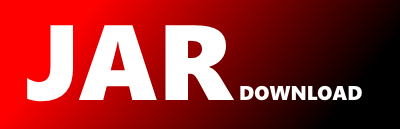
org.bimserver.models.ifc4.IfcTShapeProfileDef Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
/**
*
* A representation of the model object 'Ifc TShape Profile Def'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getDepth Depth}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getDepthAsString Depth As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeWidth Flange Width}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeWidthAsString Flange Width As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebThickness Web Thickness}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebThicknessAsString Web Thickness As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeThickness Flange Thickness}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeThicknessAsString Flange Thickness As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadius Fillet Radius}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadiusAsString Fillet Radius As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadius Flange Edge Radius}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadiusAsString Flange Edge Radius As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadius Web Edge Radius}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadiusAsString Web Edge Radius As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlope Web Slope}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlopeAsString Web Slope As String}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlope Flange Slope}
* - {@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlopeAsString Flange Slope As String}
*
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef()
* @model
* @generated
*/
public interface IfcTShapeProfileDef extends IfcParameterizedProfileDef {
/**
* Returns the value of the 'Depth' attribute.
*
*
* If the meaning of the 'Depth' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Depth' attribute.
* @see #setDepth(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_Depth()
* @model
* @generated
*/
double getDepth();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getDepth Depth}' attribute.
*
*
* @param value the new value of the 'Depth' attribute.
* @see #getDepth()
* @generated
*/
void setDepth(double value);
/**
* Returns the value of the 'Depth As String' attribute.
*
*
* If the meaning of the 'Depth As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Depth As String' attribute.
* @see #setDepthAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_DepthAsString()
* @model
* @generated
*/
String getDepthAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getDepthAsString Depth As String}' attribute.
*
*
* @param value the new value of the 'Depth As String' attribute.
* @see #getDepthAsString()
* @generated
*/
void setDepthAsString(String value);
/**
* Returns the value of the 'Flange Width' attribute.
*
*
* If the meaning of the 'Flange Width' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Width' attribute.
* @see #setFlangeWidth(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeWidth()
* @model
* @generated
*/
double getFlangeWidth();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeWidth Flange Width}' attribute.
*
*
* @param value the new value of the 'Flange Width' attribute.
* @see #getFlangeWidth()
* @generated
*/
void setFlangeWidth(double value);
/**
* Returns the value of the 'Flange Width As String' attribute.
*
*
* If the meaning of the 'Flange Width As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Width As String' attribute.
* @see #setFlangeWidthAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeWidthAsString()
* @model
* @generated
*/
String getFlangeWidthAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeWidthAsString Flange Width As String}' attribute.
*
*
* @param value the new value of the 'Flange Width As String' attribute.
* @see #getFlangeWidthAsString()
* @generated
*/
void setFlangeWidthAsString(String value);
/**
* Returns the value of the 'Web Thickness' attribute.
*
*
* If the meaning of the 'Web Thickness' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Web Thickness' attribute.
* @see #setWebThickness(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_WebThickness()
* @model
* @generated
*/
double getWebThickness();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebThickness Web Thickness}' attribute.
*
*
* @param value the new value of the 'Web Thickness' attribute.
* @see #getWebThickness()
* @generated
*/
void setWebThickness(double value);
/**
* Returns the value of the 'Web Thickness As String' attribute.
*
*
* If the meaning of the 'Web Thickness As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Web Thickness As String' attribute.
* @see #setWebThicknessAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_WebThicknessAsString()
* @model
* @generated
*/
String getWebThicknessAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebThicknessAsString Web Thickness As String}' attribute.
*
*
* @param value the new value of the 'Web Thickness As String' attribute.
* @see #getWebThicknessAsString()
* @generated
*/
void setWebThicknessAsString(String value);
/**
* Returns the value of the 'Flange Thickness' attribute.
*
*
* If the meaning of the 'Flange Thickness' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Thickness' attribute.
* @see #setFlangeThickness(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeThickness()
* @model
* @generated
*/
double getFlangeThickness();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeThickness Flange Thickness}' attribute.
*
*
* @param value the new value of the 'Flange Thickness' attribute.
* @see #getFlangeThickness()
* @generated
*/
void setFlangeThickness(double value);
/**
* Returns the value of the 'Flange Thickness As String' attribute.
*
*
* If the meaning of the 'Flange Thickness As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Thickness As String' attribute.
* @see #setFlangeThicknessAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeThicknessAsString()
* @model
* @generated
*/
String getFlangeThicknessAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeThicknessAsString Flange Thickness As String}' attribute.
*
*
* @param value the new value of the 'Flange Thickness As String' attribute.
* @see #getFlangeThicknessAsString()
* @generated
*/
void setFlangeThicknessAsString(String value);
/**
* Returns the value of the 'Fillet Radius' attribute.
*
*
* If the meaning of the 'Fillet Radius' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Fillet Radius' attribute.
* @see #isSetFilletRadius()
* @see #unsetFilletRadius()
* @see #setFilletRadius(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FilletRadius()
* @model unsettable="true"
* @generated
*/
double getFilletRadius();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadius Fillet Radius}' attribute.
*
*
* @param value the new value of the 'Fillet Radius' attribute.
* @see #isSetFilletRadius()
* @see #unsetFilletRadius()
* @see #getFilletRadius()
* @generated
*/
void setFilletRadius(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadius Fillet Radius}' attribute.
*
*
* @see #isSetFilletRadius()
* @see #getFilletRadius()
* @see #setFilletRadius(double)
* @generated
*/
void unsetFilletRadius();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadius Fillet Radius}' attribute is set.
*
*
* @return whether the value of the 'Fillet Radius' attribute is set.
* @see #unsetFilletRadius()
* @see #getFilletRadius()
* @see #setFilletRadius(double)
* @generated
*/
boolean isSetFilletRadius();
/**
* Returns the value of the 'Fillet Radius As String' attribute.
*
*
* If the meaning of the 'Fillet Radius As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Fillet Radius As String' attribute.
* @see #isSetFilletRadiusAsString()
* @see #unsetFilletRadiusAsString()
* @see #setFilletRadiusAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FilletRadiusAsString()
* @model unsettable="true"
* @generated
*/
String getFilletRadiusAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadiusAsString Fillet Radius As String}' attribute.
*
*
* @param value the new value of the 'Fillet Radius As String' attribute.
* @see #isSetFilletRadiusAsString()
* @see #unsetFilletRadiusAsString()
* @see #getFilletRadiusAsString()
* @generated
*/
void setFilletRadiusAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadiusAsString Fillet Radius As String}' attribute.
*
*
* @see #isSetFilletRadiusAsString()
* @see #getFilletRadiusAsString()
* @see #setFilletRadiusAsString(String)
* @generated
*/
void unsetFilletRadiusAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFilletRadiusAsString Fillet Radius As String}' attribute is set.
*
*
* @return whether the value of the 'Fillet Radius As String' attribute is set.
* @see #unsetFilletRadiusAsString()
* @see #getFilletRadiusAsString()
* @see #setFilletRadiusAsString(String)
* @generated
*/
boolean isSetFilletRadiusAsString();
/**
* Returns the value of the 'Flange Edge Radius' attribute.
*
*
* If the meaning of the 'Flange Edge Radius' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Edge Radius' attribute.
* @see #isSetFlangeEdgeRadius()
* @see #unsetFlangeEdgeRadius()
* @see #setFlangeEdgeRadius(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeEdgeRadius()
* @model unsettable="true"
* @generated
*/
double getFlangeEdgeRadius();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadius Flange Edge Radius}' attribute.
*
*
* @param value the new value of the 'Flange Edge Radius' attribute.
* @see #isSetFlangeEdgeRadius()
* @see #unsetFlangeEdgeRadius()
* @see #getFlangeEdgeRadius()
* @generated
*/
void setFlangeEdgeRadius(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadius Flange Edge Radius}' attribute.
*
*
* @see #isSetFlangeEdgeRadius()
* @see #getFlangeEdgeRadius()
* @see #setFlangeEdgeRadius(double)
* @generated
*/
void unsetFlangeEdgeRadius();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadius Flange Edge Radius}' attribute is set.
*
*
* @return whether the value of the 'Flange Edge Radius' attribute is set.
* @see #unsetFlangeEdgeRadius()
* @see #getFlangeEdgeRadius()
* @see #setFlangeEdgeRadius(double)
* @generated
*/
boolean isSetFlangeEdgeRadius();
/**
* Returns the value of the 'Flange Edge Radius As String' attribute.
*
*
* If the meaning of the 'Flange Edge Radius As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Edge Radius As String' attribute.
* @see #isSetFlangeEdgeRadiusAsString()
* @see #unsetFlangeEdgeRadiusAsString()
* @see #setFlangeEdgeRadiusAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeEdgeRadiusAsString()
* @model unsettable="true"
* @generated
*/
String getFlangeEdgeRadiusAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadiusAsString Flange Edge Radius As String}' attribute.
*
*
* @param value the new value of the 'Flange Edge Radius As String' attribute.
* @see #isSetFlangeEdgeRadiusAsString()
* @see #unsetFlangeEdgeRadiusAsString()
* @see #getFlangeEdgeRadiusAsString()
* @generated
*/
void setFlangeEdgeRadiusAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadiusAsString Flange Edge Radius As String}' attribute.
*
*
* @see #isSetFlangeEdgeRadiusAsString()
* @see #getFlangeEdgeRadiusAsString()
* @see #setFlangeEdgeRadiusAsString(String)
* @generated
*/
void unsetFlangeEdgeRadiusAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeEdgeRadiusAsString Flange Edge Radius As String}' attribute is set.
*
*
* @return whether the value of the 'Flange Edge Radius As String' attribute is set.
* @see #unsetFlangeEdgeRadiusAsString()
* @see #getFlangeEdgeRadiusAsString()
* @see #setFlangeEdgeRadiusAsString(String)
* @generated
*/
boolean isSetFlangeEdgeRadiusAsString();
/**
* Returns the value of the 'Web Edge Radius' attribute.
*
*
* If the meaning of the 'Web Edge Radius' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Web Edge Radius' attribute.
* @see #isSetWebEdgeRadius()
* @see #unsetWebEdgeRadius()
* @see #setWebEdgeRadius(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_WebEdgeRadius()
* @model unsettable="true"
* @generated
*/
double getWebEdgeRadius();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadius Web Edge Radius}' attribute.
*
*
* @param value the new value of the 'Web Edge Radius' attribute.
* @see #isSetWebEdgeRadius()
* @see #unsetWebEdgeRadius()
* @see #getWebEdgeRadius()
* @generated
*/
void setWebEdgeRadius(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadius Web Edge Radius}' attribute.
*
*
* @see #isSetWebEdgeRadius()
* @see #getWebEdgeRadius()
* @see #setWebEdgeRadius(double)
* @generated
*/
void unsetWebEdgeRadius();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadius Web Edge Radius}' attribute is set.
*
*
* @return whether the value of the 'Web Edge Radius' attribute is set.
* @see #unsetWebEdgeRadius()
* @see #getWebEdgeRadius()
* @see #setWebEdgeRadius(double)
* @generated
*/
boolean isSetWebEdgeRadius();
/**
* Returns the value of the 'Web Edge Radius As String' attribute.
*
*
* If the meaning of the 'Web Edge Radius As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Web Edge Radius As String' attribute.
* @see #isSetWebEdgeRadiusAsString()
* @see #unsetWebEdgeRadiusAsString()
* @see #setWebEdgeRadiusAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_WebEdgeRadiusAsString()
* @model unsettable="true"
* @generated
*/
String getWebEdgeRadiusAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadiusAsString Web Edge Radius As String}' attribute.
*
*
* @param value the new value of the 'Web Edge Radius As String' attribute.
* @see #isSetWebEdgeRadiusAsString()
* @see #unsetWebEdgeRadiusAsString()
* @see #getWebEdgeRadiusAsString()
* @generated
*/
void setWebEdgeRadiusAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadiusAsString Web Edge Radius As String}' attribute.
*
*
* @see #isSetWebEdgeRadiusAsString()
* @see #getWebEdgeRadiusAsString()
* @see #setWebEdgeRadiusAsString(String)
* @generated
*/
void unsetWebEdgeRadiusAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebEdgeRadiusAsString Web Edge Radius As String}' attribute is set.
*
*
* @return whether the value of the 'Web Edge Radius As String' attribute is set.
* @see #unsetWebEdgeRadiusAsString()
* @see #getWebEdgeRadiusAsString()
* @see #setWebEdgeRadiusAsString(String)
* @generated
*/
boolean isSetWebEdgeRadiusAsString();
/**
* Returns the value of the 'Web Slope' attribute.
*
*
* If the meaning of the 'Web Slope' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Web Slope' attribute.
* @see #isSetWebSlope()
* @see #unsetWebSlope()
* @see #setWebSlope(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_WebSlope()
* @model unsettable="true"
* @generated
*/
double getWebSlope();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlope Web Slope}' attribute.
*
*
* @param value the new value of the 'Web Slope' attribute.
* @see #isSetWebSlope()
* @see #unsetWebSlope()
* @see #getWebSlope()
* @generated
*/
void setWebSlope(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlope Web Slope}' attribute.
*
*
* @see #isSetWebSlope()
* @see #getWebSlope()
* @see #setWebSlope(double)
* @generated
*/
void unsetWebSlope();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlope Web Slope}' attribute is set.
*
*
* @return whether the value of the 'Web Slope' attribute is set.
* @see #unsetWebSlope()
* @see #getWebSlope()
* @see #setWebSlope(double)
* @generated
*/
boolean isSetWebSlope();
/**
* Returns the value of the 'Web Slope As String' attribute.
*
*
* If the meaning of the 'Web Slope As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Web Slope As String' attribute.
* @see #isSetWebSlopeAsString()
* @see #unsetWebSlopeAsString()
* @see #setWebSlopeAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_WebSlopeAsString()
* @model unsettable="true"
* @generated
*/
String getWebSlopeAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlopeAsString Web Slope As String}' attribute.
*
*
* @param value the new value of the 'Web Slope As String' attribute.
* @see #isSetWebSlopeAsString()
* @see #unsetWebSlopeAsString()
* @see #getWebSlopeAsString()
* @generated
*/
void setWebSlopeAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlopeAsString Web Slope As String}' attribute.
*
*
* @see #isSetWebSlopeAsString()
* @see #getWebSlopeAsString()
* @see #setWebSlopeAsString(String)
* @generated
*/
void unsetWebSlopeAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getWebSlopeAsString Web Slope As String}' attribute is set.
*
*
* @return whether the value of the 'Web Slope As String' attribute is set.
* @see #unsetWebSlopeAsString()
* @see #getWebSlopeAsString()
* @see #setWebSlopeAsString(String)
* @generated
*/
boolean isSetWebSlopeAsString();
/**
* Returns the value of the 'Flange Slope' attribute.
*
*
* If the meaning of the 'Flange Slope' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Slope' attribute.
* @see #isSetFlangeSlope()
* @see #unsetFlangeSlope()
* @see #setFlangeSlope(double)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeSlope()
* @model unsettable="true"
* @generated
*/
double getFlangeSlope();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlope Flange Slope}' attribute.
*
*
* @param value the new value of the 'Flange Slope' attribute.
* @see #isSetFlangeSlope()
* @see #unsetFlangeSlope()
* @see #getFlangeSlope()
* @generated
*/
void setFlangeSlope(double value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlope Flange Slope}' attribute.
*
*
* @see #isSetFlangeSlope()
* @see #getFlangeSlope()
* @see #setFlangeSlope(double)
* @generated
*/
void unsetFlangeSlope();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlope Flange Slope}' attribute is set.
*
*
* @return whether the value of the 'Flange Slope' attribute is set.
* @see #unsetFlangeSlope()
* @see #getFlangeSlope()
* @see #setFlangeSlope(double)
* @generated
*/
boolean isSetFlangeSlope();
/**
* Returns the value of the 'Flange Slope As String' attribute.
*
*
* If the meaning of the 'Flange Slope As String' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Flange Slope As String' attribute.
* @see #isSetFlangeSlopeAsString()
* @see #unsetFlangeSlopeAsString()
* @see #setFlangeSlopeAsString(String)
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcTShapeProfileDef_FlangeSlopeAsString()
* @model unsettable="true"
* @generated
*/
String getFlangeSlopeAsString();
/**
* Sets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlopeAsString Flange Slope As String}' attribute.
*
*
* @param value the new value of the 'Flange Slope As String' attribute.
* @see #isSetFlangeSlopeAsString()
* @see #unsetFlangeSlopeAsString()
* @see #getFlangeSlopeAsString()
* @generated
*/
void setFlangeSlopeAsString(String value);
/**
* Unsets the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlopeAsString Flange Slope As String}' attribute.
*
*
* @see #isSetFlangeSlopeAsString()
* @see #getFlangeSlopeAsString()
* @see #setFlangeSlopeAsString(String)
* @generated
*/
void unsetFlangeSlopeAsString();
/**
* Returns whether the value of the '{@link org.bimserver.models.ifc4.IfcTShapeProfileDef#getFlangeSlopeAsString Flange Slope As String}' attribute is set.
*
*
* @return whether the value of the 'Flange Slope As String' attribute is set.
* @see #unsetFlangeSlopeAsString()
* @see #getFlangeSlopeAsString()
* @see #setFlangeSlopeAsString(String)
* @generated
*/
boolean isSetFlangeSlopeAsString();
} // IfcTShapeProfileDef