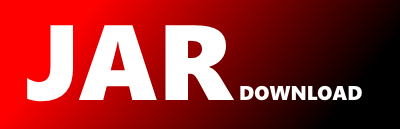
nl.tue.buildingsmart.express.population.ModelPopulation Maven / Gradle / Ivy
package nl.tue.buildingsmart.express.population;
/******************************************************************************
* Copyright (C) 2009-2016 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see {@literal }.
*****************************************************************************/
import java.io.FileInputStream;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Vector;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import nl.tue.buildingsmart.schema.EntityDefinition;
import nl.tue.buildingsmart.schema.SchemaDefinition;
@SuppressWarnings("all")
public class ModelPopulation {
private static final Logger LOGGER = LoggerFactory.getLogger(ModelPopulation.class);
private HashMap instances;
// hashmap of all instances sorted by type name;
private HashMap> typeNameMap;
private SchemaDefinition schema;
private Part21Parser parser;
private Path schemaFile;
private String entityPrefixString = "ENTITY_";
public ModelPopulation(FileInputStream input) {
parser = new Part21Parser(input);
}
public SchemaDefinition getSchema() {
return schema;
}
public void setSchema(SchemaDefinition schema) {
this.schema = schema;
if (this.parser != null)
this.parser.setSchema(schema);
}
public Path getSchemaFile() {
return schemaFile;
}
public void setSchemaFile(Path schemaFile) {
this.schemaFile = schemaFile;
parser.setSchemaFile(schemaFile);
}
public void load() {
try {
parser.setModel(this);
parser.init();
parser.syntax();
this.setInstances(parser.getInstances());
buildTypeNameMap();
} catch (ParseException e) {
// TODO Auto-generated catch block
LOGGER.error("", e);
}
}
public HashMap getInstances() {
return instances;
}
public void setInstances(HashMap instances) {
this.instances = instances;
}
public EntityInstance getEntity(Integer id) {
return instances.get(id);
}
private void buildTypeNameMap() {
typeNameMap = new HashMap>();
for (Iterator instKeyIter = instances.keySet().iterator(); instKeyIter.hasNext();) {
EntityInstance inst = instances.get(instKeyIter.next());
String typeName = inst.getEntityDefinition().getName();
if (!typeNameMap.containsKey(typeName)) {
typeNameMap.put(typeName, new Vector());
}
typeNameMap.get(typeName).add(inst);
}
}
public Vector getInstancesOfType(String typeName) {
return getInstancesOfType(typeName, false);
}
/**
* Given an (abstract) supertype recursively descends down the subtype axis
* until the first layer first layer of concrete types is found and returns
* all instances
*
* @param typeName
* the (abstract) type name
* @return a vector of EntityInstances of possible various (concrete) types
*/
public Vector getInstancesOfFirstNonAbstractTypes(String typeName) {
Vector instances = new Vector();
EntityDefinition ent = schema.getEnitiesBN().get(typeName.toUpperCase());
if (!ent.isInstantiable()) {
for (EntityDefinition subEnt : ent.getSubtypes()) {
Vector tmp = getInstancesOfFirstNonAbstractTypes(subEnt.getName());
instances.addAll(tmp);
}
} else {
Vector tmp = getInstancesOfType(ent.getName());
instances.addAll(tmp);
}
return instances;
}
public Vector getInstancesOfType(String typeName, boolean includeSubClasses) {
// get the direct instances
System.out.println("checking:" + typeName);
// typeName=typeName.toUpperCase();
Vector instances = typeNameMap.get(typeName);
if (includeSubClasses) {
// recurse into subtypes and get respective instances
EntityDefinition ent = schema.getEnitiesBN().get(typeName.toUpperCase());
for (EntityDefinition subClass : ent.getSubtypes()) {
Vector subClassInstances = getInstancesOfType(subClass.getName(), includeSubClasses);
if (subClassInstances != null) {
if (instances == null)
instances = new Vector();
instances.addAll(subClassInstances);
}
}
}
if (instances == null)
instances = new Vector();
return instances;
}
public String getEntityPrefix() {
// TODO Auto-generated method stub
return entityPrefixString;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy