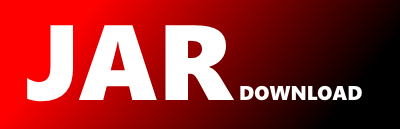
org.bimserver.models.ifc4.IfcProduct Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
import org.bimserver.models.geometry.GeometryInfo;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Product'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc4.IfcProduct#getObjectPlacement Object Placement}
* - {@link org.bimserver.models.ifc4.IfcProduct#getRepresentation Representation}
* - {@link org.bimserver.models.ifc4.IfcProduct#getReferencedBy Referenced By}
* - {@link org.bimserver.models.ifc4.IfcProduct#getGeometry Geometry}
*
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcProduct()
* @model
* @generated
*/
public interface IfcProduct extends IfcObject, IfcProductSelect {
/**
* Returns the value of the 'Object Placement' reference.
* It is bidirectional and its opposite is '{@link org.bimserver.models.ifc4.IfcObjectPlacement#getPlacesObject Places Object}'.
*
*
* If the meaning of the 'Object Placement' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Object Placement' reference.
* @see #isSetObjectPlacement()
* @see #unsetObjectPlacement()
* @see #setObjectPlacement(IfcObjectPlacement)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcProduct_ObjectPlacement()
* @see org.bimserver.models.ifc4.IfcObjectPlacement#getPlacesObject
* @model opposite="PlacesObject" unsettable="true"
* @generated
*/
IfcObjectPlacement getObjectPlacement();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getObjectPlacement Object Placement}' reference.
*
*
* @param value the new value of the 'Object Placement' reference.
* @see #isSetObjectPlacement()
* @see #unsetObjectPlacement()
* @see #getObjectPlacement()
* @generated
*/
void setObjectPlacement(IfcObjectPlacement value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getObjectPlacement Object Placement}' reference.
*
*
* @see #isSetObjectPlacement()
* @see #getObjectPlacement()
* @see #setObjectPlacement(IfcObjectPlacement)
* @generated
*/
void unsetObjectPlacement();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getObjectPlacement Object Placement}' reference is set.
*
*
* @return whether the value of the 'Object Placement' reference is set.
* @see #unsetObjectPlacement()
* @see #getObjectPlacement()
* @see #setObjectPlacement(IfcObjectPlacement)
* @generated
*/
boolean isSetObjectPlacement();
/**
* Returns the value of the 'Representation' reference.
*
*
* If the meaning of the 'Representation' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Representation' reference.
* @see #isSetRepresentation()
* @see #unsetRepresentation()
* @see #setRepresentation(IfcProductRepresentation)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcProduct_Representation()
* @model unsettable="true"
* @generated
*/
IfcProductRepresentation getRepresentation();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getRepresentation Representation}' reference.
*
*
* @param value the new value of the 'Representation' reference.
* @see #isSetRepresentation()
* @see #unsetRepresentation()
* @see #getRepresentation()
* @generated
*/
void setRepresentation(IfcProductRepresentation value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getRepresentation Representation}' reference.
*
*
* @see #isSetRepresentation()
* @see #getRepresentation()
* @see #setRepresentation(IfcProductRepresentation)
* @generated
*/
void unsetRepresentation();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getRepresentation Representation}' reference is set.
*
*
* @return whether the value of the 'Representation' reference is set.
* @see #unsetRepresentation()
* @see #getRepresentation()
* @see #setRepresentation(IfcProductRepresentation)
* @generated
*/
boolean isSetRepresentation();
/**
* Returns the value of the 'Referenced By' reference list.
* The list contents are of type {@link org.bimserver.models.ifc4.IfcRelAssignsToProduct}.
*
*
* If the meaning of the 'Referenced By' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Referenced By' reference list.
* @see #isSetReferencedBy()
* @see #unsetReferencedBy()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcProduct_ReferencedBy()
* @model unsettable="true"
* @generated
*/
EList getReferencedBy();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getReferencedBy Referenced By}' reference list.
*
*
* @see #isSetReferencedBy()
* @see #getReferencedBy()
* @generated
*/
void unsetReferencedBy();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getReferencedBy Referenced By}' reference list is set.
*
*
* @return whether the value of the 'Referenced By' reference list is set.
* @see #unsetReferencedBy()
* @see #getReferencedBy()
* @generated
*/
boolean isSetReferencedBy();
/**
* Returns the value of the 'Geometry' reference.
*
*
* If the meaning of the 'Geometry' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Geometry' reference.
* @see #isSetGeometry()
* @see #unsetGeometry()
* @see #setGeometry(GeometryInfo)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcProduct_Geometry()
* @model unsettable="true"
* @generated
*/
GeometryInfo getGeometry();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getGeometry Geometry}' reference.
*
*
* @param value the new value of the 'Geometry' reference.
* @see #isSetGeometry()
* @see #unsetGeometry()
* @see #getGeometry()
* @generated
*/
void setGeometry(GeometryInfo value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getGeometry Geometry}' reference.
*
*
* @see #isSetGeometry()
* @see #getGeometry()
* @see #setGeometry(GeometryInfo)
* @generated
*/
void unsetGeometry();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcProduct#getGeometry Geometry}' reference is set.
*
*
* @return whether the value of the 'Geometry' reference is set.
* @see #unsetGeometry()
* @see #getGeometry()
* @see #setGeometry(GeometryInfo)
* @generated
*/
boolean isSetGeometry();
} // IfcProduct
© 2015 - 2025 Weber Informatics LLC | Privacy Policy