ">=";
}
: '>' '='
;
DIVSIGN
options {
paraphrase = "/";
}
: '/'
;
PLUS
options {
paraphrase = "+";
}
: '+'
;
MINUS
options {
paraphrase = "-";
}
: '-'
;
STAR
options {
paraphrase = "*";
}
: '*'
;
AT
options {
paraphrase = "@";
}
: '@'
;
WS
options {
paraphrase = "white space";
}
: ( ' '
| '\f'
| '\t'
| ( "\r\n" // Evil Dos
| "\n\r" // Unknown
| '\n' // Unix
| '\r' // Macintosh
)
{
newline();
}
)
{ $setType(Token.SKIP); }
;
LTSTAR
options {
paraphrase = "less than star";
}
: '<'
'*'
;
LTGT
options {
paraphrase = "less-than/greater-than thing";
}
: '<'
'>'
;
DOUBLESTAR
options {
paraphrase = "double star";
}
: '*'
'*'
;
DOUBLEBAR
options {
paraphrase = "double bar";
}
: '|'
'|'
;
STRING
options {
paraphrase = "a string literal";
}
:
'\''
(~'\'')*
'\''
;
protected
DIGIT
options {
paraphrase = "a digit";
}
: '0'..'9'
;
INT
options {
paraphrase = "an integer value";
}
: (DIGIT)+
;
FLOAT
options {
paraphrase = "an floating point value";
}
: '.' (DIGIT)+ (('e' | 'E') ('+' | '-')? (DIGIT)+)?
;
IDENT
options {
testLiterals = true;
paraphrase = "an identifer";
}
: ('a'..'z'|'A'..'Z'|'_') ('a'..'z'|'A'..'Z'|'_'|'0'..'9')*
{
if (!parser.isFirst) $setType(globalSearchId($getText));
}
;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy