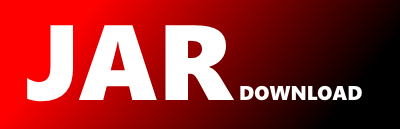
org.bimserver.models.ifc2x3tc1.IfcInventory Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Inventory'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcInventory#getInventoryType Inventory Type}
* - {@link org.bimserver.models.ifc2x3tc1.IfcInventory#getJurisdiction Jurisdiction}
* - {@link org.bimserver.models.ifc2x3tc1.IfcInventory#getResponsiblePersons Responsible Persons}
* - {@link org.bimserver.models.ifc2x3tc1.IfcInventory#getLastUpdateDate Last Update Date}
* - {@link org.bimserver.models.ifc2x3tc1.IfcInventory#getCurrentValue Current Value}
* - {@link org.bimserver.models.ifc2x3tc1.IfcInventory#getOriginalValue Original Value}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory()
* @model
* @generated
*/
public interface IfcInventory extends IfcGroup {
/**
* Returns the value of the 'Inventory Type' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcInventoryTypeEnum}.
*
*
* If the meaning of the 'Inventory Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Inventory Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcInventoryTypeEnum
* @see #setInventoryType(IfcInventoryTypeEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory_InventoryType()
* @model
* @generated
*/
IfcInventoryTypeEnum getInventoryType();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getInventoryType Inventory Type}' attribute.
*
*
* @param value the new value of the 'Inventory Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcInventoryTypeEnum
* @see #getInventoryType()
* @generated
*/
void setInventoryType(IfcInventoryTypeEnum value);
/**
* Returns the value of the 'Jurisdiction' reference.
*
*
* If the meaning of the 'Jurisdiction' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Jurisdiction' reference.
* @see #setJurisdiction(IfcActorSelect)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory_Jurisdiction()
* @model
* @generated
*/
IfcActorSelect getJurisdiction();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getJurisdiction Jurisdiction}' reference.
*
*
* @param value the new value of the 'Jurisdiction' reference.
* @see #getJurisdiction()
* @generated
*/
void setJurisdiction(IfcActorSelect value);
/**
* Returns the value of the 'Responsible Persons' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcPerson}.
*
*
* If the meaning of the 'Responsible Persons' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Responsible Persons' reference list.
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory_ResponsiblePersons()
* @model
* @generated
*/
EList getResponsiblePersons();
/**
* Returns the value of the 'Last Update Date' reference.
*
*
* If the meaning of the 'Last Update Date' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Last Update Date' reference.
* @see #setLastUpdateDate(IfcCalendarDate)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory_LastUpdateDate()
* @model
* @generated
*/
IfcCalendarDate getLastUpdateDate();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getLastUpdateDate Last Update Date}' reference.
*
*
* @param value the new value of the 'Last Update Date' reference.
* @see #getLastUpdateDate()
* @generated
*/
void setLastUpdateDate(IfcCalendarDate value);
/**
* Returns the value of the 'Current Value' reference.
*
*
* If the meaning of the 'Current Value' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Current Value' reference.
* @see #isSetCurrentValue()
* @see #unsetCurrentValue()
* @see #setCurrentValue(IfcCostValue)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory_CurrentValue()
* @model unsettable="true"
* @generated
*/
IfcCostValue getCurrentValue();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getCurrentValue Current Value}' reference.
*
*
* @param value the new value of the 'Current Value' reference.
* @see #isSetCurrentValue()
* @see #unsetCurrentValue()
* @see #getCurrentValue()
* @generated
*/
void setCurrentValue(IfcCostValue value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getCurrentValue Current Value}' reference.
*
*
* @see #isSetCurrentValue()
* @see #getCurrentValue()
* @see #setCurrentValue(IfcCostValue)
* @generated
*/
void unsetCurrentValue();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getCurrentValue Current Value}' reference is set.
*
*
* @return whether the value of the 'Current Value' reference is set.
* @see #unsetCurrentValue()
* @see #getCurrentValue()
* @see #setCurrentValue(IfcCostValue)
* @generated
*/
boolean isSetCurrentValue();
/**
* Returns the value of the 'Original Value' reference.
*
*
* If the meaning of the 'Original Value' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Original Value' reference.
* @see #isSetOriginalValue()
* @see #unsetOriginalValue()
* @see #setOriginalValue(IfcCostValue)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcInventory_OriginalValue()
* @model unsettable="true"
* @generated
*/
IfcCostValue getOriginalValue();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getOriginalValue Original Value}' reference.
*
*
* @param value the new value of the 'Original Value' reference.
* @see #isSetOriginalValue()
* @see #unsetOriginalValue()
* @see #getOriginalValue()
* @generated
*/
void setOriginalValue(IfcCostValue value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getOriginalValue Original Value}' reference.
*
*
* @see #isSetOriginalValue()
* @see #getOriginalValue()
* @see #setOriginalValue(IfcCostValue)
* @generated
*/
void unsetOriginalValue();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcInventory#getOriginalValue Original Value}' reference is set.
*
*
* @return whether the value of the 'Original Value' reference is set.
* @see #unsetOriginalValue()
* @see #getOriginalValue()
* @see #setOriginalValue(IfcCostValue)
* @generated
*/
boolean isSetOriginalValue();
} // IfcInventory