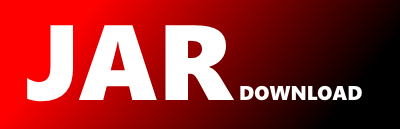
org.bimserver.models.ifc4.IfcDocumentInformation Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Document Information'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getIdentification Identification}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getName Name}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getDescription Description}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getLocation Location}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getPurpose Purpose}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getIntendedUse Intended Use}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getScope Scope}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getRevision Revision}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentOwner Document Owner}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getEditors Editors}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getCreationTime Creation Time}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getLastRevisionTime Last Revision Time}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getElectronicFormat Electronic Format}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidFrom Valid From}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidUntil Valid Until}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getConfidentiality Confidentiality}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getStatus Status}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentInfoForObjects Document Info For Objects}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getHasDocumentReferences Has Document References}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getIsPointedTo Is Pointed To}
* - {@link org.bimserver.models.ifc4.IfcDocumentInformation#getIsPointer Is Pointer}
*
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation()
* @model
* @generated
*/
public interface IfcDocumentInformation extends IfcExternalInformation, IfcDocumentSelect {
/**
* Returns the value of the 'Identification' attribute.
*
*
* If the meaning of the 'Identification' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Identification' attribute.
* @see #setIdentification(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Identification()
* @model
* @generated
*/
String getIdentification();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIdentification Identification}' attribute.
*
*
* @param value the new value of the 'Identification' attribute.
* @see #getIdentification()
* @generated
*/
void setIdentification(String value);
/**
* Returns the value of the 'Name' attribute.
*
*
* If the meaning of the 'Name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Name' attribute.
* @see #setName(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Name()
* @model
* @generated
*/
String getName();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getName Name}' attribute.
*
*
* @param value the new value of the 'Name' attribute.
* @see #getName()
* @generated
*/
void setName(String value);
/**
* Returns the value of the 'Description' attribute.
*
*
* If the meaning of the 'Description' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Description' attribute.
* @see #isSetDescription()
* @see #unsetDescription()
* @see #setDescription(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Description()
* @model unsettable="true"
* @generated
*/
String getDescription();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDescription Description}' attribute.
*
*
* @param value the new value of the 'Description' attribute.
* @see #isSetDescription()
* @see #unsetDescription()
* @see #getDescription()
* @generated
*/
void setDescription(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDescription Description}' attribute.
*
*
* @see #isSetDescription()
* @see #getDescription()
* @see #setDescription(String)
* @generated
*/
void unsetDescription();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDescription Description}' attribute is set.
*
*
* @return whether the value of the 'Description' attribute is set.
* @see #unsetDescription()
* @see #getDescription()
* @see #setDescription(String)
* @generated
*/
boolean isSetDescription();
/**
* Returns the value of the 'Location' attribute.
*
*
* If the meaning of the 'Location' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Location' attribute.
* @see #isSetLocation()
* @see #unsetLocation()
* @see #setLocation(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Location()
* @model unsettable="true"
* @generated
*/
String getLocation();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getLocation Location}' attribute.
*
*
* @param value the new value of the 'Location' attribute.
* @see #isSetLocation()
* @see #unsetLocation()
* @see #getLocation()
* @generated
*/
void setLocation(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getLocation Location}' attribute.
*
*
* @see #isSetLocation()
* @see #getLocation()
* @see #setLocation(String)
* @generated
*/
void unsetLocation();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getLocation Location}' attribute is set.
*
*
* @return whether the value of the 'Location' attribute is set.
* @see #unsetLocation()
* @see #getLocation()
* @see #setLocation(String)
* @generated
*/
boolean isSetLocation();
/**
* Returns the value of the 'Purpose' attribute.
*
*
* If the meaning of the 'Purpose' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Purpose' attribute.
* @see #isSetPurpose()
* @see #unsetPurpose()
* @see #setPurpose(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Purpose()
* @model unsettable="true"
* @generated
*/
String getPurpose();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getPurpose Purpose}' attribute.
*
*
* @param value the new value of the 'Purpose' attribute.
* @see #isSetPurpose()
* @see #unsetPurpose()
* @see #getPurpose()
* @generated
*/
void setPurpose(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getPurpose Purpose}' attribute.
*
*
* @see #isSetPurpose()
* @see #getPurpose()
* @see #setPurpose(String)
* @generated
*/
void unsetPurpose();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getPurpose Purpose}' attribute is set.
*
*
* @return whether the value of the 'Purpose' attribute is set.
* @see #unsetPurpose()
* @see #getPurpose()
* @see #setPurpose(String)
* @generated
*/
boolean isSetPurpose();
/**
* Returns the value of the 'Intended Use' attribute.
*
*
* If the meaning of the 'Intended Use' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Intended Use' attribute.
* @see #isSetIntendedUse()
* @see #unsetIntendedUse()
* @see #setIntendedUse(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_IntendedUse()
* @model unsettable="true"
* @generated
*/
String getIntendedUse();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIntendedUse Intended Use}' attribute.
*
*
* @param value the new value of the 'Intended Use' attribute.
* @see #isSetIntendedUse()
* @see #unsetIntendedUse()
* @see #getIntendedUse()
* @generated
*/
void setIntendedUse(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIntendedUse Intended Use}' attribute.
*
*
* @see #isSetIntendedUse()
* @see #getIntendedUse()
* @see #setIntendedUse(String)
* @generated
*/
void unsetIntendedUse();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIntendedUse Intended Use}' attribute is set.
*
*
* @return whether the value of the 'Intended Use' attribute is set.
* @see #unsetIntendedUse()
* @see #getIntendedUse()
* @see #setIntendedUse(String)
* @generated
*/
boolean isSetIntendedUse();
/**
* Returns the value of the 'Scope' attribute.
*
*
* If the meaning of the 'Scope' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Scope' attribute.
* @see #isSetScope()
* @see #unsetScope()
* @see #setScope(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Scope()
* @model unsettable="true"
* @generated
*/
String getScope();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getScope Scope}' attribute.
*
*
* @param value the new value of the 'Scope' attribute.
* @see #isSetScope()
* @see #unsetScope()
* @see #getScope()
* @generated
*/
void setScope(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getScope Scope}' attribute.
*
*
* @see #isSetScope()
* @see #getScope()
* @see #setScope(String)
* @generated
*/
void unsetScope();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getScope Scope}' attribute is set.
*
*
* @return whether the value of the 'Scope' attribute is set.
* @see #unsetScope()
* @see #getScope()
* @see #setScope(String)
* @generated
*/
boolean isSetScope();
/**
* Returns the value of the 'Revision' attribute.
*
*
* If the meaning of the 'Revision' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Revision' attribute.
* @see #isSetRevision()
* @see #unsetRevision()
* @see #setRevision(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Revision()
* @model unsettable="true"
* @generated
*/
String getRevision();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getRevision Revision}' attribute.
*
*
* @param value the new value of the 'Revision' attribute.
* @see #isSetRevision()
* @see #unsetRevision()
* @see #getRevision()
* @generated
*/
void setRevision(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getRevision Revision}' attribute.
*
*
* @see #isSetRevision()
* @see #getRevision()
* @see #setRevision(String)
* @generated
*/
void unsetRevision();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getRevision Revision}' attribute is set.
*
*
* @return whether the value of the 'Revision' attribute is set.
* @see #unsetRevision()
* @see #getRevision()
* @see #setRevision(String)
* @generated
*/
boolean isSetRevision();
/**
* Returns the value of the 'Document Owner' reference.
*
*
* If the meaning of the 'Document Owner' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Document Owner' reference.
* @see #isSetDocumentOwner()
* @see #unsetDocumentOwner()
* @see #setDocumentOwner(IfcActorSelect)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_DocumentOwner()
* @model unsettable="true"
* @generated
*/
IfcActorSelect getDocumentOwner();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentOwner Document Owner}' reference.
*
*
* @param value the new value of the 'Document Owner' reference.
* @see #isSetDocumentOwner()
* @see #unsetDocumentOwner()
* @see #getDocumentOwner()
* @generated
*/
void setDocumentOwner(IfcActorSelect value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentOwner Document Owner}' reference.
*
*
* @see #isSetDocumentOwner()
* @see #getDocumentOwner()
* @see #setDocumentOwner(IfcActorSelect)
* @generated
*/
void unsetDocumentOwner();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentOwner Document Owner}' reference is set.
*
*
* @return whether the value of the 'Document Owner' reference is set.
* @see #unsetDocumentOwner()
* @see #getDocumentOwner()
* @see #setDocumentOwner(IfcActorSelect)
* @generated
*/
boolean isSetDocumentOwner();
/**
* Returns the value of the 'Editors' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcActorSelect}.
*
*
* If the meaning of the 'Editors' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Editors' reference list.
* @see #isSetEditors()
* @see #unsetEditors()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Editors()
* @model unsettable="true"
* @generated
*/
EList getEditors();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getEditors Editors}' reference list.
*
*
* @see #isSetEditors()
* @see #getEditors()
* @generated
*/
void unsetEditors();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getEditors Editors}' reference list is set.
*
*
* @return whether the value of the 'Editors' reference list is set.
* @see #unsetEditors()
* @see #getEditors()
* @generated
*/
boolean isSetEditors();
/**
* Returns the value of the 'Creation Time' attribute.
*
*
* If the meaning of the 'Creation Time' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Creation Time' attribute.
* @see #isSetCreationTime()
* @see #unsetCreationTime()
* @see #setCreationTime(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_CreationTime()
* @model unsettable="true"
* @generated
*/
String getCreationTime();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getCreationTime Creation Time}' attribute.
*
*
* @param value the new value of the 'Creation Time' attribute.
* @see #isSetCreationTime()
* @see #unsetCreationTime()
* @see #getCreationTime()
* @generated
*/
void setCreationTime(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getCreationTime Creation Time}' attribute.
*
*
* @see #isSetCreationTime()
* @see #getCreationTime()
* @see #setCreationTime(String)
* @generated
*/
void unsetCreationTime();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getCreationTime Creation Time}' attribute is set.
*
*
* @return whether the value of the 'Creation Time' attribute is set.
* @see #unsetCreationTime()
* @see #getCreationTime()
* @see #setCreationTime(String)
* @generated
*/
boolean isSetCreationTime();
/**
* Returns the value of the 'Last Revision Time' attribute.
*
*
* If the meaning of the 'Last Revision Time' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Last Revision Time' attribute.
* @see #isSetLastRevisionTime()
* @see #unsetLastRevisionTime()
* @see #setLastRevisionTime(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_LastRevisionTime()
* @model unsettable="true"
* @generated
*/
String getLastRevisionTime();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getLastRevisionTime Last Revision Time}' attribute.
*
*
* @param value the new value of the 'Last Revision Time' attribute.
* @see #isSetLastRevisionTime()
* @see #unsetLastRevisionTime()
* @see #getLastRevisionTime()
* @generated
*/
void setLastRevisionTime(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getLastRevisionTime Last Revision Time}' attribute.
*
*
* @see #isSetLastRevisionTime()
* @see #getLastRevisionTime()
* @see #setLastRevisionTime(String)
* @generated
*/
void unsetLastRevisionTime();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getLastRevisionTime Last Revision Time}' attribute is set.
*
*
* @return whether the value of the 'Last Revision Time' attribute is set.
* @see #unsetLastRevisionTime()
* @see #getLastRevisionTime()
* @see #setLastRevisionTime(String)
* @generated
*/
boolean isSetLastRevisionTime();
/**
* Returns the value of the 'Electronic Format' attribute.
*
*
* If the meaning of the 'Electronic Format' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Electronic Format' attribute.
* @see #isSetElectronicFormat()
* @see #unsetElectronicFormat()
* @see #setElectronicFormat(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_ElectronicFormat()
* @model unsettable="true"
* @generated
*/
String getElectronicFormat();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getElectronicFormat Electronic Format}' attribute.
*
*
* @param value the new value of the 'Electronic Format' attribute.
* @see #isSetElectronicFormat()
* @see #unsetElectronicFormat()
* @see #getElectronicFormat()
* @generated
*/
void setElectronicFormat(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getElectronicFormat Electronic Format}' attribute.
*
*
* @see #isSetElectronicFormat()
* @see #getElectronicFormat()
* @see #setElectronicFormat(String)
* @generated
*/
void unsetElectronicFormat();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getElectronicFormat Electronic Format}' attribute is set.
*
*
* @return whether the value of the 'Electronic Format' attribute is set.
* @see #unsetElectronicFormat()
* @see #getElectronicFormat()
* @see #setElectronicFormat(String)
* @generated
*/
boolean isSetElectronicFormat();
/**
* Returns the value of the 'Valid From' attribute.
*
*
* If the meaning of the 'Valid From' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Valid From' attribute.
* @see #isSetValidFrom()
* @see #unsetValidFrom()
* @see #setValidFrom(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_ValidFrom()
* @model unsettable="true"
* @generated
*/
String getValidFrom();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidFrom Valid From}' attribute.
*
*
* @param value the new value of the 'Valid From' attribute.
* @see #isSetValidFrom()
* @see #unsetValidFrom()
* @see #getValidFrom()
* @generated
*/
void setValidFrom(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidFrom Valid From}' attribute.
*
*
* @see #isSetValidFrom()
* @see #getValidFrom()
* @see #setValidFrom(String)
* @generated
*/
void unsetValidFrom();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidFrom Valid From}' attribute is set.
*
*
* @return whether the value of the 'Valid From' attribute is set.
* @see #unsetValidFrom()
* @see #getValidFrom()
* @see #setValidFrom(String)
* @generated
*/
boolean isSetValidFrom();
/**
* Returns the value of the 'Valid Until' attribute.
*
*
* If the meaning of the 'Valid Until' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Valid Until' attribute.
* @see #isSetValidUntil()
* @see #unsetValidUntil()
* @see #setValidUntil(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_ValidUntil()
* @model unsettable="true"
* @generated
*/
String getValidUntil();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidUntil Valid Until}' attribute.
*
*
* @param value the new value of the 'Valid Until' attribute.
* @see #isSetValidUntil()
* @see #unsetValidUntil()
* @see #getValidUntil()
* @generated
*/
void setValidUntil(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidUntil Valid Until}' attribute.
*
*
* @see #isSetValidUntil()
* @see #getValidUntil()
* @see #setValidUntil(String)
* @generated
*/
void unsetValidUntil();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getValidUntil Valid Until}' attribute is set.
*
*
* @return whether the value of the 'Valid Until' attribute is set.
* @see #unsetValidUntil()
* @see #getValidUntil()
* @see #setValidUntil(String)
* @generated
*/
boolean isSetValidUntil();
/**
* Returns the value of the 'Confidentiality' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc4.IfcDocumentConfidentialityEnum}.
*
*
* If the meaning of the 'Confidentiality' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Confidentiality' attribute.
* @see org.bimserver.models.ifc4.IfcDocumentConfidentialityEnum
* @see #isSetConfidentiality()
* @see #unsetConfidentiality()
* @see #setConfidentiality(IfcDocumentConfidentialityEnum)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Confidentiality()
* @model unsettable="true"
* @generated
*/
IfcDocumentConfidentialityEnum getConfidentiality();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getConfidentiality Confidentiality}' attribute.
*
*
* @param value the new value of the 'Confidentiality' attribute.
* @see org.bimserver.models.ifc4.IfcDocumentConfidentialityEnum
* @see #isSetConfidentiality()
* @see #unsetConfidentiality()
* @see #getConfidentiality()
* @generated
*/
void setConfidentiality(IfcDocumentConfidentialityEnum value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getConfidentiality Confidentiality}' attribute.
*
*
* @see #isSetConfidentiality()
* @see #getConfidentiality()
* @see #setConfidentiality(IfcDocumentConfidentialityEnum)
* @generated
*/
void unsetConfidentiality();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getConfidentiality Confidentiality}' attribute is set.
*
*
* @return whether the value of the 'Confidentiality' attribute is set.
* @see #unsetConfidentiality()
* @see #getConfidentiality()
* @see #setConfidentiality(IfcDocumentConfidentialityEnum)
* @generated
*/
boolean isSetConfidentiality();
/**
* Returns the value of the 'Status' attribute.
* The literals are from the enumeration {@link org.bimserver.models.ifc4.IfcDocumentStatusEnum}.
*
*
* If the meaning of the 'Status' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Status' attribute.
* @see org.bimserver.models.ifc4.IfcDocumentStatusEnum
* @see #isSetStatus()
* @see #unsetStatus()
* @see #setStatus(IfcDocumentStatusEnum)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_Status()
* @model unsettable="true"
* @generated
*/
IfcDocumentStatusEnum getStatus();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getStatus Status}' attribute.
*
*
* @param value the new value of the 'Status' attribute.
* @see org.bimserver.models.ifc4.IfcDocumentStatusEnum
* @see #isSetStatus()
* @see #unsetStatus()
* @see #getStatus()
* @generated
*/
void setStatus(IfcDocumentStatusEnum value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getStatus Status}' attribute.
*
*
* @see #isSetStatus()
* @see #getStatus()
* @see #setStatus(IfcDocumentStatusEnum)
* @generated
*/
void unsetStatus();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getStatus Status}' attribute is set.
*
*
* @return whether the value of the 'Status' attribute is set.
* @see #unsetStatus()
* @see #getStatus()
* @see #setStatus(IfcDocumentStatusEnum)
* @generated
*/
boolean isSetStatus();
/**
* Returns the value of the 'Document Info For Objects' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcRelAssociatesDocument}.
*
*
* If the meaning of the 'Document Info For Objects' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Document Info For Objects' reference list.
* @see #isSetDocumentInfoForObjects()
* @see #unsetDocumentInfoForObjects()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_DocumentInfoForObjects()
* @model unsettable="true"
* @generated
*/
EList getDocumentInfoForObjects();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentInfoForObjects Document Info For Objects}' reference list.
*
*
* @see #isSetDocumentInfoForObjects()
* @see #getDocumentInfoForObjects()
* @generated
*/
void unsetDocumentInfoForObjects();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getDocumentInfoForObjects Document Info For Objects}' reference list is set.
*
*
* @return whether the value of the 'Document Info For Objects' reference list is set.
* @see #unsetDocumentInfoForObjects()
* @see #getDocumentInfoForObjects()
* @generated
*/
boolean isSetDocumentInfoForObjects();
/**
* Returns the value of the 'Has Document References' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcDocumentReference}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcDocumentReference#getReferencedDocument Referenced Document}'.
*
*
* If the meaning of the 'Has Document References' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Document References' reference list.
* @see #isSetHasDocumentReferences()
* @see #unsetHasDocumentReferences()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_HasDocumentReferences()
* @see org.bimserver.models.ifc4.IfcDocumentReference#getReferencedDocument
* @model opposite="ReferencedDocument" unsettable="true"
* @generated
*/
EList getHasDocumentReferences();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getHasDocumentReferences Has Document References}' reference list.
*
*
* @see #isSetHasDocumentReferences()
* @see #getHasDocumentReferences()
* @generated
*/
void unsetHasDocumentReferences();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getHasDocumentReferences Has Document References}' reference list is set.
*
*
* @return whether the value of the 'Has Document References' reference list is set.
* @see #unsetHasDocumentReferences()
* @see #getHasDocumentReferences()
* @generated
*/
boolean isSetHasDocumentReferences();
/**
* Returns the value of the 'Is Pointed To' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcDocumentInformationRelationship}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcDocumentInformationRelationship#getRelatedDocuments Related Documents}'.
*
*
* If the meaning of the 'Is Pointed To' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Pointed To' reference list.
* @see #isSetIsPointedTo()
* @see #unsetIsPointedTo()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_IsPointedTo()
* @see org.bimserver.models.ifc4.IfcDocumentInformationRelationship#getRelatedDocuments
* @model opposite="RelatedDocuments" unsettable="true"
* @generated
*/
EList getIsPointedTo();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIsPointedTo Is Pointed To}' reference list.
*
*
* @see #isSetIsPointedTo()
* @see #getIsPointedTo()
* @generated
*/
void unsetIsPointedTo();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIsPointedTo Is Pointed To}' reference list is set.
*
*
* @return whether the value of the 'Is Pointed To' reference list is set.
* @see #unsetIsPointedTo()
* @see #getIsPointedTo()
* @generated
*/
boolean isSetIsPointedTo();
/**
* Returns the value of the 'Is Pointer' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcDocumentInformationRelationship}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcDocumentInformationRelationship#getRelatingDocument Relating Document}'.
*
*
* If the meaning of the 'Is Pointer' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Pointer' reference list.
* @see #isSetIsPointer()
* @see #unsetIsPointer()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcDocumentInformation_IsPointer()
* @see org.bimserver.models.ifc4.IfcDocumentInformationRelationship#getRelatingDocument
* @model opposite="RelatingDocument" unsettable="true" upper="2"
* @generated
*/
EList getIsPointer();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIsPointer Is Pointer}' reference list.
*
*
* @see #isSetIsPointer()
* @see #getIsPointer()
* @generated
*/
void unsetIsPointer();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcDocumentInformation#getIsPointer Is Pointer}' reference list is set.
*
*
* @return whether the value of the 'Is Pointer' reference list is set.
* @see #unsetIsPointer()
* @see #getIsPointer()
* @generated
*/
boolean isSetIsPointer();
} // IfcDocumentInformation