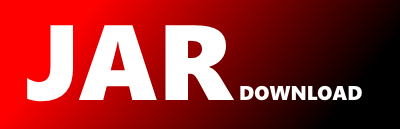
org.bimserver.models.ifc4.IfcWindowStyleConstructionEnum Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.eclipse.emf.common.util.Enumerator;
/**
*
* A representation of the literals of the enumeration 'Ifc Window Style Construction Enum',
* and utility methods for working with them.
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcWindowStyleConstructionEnum()
* @model
* @generated
*/
public enum IfcWindowStyleConstructionEnum implements Enumerator {
/**
* The 'NULL' literal object.
*
*
* @see #NULL_VALUE
* @generated
* @ordered
*/
NULL(0, "NULL", "NULL"),
/**
* The 'ALUMINIUM' literal object.
*
*
* @see #ALUMINIUM_VALUE
* @generated
* @ordered
*/
ALUMINIUM(1, "ALUMINIUM", "ALUMINIUM"),
/**
* The 'HIGH GRADE STEEL' literal object.
*
*
* @see #HIGH_GRADE_STEEL_VALUE
* @generated
* @ordered
*/
HIGH_GRADE_STEEL(2, "HIGH_GRADE_STEEL", "HIGH_GRADE_STEEL"),
/**
* The 'NOTDEFINED' literal object.
*
*
* @see #NOTDEFINED_VALUE
* @generated
* @ordered
*/
NOTDEFINED(3, "NOTDEFINED", "NOTDEFINED"),
/**
* The 'STEEL' literal object.
*
*
* @see #STEEL_VALUE
* @generated
* @ordered
*/
STEEL(4, "STEEL", "STEEL"),
/**
* The 'WOOD' literal object.
*
*
* @see #WOOD_VALUE
* @generated
* @ordered
*/
WOOD(5, "WOOD", "WOOD"),
/**
* The 'ALUMINIUM WOOD' literal object.
*
*
* @see #ALUMINIUM_WOOD_VALUE
* @generated
* @ordered
*/
ALUMINIUM_WOOD(6, "ALUMINIUM_WOOD", "ALUMINIUM_WOOD"),
/**
* The 'OTHER CONSTRUCTION' literal object.
*
*
* @see #OTHER_CONSTRUCTION_VALUE
* @generated
* @ordered
*/
OTHER_CONSTRUCTION(7, "OTHER_CONSTRUCTION", "OTHER_CONSTRUCTION"),
/**
* The 'PLASTIC' literal object.
*
*
* @see #PLASTIC_VALUE
* @generated
* @ordered
*/
PLASTIC(8, "PLASTIC", "PLASTIC");
/**
* The 'NULL' literal value.
*
*
* If the meaning of 'NULL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NULL
* @model
* @generated
* @ordered
*/
public static final int NULL_VALUE = 0;
/**
* The 'ALUMINIUM' literal value.
*
*
* If the meaning of 'ALUMINIUM' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #ALUMINIUM
* @model
* @generated
* @ordered
*/
public static final int ALUMINIUM_VALUE = 1;
/**
* The 'HIGH GRADE STEEL' literal value.
*
*
* If the meaning of 'HIGH GRADE STEEL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #HIGH_GRADE_STEEL
* @model
* @generated
* @ordered
*/
public static final int HIGH_GRADE_STEEL_VALUE = 2;
/**
* The 'NOTDEFINED' literal value.
*
*
* If the meaning of 'NOTDEFINED' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NOTDEFINED
* @model
* @generated
* @ordered
*/
public static final int NOTDEFINED_VALUE = 3;
/**
* The 'STEEL' literal value.
*
*
* If the meaning of 'STEEL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #STEEL
* @model
* @generated
* @ordered
*/
public static final int STEEL_VALUE = 4;
/**
* The 'WOOD' literal value.
*
*
* If the meaning of 'WOOD' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #WOOD
* @model
* @generated
* @ordered
*/
public static final int WOOD_VALUE = 5;
/**
* The 'ALUMINIUM WOOD' literal value.
*
*
* If the meaning of 'ALUMINIUM WOOD' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #ALUMINIUM_WOOD
* @model
* @generated
* @ordered
*/
public static final int ALUMINIUM_WOOD_VALUE = 6;
/**
* The 'OTHER CONSTRUCTION' literal value.
*
*
* If the meaning of 'OTHER CONSTRUCTION' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #OTHER_CONSTRUCTION
* @model
* @generated
* @ordered
*/
public static final int OTHER_CONSTRUCTION_VALUE = 7;
/**
* The 'PLASTIC' literal value.
*
*
* If the meaning of 'PLASTIC' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #PLASTIC
* @model
* @generated
* @ordered
*/
public static final int PLASTIC_VALUE = 8;
/**
* An array of all the 'Ifc Window Style Construction Enum' enumerators.
*
*
* @generated
*/
private static final IfcWindowStyleConstructionEnum[] VALUES_ARRAY = new IfcWindowStyleConstructionEnum[] { NULL,
ALUMINIUM, HIGH_GRADE_STEEL, NOTDEFINED, STEEL, WOOD, ALUMINIUM_WOOD, OTHER_CONSTRUCTION, PLASTIC, };
/**
* A public read-only list of all the 'Ifc Window Style Construction Enum' enumerators.
*
*
* @generated
*/
public static final List VALUES = Collections
.unmodifiableList(Arrays.asList(VALUES_ARRAY));
/**
* Returns the 'Ifc Window Style Construction Enum' literal with the specified literal value.
*
*
* @param literal the literal.
* @return the matching enumerator or null
.
* @generated
*/
public static IfcWindowStyleConstructionEnum get(String literal) {
for (int i = 0; i < VALUES_ARRAY.length; ++i) {
IfcWindowStyleConstructionEnum result = VALUES_ARRAY[i];
if (result.toString().equals(literal)) {
return result;
}
}
return null;
}
/**
* Returns the 'Ifc Window Style Construction Enum' literal with the specified name.
*
*
* @param name the name.
* @return the matching enumerator or null
.
* @generated
*/
public static IfcWindowStyleConstructionEnum getByName(String name) {
for (int i = 0; i < VALUES_ARRAY.length; ++i) {
IfcWindowStyleConstructionEnum result = VALUES_ARRAY[i];
if (result.getName().equals(name)) {
return result;
}
}
return null;
}
/**
* Returns the 'Ifc Window Style Construction Enum' literal with the specified integer value.
*
*
* @param value the integer value.
* @return the matching enumerator or null
.
* @generated
*/
public static IfcWindowStyleConstructionEnum get(int value) {
switch (value) {
case NULL_VALUE:
return NULL;
case ALUMINIUM_VALUE:
return ALUMINIUM;
case HIGH_GRADE_STEEL_VALUE:
return HIGH_GRADE_STEEL;
case NOTDEFINED_VALUE:
return NOTDEFINED;
case STEEL_VALUE:
return STEEL;
case WOOD_VALUE:
return WOOD;
case ALUMINIUM_WOOD_VALUE:
return ALUMINIUM_WOOD;
case OTHER_CONSTRUCTION_VALUE:
return OTHER_CONSTRUCTION;
case PLASTIC_VALUE:
return PLASTIC;
}
return null;
}
/**
*
*
* @generated
*/
private final int value;
/**
*
*
* @generated
*/
private final String name;
/**
*
*
* @generated
*/
private final String literal;
/**
* Only this class can construct instances.
*
*
* @generated
*/
private IfcWindowStyleConstructionEnum(int value, String name, String literal) {
this.value = value;
this.name = name;
this.literal = literal;
}
/**
*
*
* @generated
*/
public int getValue() {
return value;
}
/**
*
*
* @generated
*/
public String getName() {
return name;
}
/**
*
*
* @generated
*/
public String getLiteral() {
return literal;
}
/**
* Returns the literal value of the enumerator, which is its string representation.
*
*
* @generated
*/
@Override
public String toString() {
return literal;
}
} //IfcWindowStyleConstructionEnum