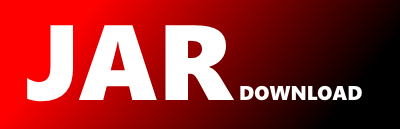
org.bimserver.models.ifc2x3tc1.IfcOwnerHistory Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.bimserver.emf.IdEObject;
/**
*
* A representation of the model object 'Ifc Owner History'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getOwningUser Owning User}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getOwningApplication Owning Application}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getState State}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getChangeAction Change Action}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifiedDate Last Modified Date}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingUser Last Modifying User}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingApplication Last Modifying Application}
* - {@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getCreationDate Creation Date}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory()
* @model
* @extends IdEObject
* @generated
*/
public interface IfcOwnerHistory extends IdEObject {
/**
* Returns the value of the 'Owning User' reference.
*
*
* If the meaning of the 'Owning User' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Owning User' reference.
* @see #setOwningUser(IfcPersonAndOrganization)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_OwningUser()
* @model
* @generated
*/
IfcPersonAndOrganization getOwningUser();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getOwningUser Owning User}' reference.
*
*
* @param value the new value of the 'Owning User' reference.
* @see #getOwningUser()
* @generated
*/
void setOwningUser(IfcPersonAndOrganization value);
/**
* Returns the value of the 'Owning Application' reference.
*
*
* If the meaning of the 'Owning Application' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Owning Application' reference.
* @see #setOwningApplication(IfcApplication)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_OwningApplication()
* @model
* @generated
*/
IfcApplication getOwningApplication();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getOwningApplication Owning Application}' reference.
*
*
* @param value the new value of the 'Owning Application' reference.
* @see #getOwningApplication()
* @generated
*/
void setOwningApplication(IfcApplication value);
/**
* Returns the value of the 'State' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcStateEnum}.
*
*
* If the meaning of the 'State' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'State' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcStateEnum
* @see #isSetState()
* @see #unsetState()
* @see #setState(IfcStateEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_State()
* @model unsettable="true"
* @generated
*/
IfcStateEnum getState();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getState State}' attribute.
*
*
* @param value the new value of the 'State' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcStateEnum
* @see #isSetState()
* @see #unsetState()
* @see #getState()
* @generated
*/
void setState(IfcStateEnum value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getState State}' attribute.
*
*
* @see #isSetState()
* @see #getState()
* @see #setState(IfcStateEnum)
* @generated
*/
void unsetState();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getState State}' attribute is set.
*
*
* @return whether the value of the 'State' attribute is set.
* @see #unsetState()
* @see #getState()
* @see #setState(IfcStateEnum)
* @generated
*/
boolean isSetState();
/**
* Returns the value of the 'Change Action' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcChangeActionEnum}.
*
*
* If the meaning of the 'Change Action' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Change Action' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcChangeActionEnum
* @see #setChangeAction(IfcChangeActionEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_ChangeAction()
* @model
* @generated
*/
IfcChangeActionEnum getChangeAction();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getChangeAction Change Action}' attribute.
*
*
* @param value the new value of the 'Change Action' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcChangeActionEnum
* @see #getChangeAction()
* @generated
*/
void setChangeAction(IfcChangeActionEnum value);
/**
* Returns the value of the 'Last Modified Date' attribute.
*
*
* If the meaning of the 'Last Modified Date' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Last Modified Date' attribute.
* @see #isSetLastModifiedDate()
* @see #unsetLastModifiedDate()
* @see #setLastModifiedDate(int)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_LastModifiedDate()
* @model unsettable="true"
* @generated
*/
int getLastModifiedDate();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifiedDate Last Modified Date}' attribute.
*
*
* @param value the new value of the 'Last Modified Date' attribute.
* @see #isSetLastModifiedDate()
* @see #unsetLastModifiedDate()
* @see #getLastModifiedDate()
* @generated
*/
void setLastModifiedDate(int value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifiedDate Last Modified Date}' attribute.
*
*
* @see #isSetLastModifiedDate()
* @see #getLastModifiedDate()
* @see #setLastModifiedDate(int)
* @generated
*/
void unsetLastModifiedDate();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifiedDate Last Modified Date}' attribute is set.
*
*
* @return whether the value of the 'Last Modified Date' attribute is set.
* @see #unsetLastModifiedDate()
* @see #getLastModifiedDate()
* @see #setLastModifiedDate(int)
* @generated
*/
boolean isSetLastModifiedDate();
/**
* Returns the value of the 'Last Modifying User' reference.
*
*
* If the meaning of the 'Last Modifying User' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Last Modifying User' reference.
* @see #isSetLastModifyingUser()
* @see #unsetLastModifyingUser()
* @see #setLastModifyingUser(IfcPersonAndOrganization)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_LastModifyingUser()
* @model unsettable="true"
* @generated
*/
IfcPersonAndOrganization getLastModifyingUser();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingUser Last Modifying User}' reference.
*
*
* @param value the new value of the 'Last Modifying User' reference.
* @see #isSetLastModifyingUser()
* @see #unsetLastModifyingUser()
* @see #getLastModifyingUser()
* @generated
*/
void setLastModifyingUser(IfcPersonAndOrganization value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingUser Last Modifying User}' reference.
*
*
* @see #isSetLastModifyingUser()
* @see #getLastModifyingUser()
* @see #setLastModifyingUser(IfcPersonAndOrganization)
* @generated
*/
void unsetLastModifyingUser();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingUser Last Modifying User}' reference is set.
*
*
* @return whether the value of the 'Last Modifying User' reference is set.
* @see #unsetLastModifyingUser()
* @see #getLastModifyingUser()
* @see #setLastModifyingUser(IfcPersonAndOrganization)
* @generated
*/
boolean isSetLastModifyingUser();
/**
* Returns the value of the 'Last Modifying Application' reference.
*
*
* If the meaning of the 'Last Modifying Application' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Last Modifying Application' reference.
* @see #isSetLastModifyingApplication()
* @see #unsetLastModifyingApplication()
* @see #setLastModifyingApplication(IfcApplication)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_LastModifyingApplication()
* @model unsettable="true"
* @generated
*/
IfcApplication getLastModifyingApplication();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingApplication Last Modifying Application}' reference.
*
*
* @param value the new value of the 'Last Modifying Application' reference.
* @see #isSetLastModifyingApplication()
* @see #unsetLastModifyingApplication()
* @see #getLastModifyingApplication()
* @generated
*/
void setLastModifyingApplication(IfcApplication value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingApplication Last Modifying Application}' reference.
*
*
* @see #isSetLastModifyingApplication()
* @see #getLastModifyingApplication()
* @see #setLastModifyingApplication(IfcApplication)
* @generated
*/
void unsetLastModifyingApplication();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getLastModifyingApplication Last Modifying Application}' reference is set.
*
*
* @return whether the value of the 'Last Modifying Application' reference is set.
* @see #unsetLastModifyingApplication()
* @see #getLastModifyingApplication()
* @see #setLastModifyingApplication(IfcApplication)
* @generated
*/
boolean isSetLastModifyingApplication();
/**
* Returns the value of the 'Creation Date' attribute.
*
*
* If the meaning of the 'Creation Date' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Creation Date' attribute.
* @see #setCreationDate(int)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcOwnerHistory_CreationDate()
* @model
* @generated
*/
int getCreationDate();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcOwnerHistory#getCreationDate Creation Date}' attribute.
*
*
* @param value the new value of the 'Creation Date' attribute.
* @see #getCreationDate()
* @generated
*/
void setCreationDate(int value);
} // IfcOwnerHistory