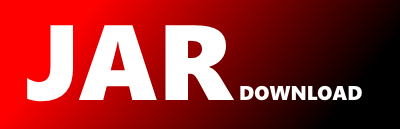
org.bimserver.models.ifc4.IfcSite Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Site'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc4.IfcSite#getRefLatitude Ref Latitude}
* - {@link org.bimserver.models.ifc4.IfcSite#getRefLongitude Ref Longitude}
* - {@link org.bimserver.models.ifc4.IfcSite#getRefElevation Ref Elevation}
* - {@link org.bimserver.models.ifc4.IfcSite#getRefElevationAsString Ref Elevation As String}
* - {@link org.bimserver.models.ifc4.IfcSite#getLandTitleNumber Land Title Number}
* - {@link org.bimserver.models.ifc4.IfcSite#getSiteAddress Site Address}
*
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite()
* @model
* @generated
*/
public interface IfcSite extends IfcSpatialStructureElement {
/**
* Returns the value of the 'Ref Latitude' attribute list.
* The list contents are of type {@link java.lang.Integer}.
*
*
* If the meaning of the 'Ref Latitude' attribute list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Ref Latitude' attribute list.
* @see #isSetRefLatitude()
* @see #unsetRefLatitude()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite_RefLatitude()
* @model unique="false" unsettable="true" upper="3"
* @generated
*/
EList getRefLatitude();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefLatitude Ref Latitude}' attribute list.
*
*
* @see #isSetRefLatitude()
* @see #getRefLatitude()
* @generated
*/
void unsetRefLatitude();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefLatitude Ref Latitude}' attribute list is set.
*
*
* @return whether the value of the 'Ref Latitude' attribute list is set.
* @see #unsetRefLatitude()
* @see #getRefLatitude()
* @generated
*/
boolean isSetRefLatitude();
/**
* Returns the value of the 'Ref Longitude' attribute list.
* The list contents are of type {@link java.lang.Integer}.
*
*
* If the meaning of the 'Ref Longitude' attribute list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Ref Longitude' attribute list.
* @see #isSetRefLongitude()
* @see #unsetRefLongitude()
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite_RefLongitude()
* @model unique="false" unsettable="true" upper="3"
* @generated
*/
EList getRefLongitude();
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefLongitude Ref Longitude}' attribute list.
*
*
* @see #isSetRefLongitude()
* @see #getRefLongitude()
* @generated
*/
void unsetRefLongitude();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefLongitude Ref Longitude}' attribute list is set.
*
*
* @return whether the value of the 'Ref Longitude' attribute list is set.
* @see #unsetRefLongitude()
* @see #getRefLongitude()
* @generated
*/
boolean isSetRefLongitude();
/**
* Returns the value of the 'Ref Elevation' attribute.
*
*
* If the meaning of the 'Ref Elevation' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Ref Elevation' attribute.
* @see #isSetRefElevation()
* @see #unsetRefElevation()
* @see #setRefElevation(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite_RefElevation()
* @model unsettable="true"
* @generated
*/
double getRefElevation();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefElevation Ref Elevation}' attribute.
*
*
* @param value the new value of the 'Ref Elevation' attribute.
* @see #isSetRefElevation()
* @see #unsetRefElevation()
* @see #getRefElevation()
* @generated
*/
void setRefElevation(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefElevation Ref Elevation}' attribute.
*
*
* @see #isSetRefElevation()
* @see #getRefElevation()
* @see #setRefElevation(double)
* @generated
*/
void unsetRefElevation();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefElevation Ref Elevation}' attribute is set.
*
*
* @return whether the value of the 'Ref Elevation' attribute is set.
* @see #unsetRefElevation()
* @see #getRefElevation()
* @see #setRefElevation(double)
* @generated
*/
boolean isSetRefElevation();
/**
* Returns the value of the 'Ref Elevation As String' attribute.
*
*
* If the meaning of the 'Ref Elevation As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Ref Elevation As String' attribute.
* @see #isSetRefElevationAsString()
* @see #unsetRefElevationAsString()
* @see #setRefElevationAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite_RefElevationAsString()
* @model unsettable="true"
* @generated
*/
String getRefElevationAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefElevationAsString Ref Elevation As String}' attribute.
*
*
* @param value the new value of the 'Ref Elevation As String' attribute.
* @see #isSetRefElevationAsString()
* @see #unsetRefElevationAsString()
* @see #getRefElevationAsString()
* @generated
*/
void setRefElevationAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefElevationAsString Ref Elevation As String}' attribute.
*
*
* @see #isSetRefElevationAsString()
* @see #getRefElevationAsString()
* @see #setRefElevationAsString(String)
* @generated
*/
void unsetRefElevationAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcSite#getRefElevationAsString Ref Elevation As String}' attribute is set.
*
*
* @return whether the value of the 'Ref Elevation As String' attribute is set.
* @see #unsetRefElevationAsString()
* @see #getRefElevationAsString()
* @see #setRefElevationAsString(String)
* @generated
*/
boolean isSetRefElevationAsString();
/**
* Returns the value of the 'Land Title Number' attribute.
*
*
* If the meaning of the 'Land Title Number' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Land Title Number' attribute.
* @see #isSetLandTitleNumber()
* @see #unsetLandTitleNumber()
* @see #setLandTitleNumber(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite_LandTitleNumber()
* @model unsettable="true"
* @generated
*/
String getLandTitleNumber();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getLandTitleNumber Land Title Number}' attribute.
*
*
* @param value the new value of the 'Land Title Number' attribute.
* @see #isSetLandTitleNumber()
* @see #unsetLandTitleNumber()
* @see #getLandTitleNumber()
* @generated
*/
void setLandTitleNumber(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getLandTitleNumber Land Title Number}' attribute.
*
*
* @see #isSetLandTitleNumber()
* @see #getLandTitleNumber()
* @see #setLandTitleNumber(String)
* @generated
*/
void unsetLandTitleNumber();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcSite#getLandTitleNumber Land Title Number}' attribute is set.
*
*
* @return whether the value of the 'Land Title Number' attribute is set.
* @see #unsetLandTitleNumber()
* @see #getLandTitleNumber()
* @see #setLandTitleNumber(String)
* @generated
*/
boolean isSetLandTitleNumber();
/**
* Returns the value of the 'Site Address' reference.
*
*
* If the meaning of the 'Site Address' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Site Address' reference.
* @see #isSetSiteAddress()
* @see #unsetSiteAddress()
* @see #setSiteAddress(IfcPostalAddress)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcSite_SiteAddress()
* @model unsettable="true"
* @generated
*/
IfcPostalAddress getSiteAddress();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getSiteAddress Site Address}' reference.
*
*
* @param value the new value of the 'Site Address' reference.
* @see #isSetSiteAddress()
* @see #unsetSiteAddress()
* @see #getSiteAddress()
* @generated
*/
void setSiteAddress(IfcPostalAddress value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcSite#getSiteAddress Site Address}' reference.
*
*
* @see #isSetSiteAddress()
* @see #getSiteAddress()
* @see #setSiteAddress(IfcPostalAddress)
* @generated
*/
void unsetSiteAddress();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcSite#getSiteAddress Site Address}' reference is set.
*
*
* @return whether the value of the 'Site Address' reference is set.
* @see #unsetSiteAddress()
* @see #getSiteAddress()
* @see #setSiteAddress(IfcPostalAddress)
* @generated
*/
boolean isSetSiteAddress();
} // IfcSite