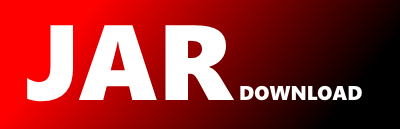
org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Geometric Representation Context'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getCoordinateSpaceDimension Coordinate Space Dimension}
* - {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecision Precision}
* - {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecisionAsString Precision As String}
* - {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getWorldCoordinateSystem World Coordinate System}
* - {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getTrueNorth True North}
* - {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getHasSubContexts Has Sub Contexts}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext()
* @model
* @generated
*/
public interface IfcGeometricRepresentationContext extends IfcRepresentationContext {
/**
* Returns the value of the 'Coordinate Space Dimension' attribute.
*
*
* If the meaning of the 'Coordinate Space Dimension' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Coordinate Space Dimension' attribute.
* @see #setCoordinateSpaceDimension(int)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext_CoordinateSpaceDimension()
* @model
* @generated
*/
int getCoordinateSpaceDimension();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getCoordinateSpaceDimension Coordinate Space Dimension}' attribute.
*
*
* @param value the new value of the 'Coordinate Space Dimension' attribute.
* @see #getCoordinateSpaceDimension()
* @generated
*/
void setCoordinateSpaceDimension(int value);
/**
* Returns the value of the 'Precision' attribute.
*
*
* If the meaning of the 'Precision' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Precision' attribute.
* @see #isSetPrecision()
* @see #unsetPrecision()
* @see #setPrecision(double)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext_Precision()
* @model unsettable="true"
* @generated
*/
double getPrecision();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecision Precision}' attribute.
*
*
* @param value the new value of the 'Precision' attribute.
* @see #isSetPrecision()
* @see #unsetPrecision()
* @see #getPrecision()
* @generated
*/
void setPrecision(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecision Precision}' attribute.
*
*
* @see #isSetPrecision()
* @see #getPrecision()
* @see #setPrecision(double)
* @generated
*/
void unsetPrecision();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecision Precision}' attribute is set.
*
*
* @return whether the value of the 'Precision' attribute is set.
* @see #unsetPrecision()
* @see #getPrecision()
* @see #setPrecision(double)
* @generated
*/
boolean isSetPrecision();
/**
* Returns the value of the 'Precision As String' attribute.
*
*
* If the meaning of the 'Precision As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Precision As String' attribute.
* @see #isSetPrecisionAsString()
* @see #unsetPrecisionAsString()
* @see #setPrecisionAsString(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext_PrecisionAsString()
* @model unsettable="true"
* @generated
*/
String getPrecisionAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecisionAsString Precision As String}' attribute.
*
*
* @param value the new value of the 'Precision As String' attribute.
* @see #isSetPrecisionAsString()
* @see #unsetPrecisionAsString()
* @see #getPrecisionAsString()
* @generated
*/
void setPrecisionAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecisionAsString Precision As String}' attribute.
*
*
* @see #isSetPrecisionAsString()
* @see #getPrecisionAsString()
* @see #setPrecisionAsString(String)
* @generated
*/
void unsetPrecisionAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getPrecisionAsString Precision As String}' attribute is set.
*
*
* @return whether the value of the 'Precision As String' attribute is set.
* @see #unsetPrecisionAsString()
* @see #getPrecisionAsString()
* @see #setPrecisionAsString(String)
* @generated
*/
boolean isSetPrecisionAsString();
/**
* Returns the value of the 'World Coordinate System' reference.
*
*
* If the meaning of the 'World Coordinate System' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'World Coordinate System' reference.
* @see #setWorldCoordinateSystem(IfcAxis2Placement)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext_WorldCoordinateSystem()
* @model
* @generated
*/
IfcAxis2Placement getWorldCoordinateSystem();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getWorldCoordinateSystem World Coordinate System}' reference.
*
*
* @param value the new value of the 'World Coordinate System' reference.
* @see #getWorldCoordinateSystem()
* @generated
*/
void setWorldCoordinateSystem(IfcAxis2Placement value);
/**
* Returns the value of the 'True North' reference.
*
*
* If the meaning of the 'True North' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'True North' reference.
* @see #isSetTrueNorth()
* @see #unsetTrueNorth()
* @see #setTrueNorth(IfcDirection)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext_TrueNorth()
* @model unsettable="true"
* @generated
*/
IfcDirection getTrueNorth();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getTrueNorth True North}' reference.
*
*
* @param value the new value of the 'True North' reference.
* @see #isSetTrueNorth()
* @see #unsetTrueNorth()
* @see #getTrueNorth()
* @generated
*/
void setTrueNorth(IfcDirection value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getTrueNorth True North}' reference.
*
*
* @see #isSetTrueNorth()
* @see #getTrueNorth()
* @see #setTrueNorth(IfcDirection)
* @generated
*/
void unsetTrueNorth();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getTrueNorth True North}' reference is set.
*
*
* @return whether the value of the 'True North' reference is set.
* @see #unsetTrueNorth()
* @see #getTrueNorth()
* @see #setTrueNorth(IfcDirection)
* @generated
*/
boolean isSetTrueNorth();
/**
* Returns the value of the 'Has Sub Contexts' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationSubContext}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationSubContext#getParentContext Parent Context}'.
*
*
* If the meaning of the 'Has Sub Contexts' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Sub Contexts' reference list.
* @see #isSetHasSubContexts()
* @see #unsetHasSubContexts()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcGeometricRepresentationContext_HasSubContexts()
* @see org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationSubContext#getParentContext
* @model opposite="ParentContext" unsettable="true"
* @generated
*/
EList getHasSubContexts();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getHasSubContexts Has Sub Contexts}' reference list.
*
*
* @see #isSetHasSubContexts()
* @see #getHasSubContexts()
* @generated
*/
void unsetHasSubContexts();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcGeometricRepresentationContext#getHasSubContexts Has Sub Contexts}' reference list is set.
*
*
* @return whether the value of the 'Has Sub Contexts' reference list is set.
* @see #unsetHasSubContexts()
* @see #getHasSubContexts()
* @generated
*/
boolean isSetHasSubContexts();
} // IfcGeometricRepresentationContext