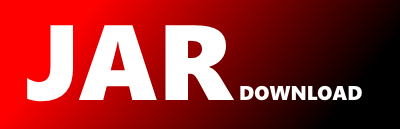
org.bimserver.models.ifc2x3tc1.IfcRepresentation Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Representation'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getContextOfItems Context Of Items}
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationIdentifier Representation Identifier}
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationType Representation Type}
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getItems Items}
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationMap Representation Map}
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getLayerAssignments Layer Assignments}
* - {@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getOfProductRepresentation Of Product Representation}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation()
* @model
* @generated
*/
public interface IfcRepresentation extends IfcLayeredItem {
/**
* Returns the value of the 'Context Of Items' reference.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentationContext#getRepresentationsInContext Representations In Context}'.
*
*
* If the meaning of the 'Context Of Items' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Context Of Items' reference.
* @see #setContextOfItems(IfcRepresentationContext)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_ContextOfItems()
* @see org.bimserver.models.ifc2x3tc1.IfcRepresentationContext#getRepresentationsInContext
* @model opposite="RepresentationsInContext"
* @generated
*/
IfcRepresentationContext getContextOfItems();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getContextOfItems Context Of Items}' reference.
*
*
* @param value the new value of the 'Context Of Items' reference.
* @see #getContextOfItems()
* @generated
*/
void setContextOfItems(IfcRepresentationContext value);
/**
* Returns the value of the 'Representation Identifier' attribute.
*
*
* If the meaning of the 'Representation Identifier' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Representation Identifier' attribute.
* @see #isSetRepresentationIdentifier()
* @see #unsetRepresentationIdentifier()
* @see #setRepresentationIdentifier(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_RepresentationIdentifier()
* @model unsettable="true"
* @generated
*/
String getRepresentationIdentifier();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationIdentifier Representation Identifier}' attribute.
*
*
* @param value the new value of the 'Representation Identifier' attribute.
* @see #isSetRepresentationIdentifier()
* @see #unsetRepresentationIdentifier()
* @see #getRepresentationIdentifier()
* @generated
*/
void setRepresentationIdentifier(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationIdentifier Representation Identifier}' attribute.
*
*
* @see #isSetRepresentationIdentifier()
* @see #getRepresentationIdentifier()
* @see #setRepresentationIdentifier(String)
* @generated
*/
void unsetRepresentationIdentifier();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationIdentifier Representation Identifier}' attribute is set.
*
*
* @return whether the value of the 'Representation Identifier' attribute is set.
* @see #unsetRepresentationIdentifier()
* @see #getRepresentationIdentifier()
* @see #setRepresentationIdentifier(String)
* @generated
*/
boolean isSetRepresentationIdentifier();
/**
* Returns the value of the 'Representation Type' attribute.
*
*
* If the meaning of the 'Representation Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Representation Type' attribute.
* @see #isSetRepresentationType()
* @see #unsetRepresentationType()
* @see #setRepresentationType(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_RepresentationType()
* @model unsettable="true"
* @generated
*/
String getRepresentationType();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationType Representation Type}' attribute.
*
*
* @param value the new value of the 'Representation Type' attribute.
* @see #isSetRepresentationType()
* @see #unsetRepresentationType()
* @see #getRepresentationType()
* @generated
*/
void setRepresentationType(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationType Representation Type}' attribute.
*
*
* @see #isSetRepresentationType()
* @see #getRepresentationType()
* @see #setRepresentationType(String)
* @generated
*/
void unsetRepresentationType();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationType Representation Type}' attribute is set.
*
*
* @return whether the value of the 'Representation Type' attribute is set.
* @see #unsetRepresentationType()
* @see #getRepresentationType()
* @see #setRepresentationType(String)
* @generated
*/
boolean isSetRepresentationType();
/**
* Returns the value of the 'Items' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRepresentationItem}.
*
*
* If the meaning of the 'Items' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Items' reference list.
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_Items()
* @model
* @generated
*/
EList getItems();
/**
* Returns the value of the 'Representation Map' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcRepresentationMap}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentationMap#getMappedRepresentation Mapped Representation}'.
*
*
* If the meaning of the 'Representation Map' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Representation Map' reference list.
* @see #isSetRepresentationMap()
* @see #unsetRepresentationMap()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_RepresentationMap()
* @see org.bimserver.models.ifc2x3tc1.IfcRepresentationMap#getMappedRepresentation
* @model opposite="MappedRepresentation" unsettable="true" upper="2"
* @generated
*/
EList getRepresentationMap();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationMap Representation Map}' reference list.
*
*
* @see #isSetRepresentationMap()
* @see #getRepresentationMap()
* @generated
*/
void unsetRepresentationMap();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getRepresentationMap Representation Map}' reference list is set.
*
*
* @return whether the value of the 'Representation Map' reference list is set.
* @see #unsetRepresentationMap()
* @see #getRepresentationMap()
* @generated
*/
boolean isSetRepresentationMap();
/**
* Returns the value of the 'Layer Assignments' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcPresentationLayerAssignment}.
*
*
* If the meaning of the 'Layer Assignments' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Layer Assignments' reference list.
* @see #isSetLayerAssignments()
* @see #unsetLayerAssignments()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_LayerAssignments()
* @model unsettable="true"
* @generated
*/
EList getLayerAssignments();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getLayerAssignments Layer Assignments}' reference list.
*
*
* @see #isSetLayerAssignments()
* @see #getLayerAssignments()
* @generated
*/
void unsetLayerAssignments();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getLayerAssignments Layer Assignments}' reference list is set.
*
*
* @return whether the value of the 'Layer Assignments' reference list is set.
* @see #unsetLayerAssignments()
* @see #getLayerAssignments()
* @generated
*/
boolean isSetLayerAssignments();
/**
* Returns the value of the 'Of Product Representation' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcProductRepresentation}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcProductRepresentation#getRepresentations Representations}'.
*
*
* If the meaning of the 'Of Product Representation' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Of Product Representation' reference list.
* @see #isSetOfProductRepresentation()
* @see #unsetOfProductRepresentation()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcRepresentation_OfProductRepresentation()
* @see org.bimserver.models.ifc2x3tc1.IfcProductRepresentation#getRepresentations
* @model opposite="Representations" unsettable="true" upper="2"
* @generated
*/
EList getOfProductRepresentation();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getOfProductRepresentation Of Product Representation}' reference list.
*
*
* @see #isSetOfProductRepresentation()
* @see #getOfProductRepresentation()
* @generated
*/
void unsetOfProductRepresentation();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcRepresentation#getOfProductRepresentation Of Product Representation}' reference list is set.
*
*
* @return whether the value of the 'Of Product Representation' reference list is set.
* @see #unsetOfProductRepresentation()
* @see #getOfProductRepresentation()
* @generated
*/
boolean isSetOfProductRepresentation();
} // IfcRepresentation