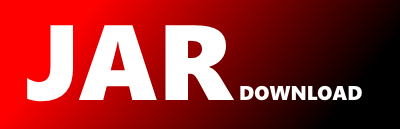
org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc2x3tc1;
/**
*
* A representation of the model object 'Ifc Window Panel Properties'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getOperationType Operation Type}
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getPanelPosition Panel Position}
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepth Frame Depth}
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepthAsString Frame Depth As String}
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThickness Frame Thickness}
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThicknessAsString Frame Thickness As String}
* - {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getShapeAspectStyle Shape Aspect Style}
*
*
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties()
* @model
* @generated
*/
public interface IfcWindowPanelProperties extends IfcPropertySetDefinition {
/**
* Returns the value of the 'Operation Type' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelOperationEnum}.
*
*
* If the meaning of the 'Operation Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Operation Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcWindowPanelOperationEnum
* @see #setOperationType(IfcWindowPanelOperationEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_OperationType()
* @model
* @generated
*/
IfcWindowPanelOperationEnum getOperationType();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getOperationType Operation Type}' attribute.
*
*
* @param value the new value of the 'Operation Type' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcWindowPanelOperationEnum
* @see #getOperationType()
* @generated
*/
void setOperationType(IfcWindowPanelOperationEnum value);
/**
* Returns the value of the 'Panel Position' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelPositionEnum}.
*
*
* If the meaning of the 'Panel Position' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Panel Position' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcWindowPanelPositionEnum
* @see #setPanelPosition(IfcWindowPanelPositionEnum)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_PanelPosition()
* @model
* @generated
*/
IfcWindowPanelPositionEnum getPanelPosition();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getPanelPosition Panel Position}' attribute.
*
*
* @param value the new value of the 'Panel Position' attribute.
* @see org.bimserver.models.ifc2x3tc1.IfcWindowPanelPositionEnum
* @see #getPanelPosition()
* @generated
*/
void setPanelPosition(IfcWindowPanelPositionEnum value);
/**
* Returns the value of the 'Frame Depth' attribute.
*
*
* If the meaning of the 'Frame Depth' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Frame Depth' attribute.
* @see #isSetFrameDepth()
* @see #unsetFrameDepth()
* @see #setFrameDepth(double)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_FrameDepth()
* @model unsettable="true"
* @generated
*/
double getFrameDepth();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepth Frame Depth}' attribute.
*
*
* @param value the new value of the 'Frame Depth' attribute.
* @see #isSetFrameDepth()
* @see #unsetFrameDepth()
* @see #getFrameDepth()
* @generated
*/
void setFrameDepth(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepth Frame Depth}' attribute.
*
*
* @see #isSetFrameDepth()
* @see #getFrameDepth()
* @see #setFrameDepth(double)
* @generated
*/
void unsetFrameDepth();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepth Frame Depth}' attribute is set.
*
*
* @return whether the value of the 'Frame Depth' attribute is set.
* @see #unsetFrameDepth()
* @see #getFrameDepth()
* @see #setFrameDepth(double)
* @generated
*/
boolean isSetFrameDepth();
/**
* Returns the value of the 'Frame Depth As String' attribute.
*
*
* If the meaning of the 'Frame Depth As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Frame Depth As String' attribute.
* @see #isSetFrameDepthAsString()
* @see #unsetFrameDepthAsString()
* @see #setFrameDepthAsString(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_FrameDepthAsString()
* @model unsettable="true"
* @generated
*/
String getFrameDepthAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepthAsString Frame Depth As String}' attribute.
*
*
* @param value the new value of the 'Frame Depth As String' attribute.
* @see #isSetFrameDepthAsString()
* @see #unsetFrameDepthAsString()
* @see #getFrameDepthAsString()
* @generated
*/
void setFrameDepthAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepthAsString Frame Depth As String}' attribute.
*
*
* @see #isSetFrameDepthAsString()
* @see #getFrameDepthAsString()
* @see #setFrameDepthAsString(String)
* @generated
*/
void unsetFrameDepthAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameDepthAsString Frame Depth As String}' attribute is set.
*
*
* @return whether the value of the 'Frame Depth As String' attribute is set.
* @see #unsetFrameDepthAsString()
* @see #getFrameDepthAsString()
* @see #setFrameDepthAsString(String)
* @generated
*/
boolean isSetFrameDepthAsString();
/**
* Returns the value of the 'Frame Thickness' attribute.
*
*
* If the meaning of the 'Frame Thickness' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Frame Thickness' attribute.
* @see #isSetFrameThickness()
* @see #unsetFrameThickness()
* @see #setFrameThickness(double)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_FrameThickness()
* @model unsettable="true"
* @generated
*/
double getFrameThickness();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThickness Frame Thickness}' attribute.
*
*
* @param value the new value of the 'Frame Thickness' attribute.
* @see #isSetFrameThickness()
* @see #unsetFrameThickness()
* @see #getFrameThickness()
* @generated
*/
void setFrameThickness(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThickness Frame Thickness}' attribute.
*
*
* @see #isSetFrameThickness()
* @see #getFrameThickness()
* @see #setFrameThickness(double)
* @generated
*/
void unsetFrameThickness();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThickness Frame Thickness}' attribute is set.
*
*
* @return whether the value of the 'Frame Thickness' attribute is set.
* @see #unsetFrameThickness()
* @see #getFrameThickness()
* @see #setFrameThickness(double)
* @generated
*/
boolean isSetFrameThickness();
/**
* Returns the value of the 'Frame Thickness As String' attribute.
*
*
* If the meaning of the 'Frame Thickness As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Frame Thickness As String' attribute.
* @see #isSetFrameThicknessAsString()
* @see #unsetFrameThicknessAsString()
* @see #setFrameThicknessAsString(String)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_FrameThicknessAsString()
* @model unsettable="true"
* @generated
*/
String getFrameThicknessAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThicknessAsString Frame Thickness As String}' attribute.
*
*
* @param value the new value of the 'Frame Thickness As String' attribute.
* @see #isSetFrameThicknessAsString()
* @see #unsetFrameThicknessAsString()
* @see #getFrameThicknessAsString()
* @generated
*/
void setFrameThicknessAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThicknessAsString Frame Thickness As String}' attribute.
*
*
* @see #isSetFrameThicknessAsString()
* @see #getFrameThicknessAsString()
* @see #setFrameThicknessAsString(String)
* @generated
*/
void unsetFrameThicknessAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getFrameThicknessAsString Frame Thickness As String}' attribute is set.
*
*
* @return whether the value of the 'Frame Thickness As String' attribute is set.
* @see #unsetFrameThicknessAsString()
* @see #getFrameThicknessAsString()
* @see #setFrameThicknessAsString(String)
* @generated
*/
boolean isSetFrameThicknessAsString();
/**
* Returns the value of the 'Shape Aspect Style' reference.
*
*
* If the meaning of the 'Shape Aspect Style' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Shape Aspect Style' reference.
* @see #isSetShapeAspectStyle()
* @see #unsetShapeAspectStyle()
* @see #setShapeAspectStyle(IfcShapeAspect)
* @see org.bimserver.models.ifc2x3tc1.Ifc2x3tc1Package#getIfcWindowPanelProperties_ShapeAspectStyle()
* @model unsettable="true"
* @generated
*/
IfcShapeAspect getShapeAspectStyle();
/**
* Sets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getShapeAspectStyle Shape Aspect Style}' reference.
*
*
* @param value the new value of the 'Shape Aspect Style' reference.
* @see #isSetShapeAspectStyle()
* @see #unsetShapeAspectStyle()
* @see #getShapeAspectStyle()
* @generated
*/
void setShapeAspectStyle(IfcShapeAspect value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getShapeAspectStyle Shape Aspect Style}' reference.
*
*
* @see #isSetShapeAspectStyle()
* @see #getShapeAspectStyle()
* @see #setShapeAspectStyle(IfcShapeAspect)
* @generated
*/
void unsetShapeAspectStyle();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc2x3tc1.IfcWindowPanelProperties#getShapeAspectStyle Shape Aspect Style}' reference is set.
*
*
* @return whether the value of the 'Shape Aspect Style' reference is set.
* @see #unsetShapeAspectStyle()
* @see #getShapeAspectStyle()
* @see #setShapeAspectStyle(IfcShapeAspect)
* @generated
*/
boolean isSetShapeAspectStyle();
} // IfcWindowPanelProperties