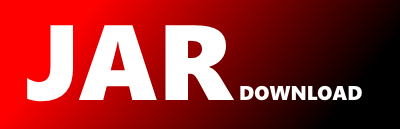
org.bimserver.models.ifc4.IfcObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Object'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc4.IfcObject#getObjectType Object Type}
* - {@link org.bimserver.models.ifc4.IfcObject#getIsDeclaredBy Is Declared By}
* - {@link org.bimserver.models.ifc4.IfcObject#getDeclares Declares}
* - {@link org.bimserver.models.ifc4.IfcObject#getIsTypedBy Is Typed By}
* - {@link org.bimserver.models.ifc4.IfcObject#getIsDefinedBy Is Defined By}
*
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcObject()
* @model
* @generated
*/
public interface IfcObject extends IfcObjectDefinition {
/**
* Returns the value of the 'Object Type' attribute.
*
*
* If the meaning of the 'Object Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Object Type' attribute.
* @see #isSetObjectType()
* @see #unsetObjectType()
* @see #setObjectType(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcObject_ObjectType()
* @model unsettable="true"
* @generated
*/
String getObjectType();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcObject#getObjectType Object Type}' attribute.
*
*
* @param value the new value of the 'Object Type' attribute.
* @see #isSetObjectType()
* @see #unsetObjectType()
* @see #getObjectType()
* @generated
*/
void setObjectType(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcObject#getObjectType Object Type}' attribute.
*
*
* @see #isSetObjectType()
* @see #getObjectType()
* @see #setObjectType(String)
* @generated
*/
void unsetObjectType();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcObject#getObjectType Object Type}' attribute is set.
*
*
* @return whether the value of the 'Object Type' attribute is set.
* @see #unsetObjectType()
* @see #getObjectType()
* @see #setObjectType(String)
* @generated
*/
boolean isSetObjectType();
/**
* Returns the value of the 'Is Declared By' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcRelDefinesByObject}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcRelDefinesByObject#getRelatedObjects Related Objects}'.
*
*
* If the meaning of the 'Is Declared By' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Declared By' reference list.
* @see #isSetIsDeclaredBy()
* @see #unsetIsDeclaredBy()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcObject_IsDeclaredBy()
* @see org.bimserver.models.ifc4.IfcRelDefinesByObject#getRelatedObjects
* @model opposite="RelatedObjects" unsettable="true" upper="2"
* @generated
*/
EList getIsDeclaredBy();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcObject#getIsDeclaredBy Is Declared By}' reference list.
*
*
* @see #isSetIsDeclaredBy()
* @see #getIsDeclaredBy()
* @generated
*/
void unsetIsDeclaredBy();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcObject#getIsDeclaredBy Is Declared By}' reference list is set.
*
*
* @return whether the value of the 'Is Declared By' reference list is set.
* @see #unsetIsDeclaredBy()
* @see #getIsDeclaredBy()
* @generated
*/
boolean isSetIsDeclaredBy();
/**
* Returns the value of the 'Declares' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcRelDefinesByObject}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcRelDefinesByObject#getRelatingObject Relating Object}'.
*
*
* If the meaning of the 'Declares' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Declares' reference list.
* @see #isSetDeclares()
* @see #unsetDeclares()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcObject_Declares()
* @see org.bimserver.models.ifc4.IfcRelDefinesByObject#getRelatingObject
* @model opposite="RelatingObject" unsettable="true"
* @generated
*/
EList getDeclares();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcObject#getDeclares Declares}' reference list.
*
*
* @see #isSetDeclares()
* @see #getDeclares()
* @generated
*/
void unsetDeclares();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcObject#getDeclares Declares}' reference list is set.
*
*
* @return whether the value of the 'Declares' reference list is set.
* @see #unsetDeclares()
* @see #getDeclares()
* @generated
*/
boolean isSetDeclares();
/**
* Returns the value of the 'Is Typed By' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcRelDefinesByType}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcRelDefinesByType#getRelatedObjects Related Objects}'.
*
*
* If the meaning of the 'Is Typed By' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Typed By' reference list.
* @see #isSetIsTypedBy()
* @see #unsetIsTypedBy()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcObject_IsTypedBy()
* @see org.bimserver.models.ifc4.IfcRelDefinesByType#getRelatedObjects
* @model opposite="RelatedObjects" unsettable="true" upper="2"
* @generated
*/
EList getIsTypedBy();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcObject#getIsTypedBy Is Typed By}' reference list.
*
*
* @see #isSetIsTypedBy()
* @see #getIsTypedBy()
* @generated
*/
void unsetIsTypedBy();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcObject#getIsTypedBy Is Typed By}' reference list is set.
*
*
* @return whether the value of the 'Is Typed By' reference list is set.
* @see #unsetIsTypedBy()
* @see #getIsTypedBy()
* @generated
*/
boolean isSetIsTypedBy();
/**
* Returns the value of the 'Is Defined By' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcRelDefinesByProperties}.
*
*
* If the meaning of the 'Is Defined By' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Is Defined By' reference list.
* @see #isSetIsDefinedBy()
* @see #unsetIsDefinedBy()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcObject_IsDefinedBy()
* @model unsettable="true"
* @generated
*/
EList getIsDefinedBy();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcObject#getIsDefinedBy Is Defined By}' reference list.
*
*
* @see #isSetIsDefinedBy()
* @see #getIsDefinedBy()
* @generated
*/
void unsetIsDefinedBy();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcObject#getIsDefinedBy Is Defined By}' reference list is set.
*
*
* @return whether the value of the 'Is Defined By' reference list is set.
* @see #unsetIsDefinedBy()
* @see #getIsDefinedBy()
* @generated
*/
boolean isSetIsDefinedBy();
} // IfcObject
© 2015 - 2025 Weber Informatics LLC | Privacy Policy