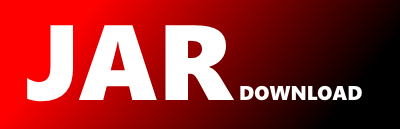
org.bimserver.models.ifc2x3tc1.IfcOrganization Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Organization'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getId Id}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getName Name}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getDescription Description}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getRoles Roles}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getAddresses Addresses}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getIsRelatedBy Is Related By}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getRelates Relates}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getEngages Engages}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization()
* @model
* @generated
*/
public interface IfcOrganization extends IfcActorSelect, IfcObjectReferenceSelect {
/**
* Returns the value of the 'Id' attribute.
*
*
* If the meaning of the 'Id' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Id' attribute.
* @see #isSetId()
* @see #unsetId()
* @see #setId(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Id()
* @model unsettable="true"
* @generated
*/
String getId();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getId Id}' attribute.
*
*
* @param value the new value of the 'Id' attribute.
* @see #isSetId()
* @see #unsetId()
* @see #getId()
* @generated
*/
void setId(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getId Id}' attribute.
*
*
* @see #isSetId()
* @see #getId()
* @see #setId(String)
* @generated
*/
void unsetId();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getId Id}' attribute is set.
*
*
* @return whether the value of the 'Id' attribute is set.
* @see #unsetId()
* @see #getId()
* @see #setId(String)
* @generated
*/
boolean isSetId();
/**
* Returns the value of the 'Name' attribute.
*
*
* If the meaning of the 'Name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Name' attribute.
* @see #setName(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Name()
* @model
* @generated
*/
String getName();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getName Name}' attribute.
*
*
* @param value the new value of the 'Name' attribute.
* @see #getName()
* @generated
*/
void setName(String value);
/**
* Returns the value of the 'Description' attribute.
*
*
* If the meaning of the 'Description' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Description' attribute.
* @see #isSetDescription()
* @see #unsetDescription()
* @see #setDescription(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Description()
* @model unsettable="true"
* @generated
*/
String getDescription();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getDescription Description}' attribute.
*
*
* @param value the new value of the 'Description' attribute.
* @see #isSetDescription()
* @see #unsetDescription()
* @see #getDescription()
* @generated
*/
void setDescription(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getDescription Description}' attribute.
*
*
* @see #isSetDescription()
* @see #getDescription()
* @see #setDescription(String)
* @generated
*/
void unsetDescription();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getDescription Description}' attribute is set.
*
*
* @return whether the value of the 'Description' attribute is set.
* @see #unsetDescription()
* @see #getDescription()
* @see #setDescription(String)
* @generated
*/
boolean isSetDescription();
/**
* Returns the value of the 'Roles' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcActorRole}.
*
*
* If the meaning of the 'Roles' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Roles' reference list.
* @see #isSetRoles()
* @see #unsetRoles()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Roles()
* @model unsettable="true"
* @generated
*/
EList getRoles();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getRoles Roles}' reference list.
*
*
* @see #isSetRoles()
* @see #getRoles()
* @generated
*/
void unsetRoles();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getRoles Roles}' reference list is set.
*
*
* @return whether the value of the 'Roles' reference list is set.
* @see #unsetRoles()
* @see #getRoles()
* @generated
*/
boolean isSetRoles();
/**
* Returns the value of the 'Addresses' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcAddress}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcAddress#getOfOrganization Of Organization}'.
*
*
* If the meaning of the 'Addresses' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Addresses' reference list.
* @see #isSetAddresses()
* @see #unsetAddresses()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Addresses()
* @see org.bimserver.models.ifc2x3tc1.IfcAddress#getOfOrganization
* @model opposite="OfOrganization" unsettable="true"
* @generated
*/
EList getAddresses();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getAddresses Addresses}' reference list.
*
*
* @see #isSetAddresses()
* @see #getAddresses()
* @generated
*/
void unsetAddresses();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getAddresses Addresses}' reference list is set.
*
*
* @return whether the value of the 'Addresses' reference list is set.
* @see #unsetAddresses()
* @see #getAddresses()
* @generated
*/
boolean isSetAddresses();
/**
* Returns the value of the 'Is Related By' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcOrganizationRelationship}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcOrganizationRelationship#getRelatedOrganizations Related Organizations}'.
*
*
* If the meaning of the 'Is Related By' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Related By' reference list.
* @see #isSetIsRelatedBy()
* @see #unsetIsRelatedBy()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_IsRelatedBy()
* @see org.bimserver.models.ifc2x3tc1.IfcOrganizationRelationship#getRelatedOrganizations
* @model opposite="RelatedOrganizations" unsettable="true"
* @generated
*/
EList getIsRelatedBy();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getIsRelatedBy Is Related By}' reference list.
*
*
* @see #isSetIsRelatedBy()
* @see #getIsRelatedBy()
* @generated
*/
void unsetIsRelatedBy();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getIsRelatedBy Is Related By}' reference list is set.
*
*
* @return whether the value of the 'Is Related By' reference list is set.
* @see #unsetIsRelatedBy()
* @see #getIsRelatedBy()
* @generated
*/
boolean isSetIsRelatedBy();
/**
* Returns the value of the 'Relates' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcOrganizationRelationship}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcOrganizationRelationship#getRelatingOrganization Relating Organization}'.
*
*
* If the meaning of the 'Relates' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Relates' reference list.
* @see #isSetRelates()
* @see #unsetRelates()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Relates()
* @see org.bimserver.models.ifc2x3tc1.IfcOrganizationRelationship#getRelatingOrganization
* @model opposite="RelatingOrganization" unsettable="true"
* @generated
*/
EList getRelates();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getRelates Relates}' reference list.
*
*
* @see #isSetRelates()
* @see #getRelates()
* @generated
*/
void unsetRelates();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getRelates Relates}' reference list is set.
*
*
* @return whether the value of the 'Relates' reference list is set.
* @see #unsetRelates()
* @see #getRelates()
* @generated
*/
boolean isSetRelates();
/**
* Returns the value of the 'Engages' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcPersonAndOrganization}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcPersonAndOrganization#getTheOrganization The Organization}'.
*
*
* If the meaning of the 'Engages' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Engages' reference list.
* @see #isSetEngages()
* @see #unsetEngages()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOrganization_Engages()
* @see org.bimserver.models.ifc2x3tc1.IfcPersonAndOrganization#getTheOrganization
* @model opposite="TheOrganization" unsettable="true"
* @generated
*/
EList getEngages();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getEngages Engages}' reference list.
*
*
* @see #isSetEngages()
* @see #getEngages()
* @generated
*/
void unsetEngages();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOrganization#getEngages Engages}' reference list is set.
*
*
* @return whether the value of the 'Engages' reference list is set.
* @see #unsetEngages()
* @see #getEngages()
* @generated
*/
boolean isSetEngages();
} // IfcOrganization