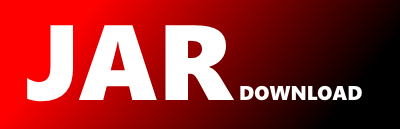
org.bimserver.models.ifc2x3tc1.IfcElement Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Element'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getTag Tag}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasStructuralMember Has Structural Member}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getFillsVoids Fills Voids}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getConnectedTo Connected To}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasCoverings Has Coverings}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasProjections Has Projections}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getReferencedInStructures Referenced In Structures}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasPorts Has Ports}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasOpenings Has Openings}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getIsConnectionRealization Is Connection Realization}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getProvidesBoundaries Provides Boundaries}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getConnectedFrom Connected From}
* - {@link org.bimserver.models.ifc2x3tc1.IfcElement#getContainedInStructure Contained In Structure}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement()
* @model
* @generated
*/
public interface IfcElement extends IfcProduct, IfcStructuralActivityAssignmentSelect {
/**
* Returns the value of the 'Tag' attribute.
*
*
* If the meaning of the 'Tag' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Tag' attribute.
* @see #isSetTag()
* @see #unsetTag()
* @see #setTag(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_Tag()
* @model unsettable="true"
* @generated
*/
String getTag();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getTag Tag}' attribute.
*
*
* @param value the new value of the 'Tag' attribute.
* @see #isSetTag()
* @see #unsetTag()
* @see #getTag()
* @generated
*/
void setTag(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getTag Tag}' attribute.
*
*
* @see #isSetTag()
* @see #getTag()
* @see #setTag(String)
* @generated
*/
void unsetTag();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getTag Tag}' attribute is set.
*
*
* @return whether the value of the 'Tag' attribute is set.
* @see #unsetTag()
* @see #getTag()
* @see #setTag(String)
* @generated
*/
boolean isSetTag();
/**
* Returns the value of the 'Has Structural Member' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsStructuralElement}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsStructuralElement#getRelatingElement Relating Element}'.
*
*
* If the meaning of the 'Has Structural Member' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Structural Member' reference list.
* @see #isSetHasStructuralMember()
* @see #unsetHasStructuralMember()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_HasStructuralMember()
* @see org.bimserver.models.ifc2x3tc1.IfcRelConnectsStructuralElement#getRelatingElement
* @model opposite="RelatingElement" unsettable="true"
* @generated
*/
EList getHasStructuralMember();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasStructuralMember Has Structural Member}' reference list.
*
*
* @see #isSetHasStructuralMember()
* @see #getHasStructuralMember()
* @generated
*/
void unsetHasStructuralMember();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasStructuralMember Has Structural Member}' reference list is set.
*
*
* @return whether the value of the 'Has Structural Member' reference list is set.
* @see #unsetHasStructuralMember()
* @see #getHasStructuralMember()
* @generated
*/
boolean isSetHasStructuralMember();
/**
* Returns the value of the 'Fills Voids' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelFillsElement}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelFillsElement#getRelatedBuildingElement Related Building Element}'.
*
*
* If the meaning of the 'Fills Voids' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Fills Voids' reference list.
* @see #isSetFillsVoids()
* @see #unsetFillsVoids()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_FillsVoids()
* @see org.bimserver.models.ifc2x3tc1.IfcRelFillsElement#getRelatedBuildingElement
* @model opposite="RelatedBuildingElement" unsettable="true" upper="2"
* @generated
*/
EList getFillsVoids();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getFillsVoids Fills Voids}' reference list.
*
*
* @see #isSetFillsVoids()
* @see #getFillsVoids()
* @generated
*/
void unsetFillsVoids();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getFillsVoids Fills Voids}' reference list is set.
*
*
* @return whether the value of the 'Fills Voids' reference list is set.
* @see #unsetFillsVoids()
* @see #getFillsVoids()
* @generated
*/
boolean isSetFillsVoids();
/**
* Returns the value of the 'Connected To' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsElements}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsElements#getRelatingElement Relating Element}'.
*
*
* If the meaning of the 'Connected To' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Connected To' reference list.
* @see #isSetConnectedTo()
* @see #unsetConnectedTo()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_ConnectedTo()
* @see org.bimserver.models.ifc2x3tc1.IfcRelConnectsElements#getRelatingElement
* @model opposite="RelatingElement" unsettable="true"
* @generated
*/
EList getConnectedTo();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getConnectedTo Connected To}' reference list.
*
*
* @see #isSetConnectedTo()
* @see #getConnectedTo()
* @generated
*/
void unsetConnectedTo();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getConnectedTo Connected To}' reference list is set.
*
*
* @return whether the value of the 'Connected To' reference list is set.
* @see #unsetConnectedTo()
* @see #getConnectedTo()
* @generated
*/
boolean isSetConnectedTo();
/**
* Returns the value of the 'Has Coverings' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelCoversBldgElements}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelCoversBldgElements#getRelatingBuildingElement Relating Building Element}'.
*
*
* If the meaning of the 'Has Coverings' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Coverings' reference list.
* @see #isSetHasCoverings()
* @see #unsetHasCoverings()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_HasCoverings()
* @see org.bimserver.models.ifc2x3tc1.IfcRelCoversBldgElements#getRelatingBuildingElement
* @model opposite="RelatingBuildingElement" unsettable="true"
* @generated
*/
EList getHasCoverings();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasCoverings Has Coverings}' reference list.
*
*
* @see #isSetHasCoverings()
* @see #getHasCoverings()
* @generated
*/
void unsetHasCoverings();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasCoverings Has Coverings}' reference list is set.
*
*
* @return whether the value of the 'Has Coverings' reference list is set.
* @see #unsetHasCoverings()
* @see #getHasCoverings()
* @generated
*/
boolean isSetHasCoverings();
/**
* Returns the value of the 'Has Projections' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelProjectsElement}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelProjectsElement#getRelatingElement Relating Element}'.
*
*
* If the meaning of the 'Has Projections' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Projections' reference list.
* @see #isSetHasProjections()
* @see #unsetHasProjections()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_HasProjections()
* @see org.bimserver.models.ifc2x3tc1.IfcRelProjectsElement#getRelatingElement
* @model opposite="RelatingElement" unsettable="true"
* @generated
*/
EList getHasProjections();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasProjections Has Projections}' reference list.
*
*
* @see #isSetHasProjections()
* @see #getHasProjections()
* @generated
*/
void unsetHasProjections();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasProjections Has Projections}' reference list is set.
*
*
* @return whether the value of the 'Has Projections' reference list is set.
* @see #unsetHasProjections()
* @see #getHasProjections()
* @generated
*/
boolean isSetHasProjections();
/**
* Returns the value of the 'Referenced In Structures' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelReferencedInSpatialStructure}.
*
*
* If the meaning of the 'Referenced In Structures' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Referenced In Structures' reference list.
* @see #isSetReferencedInStructures()
* @see #unsetReferencedInStructures()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_ReferencedInStructures()
* @model unsettable="true"
* @generated
*/
EList getReferencedInStructures();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getReferencedInStructures Referenced In Structures}' reference list.
*
*
* @see #isSetReferencedInStructures()
* @see #getReferencedInStructures()
* @generated
*/
void unsetReferencedInStructures();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getReferencedInStructures Referenced In Structures}' reference list is set.
*
*
* @return whether the value of the 'Referenced In Structures' reference list is set.
* @see #unsetReferencedInStructures()
* @see #getReferencedInStructures()
* @generated
*/
boolean isSetReferencedInStructures();
/**
* Returns the value of the 'Has Ports' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsPortToElement}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsPortToElement#getRelatedElement Related Element}'.
*
*
* If the meaning of the 'Has Ports' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Ports' reference list.
* @see #isSetHasPorts()
* @see #unsetHasPorts()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_HasPorts()
* @see org.bimserver.models.ifc2x3tc1.IfcRelConnectsPortToElement#getRelatedElement
* @model opposite="RelatedElement" unsettable="true"
* @generated
*/
EList getHasPorts();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasPorts Has Ports}' reference list.
*
*
* @see #isSetHasPorts()
* @see #getHasPorts()
* @generated
*/
void unsetHasPorts();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasPorts Has Ports}' reference list is set.
*
*
* @return whether the value of the 'Has Ports' reference list is set.
* @see #unsetHasPorts()
* @see #getHasPorts()
* @generated
*/
boolean isSetHasPorts();
/**
* Returns the value of the 'Has Openings' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelVoidsElement}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelVoidsElement#getRelatingBuildingElement Relating Building Element}'.
*
*
* If the meaning of the 'Has Openings' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Openings' reference list.
* @see #isSetHasOpenings()
* @see #unsetHasOpenings()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_HasOpenings()
* @see org.bimserver.models.ifc2x3tc1.IfcRelVoidsElement#getRelatingBuildingElement
* @model opposite="RelatingBuildingElement" unsettable="true"
* @generated
*/
EList getHasOpenings();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasOpenings Has Openings}' reference list.
*
*
* @see #isSetHasOpenings()
* @see #getHasOpenings()
* @generated
*/
void unsetHasOpenings();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getHasOpenings Has Openings}' reference list is set.
*
*
* @return whether the value of the 'Has Openings' reference list is set.
* @see #unsetHasOpenings()
* @see #getHasOpenings()
* @generated
*/
boolean isSetHasOpenings();
/**
* Returns the value of the 'Is Connection Realization' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsWithRealizingElements}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsWithRealizingElements#getRealizingElements Realizing Elements}'.
*
*
* If the meaning of the 'Is Connection Realization' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Connection Realization' reference list.
* @see #isSetIsConnectionRealization()
* @see #unsetIsConnectionRealization()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_IsConnectionRealization()
* @see org.bimserver.models.ifc2x3tc1.IfcRelConnectsWithRealizingElements#getRealizingElements
* @model opposite="RealizingElements" unsettable="true"
* @generated
*/
EList getIsConnectionRealization();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getIsConnectionRealization Is Connection Realization}' reference list.
*
*
* @see #isSetIsConnectionRealization()
* @see #getIsConnectionRealization()
* @generated
*/
void unsetIsConnectionRealization();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getIsConnectionRealization Is Connection Realization}' reference list is set.
*
*
* @return whether the value of the 'Is Connection Realization' reference list is set.
* @see #unsetIsConnectionRealization()
* @see #getIsConnectionRealization()
* @generated
*/
boolean isSetIsConnectionRealization();
/**
* Returns the value of the 'Provides Boundaries' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelSpaceBoundary}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelSpaceBoundary#getRelatedBuildingElement Related Building Element}'.
*
*
* If the meaning of the 'Provides Boundaries' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Provides Boundaries' reference list.
* @see #isSetProvidesBoundaries()
* @see #unsetProvidesBoundaries()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_ProvidesBoundaries()
* @see org.bimserver.models.ifc2x3tc1.IfcRelSpaceBoundary#getRelatedBuildingElement
* @model opposite="RelatedBuildingElement" unsettable="true"
* @generated
*/
EList getProvidesBoundaries();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getProvidesBoundaries Provides Boundaries}' reference list.
*
*
* @see #isSetProvidesBoundaries()
* @see #getProvidesBoundaries()
* @generated
*/
void unsetProvidesBoundaries();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getProvidesBoundaries Provides Boundaries}' reference list is set.
*
*
* @return whether the value of the 'Provides Boundaries' reference list is set.
* @see #unsetProvidesBoundaries()
* @see #getProvidesBoundaries()
* @generated
*/
boolean isSetProvidesBoundaries();
/**
* Returns the value of the 'Connected From' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsElements}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRelConnectsElements#getRelatedElement Related Element}'.
*
*
* If the meaning of the 'Connected From' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Connected From' reference list.
* @see #isSetConnectedFrom()
* @see #unsetConnectedFrom()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_ConnectedFrom()
* @see org.bimserver.models.ifc2x3tc1.IfcRelConnectsElements#getRelatedElement
* @model opposite="RelatedElement" unsettable="true"
* @generated
*/
EList getConnectedFrom();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getConnectedFrom Connected From}' reference list.
*
*
* @see #isSetConnectedFrom()
* @see #getConnectedFrom()
* @generated
*/
void unsetConnectedFrom();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getConnectedFrom Connected From}' reference list is set.
*
*
* @return whether the value of the 'Connected From' reference list is set.
* @see #unsetConnectedFrom()
* @see #getConnectedFrom()
* @generated
*/
boolean isSetConnectedFrom();
/**
* Returns the value of the 'Contained In Structure' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRelContainedInSpatialStructure}.
*
*
* If the meaning of the 'Contained In Structure' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Contained In Structure' reference list.
* @see #isSetContainedInStructure()
* @see #unsetContainedInStructure()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcElement_ContainedInStructure()
* @model unsettable="true" upper="2"
* @generated
*/
EList getContainedInStructure();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getContainedInStructure Contained In Structure}' reference list.
*
*
* @see #isSetContainedInStructure()
* @see #getContainedInStructure()
* @generated
*/
void unsetContainedInStructure();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcElement#getContainedInStructure Contained In Structure}' reference list is set.
*
*
* @return whether the value of the 'Contained In Structure' reference list is set.
* @see #unsetContainedInStructure()
* @see #getContainedInStructure()
* @generated
*/
boolean isSetContainedInStructure();
} // IfcElement