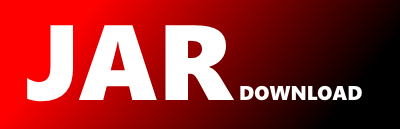
org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Structural Load Group'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getPredefinedType Predefined Type}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getActionType Action Type}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getActionSource Action Source}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficient Coefficient}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficientAsString Coefficient As String}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getPurpose Purpose}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getSourceOfResultGroup Source Of Result Group}
* - {@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getLoadGroupFor Load Group For}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup()
* @model
* @generated
*/
public interface IfcStructuralLoadGroup extends IfcGroup {
/**
* Returns the value of the 'Predefined Type' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcLoadGroupTypeEnum}.
*
*
* If the meaning of the 'Predefined Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Predefined Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcLoadGroupTypeEnum
* @see #setPredefinedType(IfcLoadGroupTypeEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_PredefinedType()
* @model
* @generated
*/
IfcLoadGroupTypeEnum getPredefinedType();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getPredefinedType Predefined Type}' attribute.
*
*
* @param value the new value of the 'Predefined Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcLoadGroupTypeEnum
* @see #getPredefinedType()
* @generated
*/
void setPredefinedType(IfcLoadGroupTypeEnum value);
/**
* Returns the value of the 'Action Type' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcActionTypeEnum}.
*
*
* If the meaning of the 'Action Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Action Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcActionTypeEnum
* @see #setActionType(IfcActionTypeEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_ActionType()
* @model
* @generated
*/
IfcActionTypeEnum getActionType();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getActionType Action Type}' attribute.
*
*
* @param value the new value of the 'Action Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcActionTypeEnum
* @see #getActionType()
* @generated
*/
void setActionType(IfcActionTypeEnum value);
/**
* Returns the value of the 'Action Source' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcActionSourceTypeEnum}.
*
*
* If the meaning of the 'Action Source' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Action Source' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcActionSourceTypeEnum
* @see #setActionSource(IfcActionSourceTypeEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_ActionSource()
* @model
* @generated
*/
IfcActionSourceTypeEnum getActionSource();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getActionSource Action Source}' attribute.
*
*
* @param value the new value of the 'Action Source' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcActionSourceTypeEnum
* @see #getActionSource()
* @generated
*/
void setActionSource(IfcActionSourceTypeEnum value);
/**
* Returns the value of the 'Coefficient' attribute.
*
*
* If the meaning of the 'Coefficient' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Coefficient' attribute.
* @see #isSetCoefficient()
* @see #unsetCoefficient()
* @see #setCoefficient(double)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_Coefficient()
* @model unsettable="true"
* @generated
*/
double getCoefficient();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficient Coefficient}' attribute.
*
*
* @param value the new value of the 'Coefficient' attribute.
* @see #isSetCoefficient()
* @see #unsetCoefficient()
* @see #getCoefficient()
* @generated
*/
void setCoefficient(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficient Coefficient}' attribute.
*
*
* @see #isSetCoefficient()
* @see #getCoefficient()
* @see #setCoefficient(double)
* @generated
*/
void unsetCoefficient();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficient Coefficient}' attribute is set.
*
*
* @return whether the value of the 'Coefficient' attribute is set.
* @see #unsetCoefficient()
* @see #getCoefficient()
* @see #setCoefficient(double)
* @generated
*/
boolean isSetCoefficient();
/**
* Returns the value of the 'Coefficient As String' attribute.
*
*
* If the meaning of the 'Coefficient As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Coefficient As String' attribute.
* @see #isSetCoefficientAsString()
* @see #unsetCoefficientAsString()
* @see #setCoefficientAsString(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_CoefficientAsString()
* @model unsettable="true"
* @generated
*/
String getCoefficientAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficientAsString Coefficient As String}' attribute.
*
*
* @param value the new value of the 'Coefficient As String' attribute.
* @see #isSetCoefficientAsString()
* @see #unsetCoefficientAsString()
* @see #getCoefficientAsString()
* @generated
*/
void setCoefficientAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficientAsString Coefficient As String}' attribute.
*
*
* @see #isSetCoefficientAsString()
* @see #getCoefficientAsString()
* @see #setCoefficientAsString(String)
* @generated
*/
void unsetCoefficientAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getCoefficientAsString Coefficient As String}' attribute is set.
*
*
* @return whether the value of the 'Coefficient As String' attribute is set.
* @see #unsetCoefficientAsString()
* @see #getCoefficientAsString()
* @see #setCoefficientAsString(String)
* @generated
*/
boolean isSetCoefficientAsString();
/**
* Returns the value of the 'Purpose' attribute.
*
*
* If the meaning of the 'Purpose' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Purpose' attribute.
* @see #isSetPurpose()
* @see #unsetPurpose()
* @see #setPurpose(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_Purpose()
* @model unsettable="true"
* @generated
*/
String getPurpose();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getPurpose Purpose}' attribute.
*
*
* @param value the new value of the 'Purpose' attribute.
* @see #isSetPurpose()
* @see #unsetPurpose()
* @see #getPurpose()
* @generated
*/
void setPurpose(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getPurpose Purpose}' attribute.
*
*
* @see #isSetPurpose()
* @see #getPurpose()
* @see #setPurpose(String)
* @generated
*/
void unsetPurpose();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getPurpose Purpose}' attribute is set.
*
*
* @return whether the value of the 'Purpose' attribute is set.
* @see #unsetPurpose()
* @see #getPurpose()
* @see #setPurpose(String)
* @generated
*/
boolean isSetPurpose();
/**
* Returns the value of the 'Source Of Result Group' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcStructuralResultGroup}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralResultGroup#getResultForLoadGroup Result For Load Group}'.
*
*
* If the meaning of the 'Source Of Result Group' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Source Of Result Group' reference list.
* @see #isSetSourceOfResultGroup()
* @see #unsetSourceOfResultGroup()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_SourceOfResultGroup()
* @see org.bimserver.models.ifc2x3tc1.IfcStructuralResultGroup#getResultForLoadGroup
* @model opposite="ResultForLoadGroup" unsettable="true" upper="2"
* @generated
*/
EList getSourceOfResultGroup();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getSourceOfResultGroup Source Of Result Group}' reference list.
*
*
* @see #isSetSourceOfResultGroup()
* @see #getSourceOfResultGroup()
* @generated
*/
void unsetSourceOfResultGroup();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getSourceOfResultGroup Source Of Result Group}' reference list is set.
*
*
* @return whether the value of the 'Source Of Result Group' reference list is set.
* @see #unsetSourceOfResultGroup()
* @see #getSourceOfResultGroup()
* @generated
*/
boolean isSetSourceOfResultGroup();
/**
* Returns the value of the 'Load Group For' reference list.
* The list contents are of type {@link org.bimserver.models.ifc2x3tc1.IfcStructuralAnalysisModel}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralAnalysisModel#getLoadedBy Loaded By}'.
*
*
* If the meaning of the 'Load Group For' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Load Group For' reference list.
* @see #isSetLoadGroupFor()
* @see #unsetLoadGroupFor()
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcStructuralLoadGroup_LoadGroupFor()
* @see org.bimserver.models.ifc2x3tc1.IfcStructuralAnalysisModel#getLoadedBy
* @model opposite="LoadedBy" unsettable="true"
* @generated
*/
EList getLoadGroupFor();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getLoadGroupFor Load Group For}' reference list.
*
*
* @see #isSetLoadGroupFor()
* @see #getLoadGroupFor()
* @generated
*/
void unsetLoadGroupFor();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcStructuralLoadGroup#getLoadGroupFor Load Group For}' reference list is set.
*
*
* @return whether the value of the 'Load Group For' reference list is set.
* @see #unsetLoadGroupFor()
* @see #getLoadGroupFor()
* @generated
*/
boolean isSetLoadGroupFor();
} // IfcStructuralLoadGroup